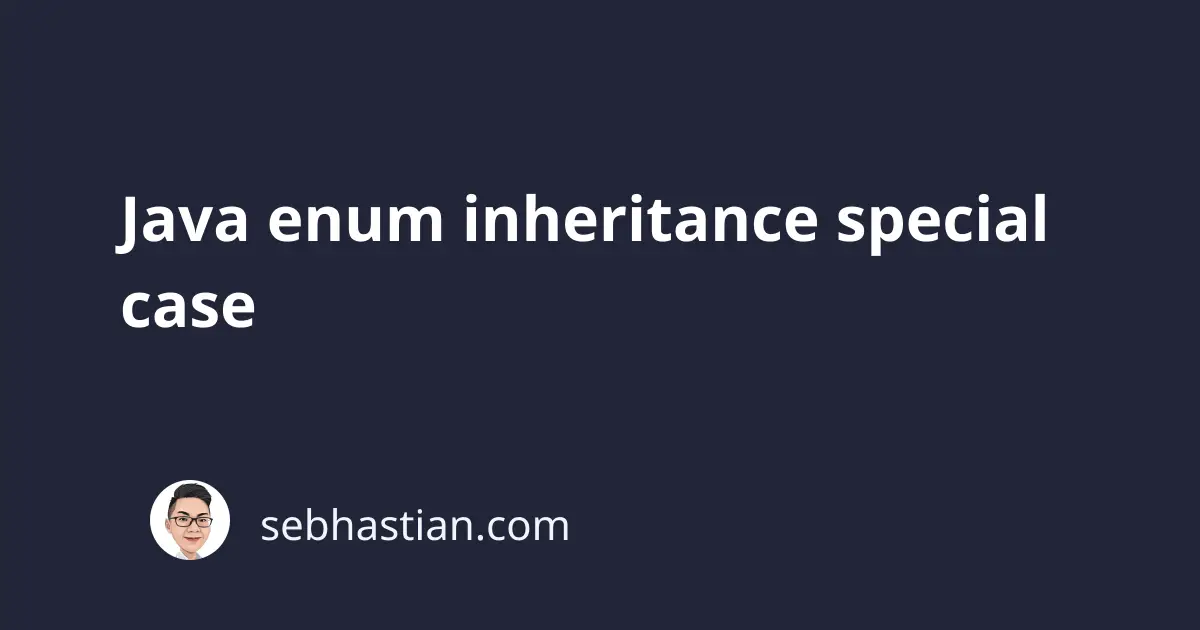
The Java enum
class is a special class that allows you to create a group of constant values (also known as enumerations).
The enum
class is compiled as a final
class in Java, so you can’t extend an enum
class in your code:
enum Colors {
RED, BLUE;
}
enum NewColors extends Colors {}
// ERROR: Cannot inherit from enum 'Colors'
The enum
class itself is also an extension of the abstract class Enum
, so you can’t create a class
that inherits an enum
as shown below:
class Levels {}
enum NewLevels extends Levels {}
// No extends clause allowed for enum
An enum
class represents a complete enumeration of possible values, so you can’t extend an enum
class to add new possible values to it.
What you can do, however, is to create a separate enum
class and use it whenever necessary:
enum Colors {
RED, BLUE;
}
enum NewColors {
GREEN, YELLOW;
}
class Main {
public static void main(String[] args) {
System.out.println(Colors.RED);
System.out.println(NewColors.GREEN);
}
}
But what if your enum
types have some methods defined in the class? The best way to share an enum
class method is to create an interface
that defines those methods.
For example, both Colors
and NewColors
enum can have a shared describeColor()
method as shown below:
interface DescribeColor {
String describeColor();
}
enum Colors implements DescribeColor {
RED, BLUE;
@Override
public String describeColor() {
return "The color is " + this;
}
}
enum NewColors implements DescribeColor {
GREEN, YELLOW;
@Override
public String describeColor() {
return "The color is " + this;
}
}
class Main {
public static void main(String[] args) {
System.out.println(Colors.RED.describeColor());
System.out.println(NewColors.GREEN.describeColor());
}
}
By providing an implementation of the describeColor()
method in each enum
class, you would be able to access the same method from those enums.
The enum
inheritance is intentionally made unavailable in Java because an enumeration should contain a complete list of possible values.
That being said, you can still share methods by implementing one or more interface
types to an enum
class.