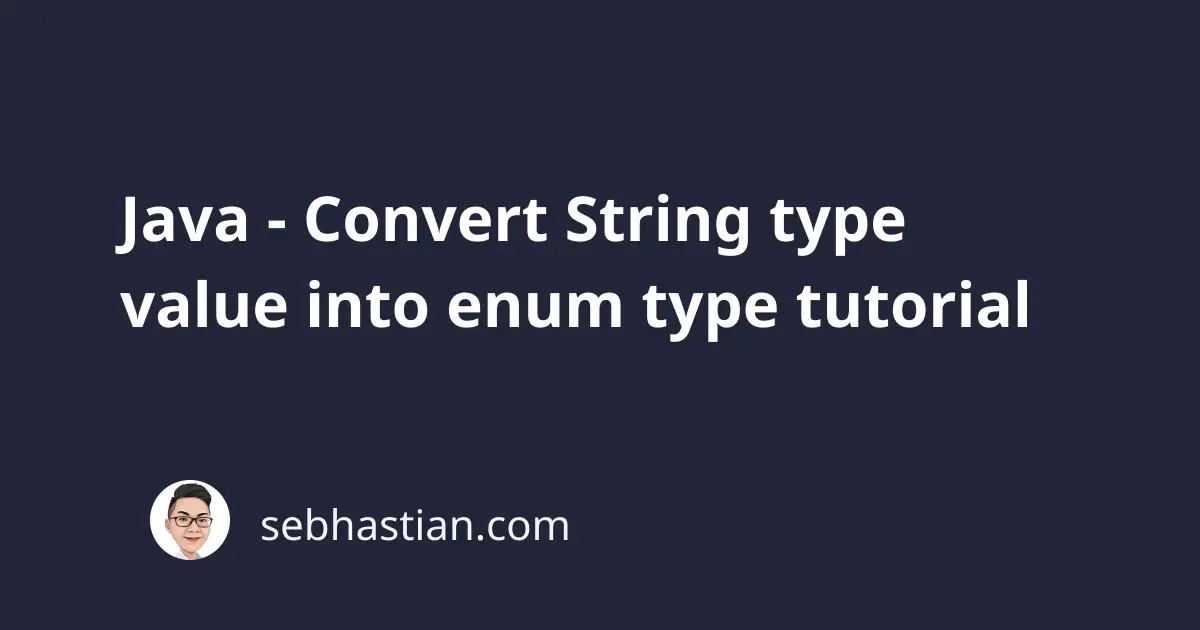
The Java enum
class has a built-in method named valueOf()
that allows you to convert a String
into its equivalent enum
value.
The valueOf()
method of the enum
class is a static method, so you can call it directly from the class without creating an instance.
Let’s see an example of converting a String
into an enum
.
Suppose you have a ColorsEnum
declared in your Java code as follows:
enum ColorsEnum {
RED, GREEN, BLUE;
}
You can convert any String
value that matches one of the three ColorsEnum
values above.
For example, the string "RED"
will be converted to ColorsEnum.RED
like this:
ColorsEnum color = ColorsEnum.valueOf("RED");
System.out.println(color); // RED
System.out.println(color == ColorsEnum.RED); // true
Please note that the valueOf()
method accepts only a String
type value and is case-sensitive.
When the string doesn’t match the case of any enum values, Java will throw the IllegalArgumentException
error:
ColorsEnum color = ColorsEnum.valueOf("red");
// ERROR: No enum constant Main.ColorsEnum.red
ColorsEnum color = ColorsEnum.valueOf("WHITE");
// ERROR: No enum constant Main.ColorsEnum.WHITE
Here’s the complete Java code for converting a String
into an enum
value:
class Main {
// Create the enum class
enum ColorsEnum {
RED, GREEN, BLUE;
}
public static void main(String[] myArgs) {
// Convert the string into an enum
ColorsEnum color = ColorsEnum.valueOf("RED");
System.out.println(color); // RED
System.out.println(color == ColorsEnum.RED); // true
}
}
Now you’ve learned how to convert a String
into an enum
in Java.
Feel free to use the code above in your Java project. 😉