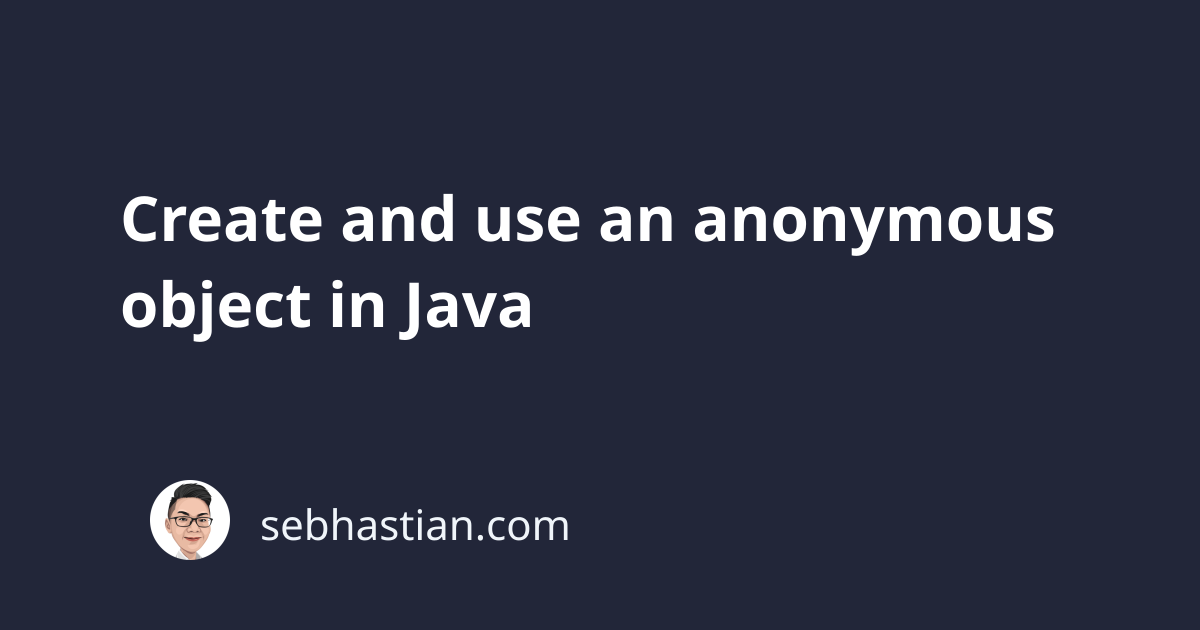
An anonymous object is an object created without any name assigned to that object.
When you create an object in Java, you usually would assign a name to the object.
In the code below, a newly instantiated Person
object is assigned to the reference variable nathan
:
class Person {
void call() {
System.out.println("Hello!");
}
}
Person nathan = new Person();
nathan.call(); // Hello!
The reference variable nathan
points to the Person
object instance, so anytime you want to use or manipulate that object in your code, you call it with the variable name.
On the other hand, an anonymous object is not assigned to a reference variable on instantiation, so you can’t refer to the object after it has been instantiated.
In the following code, the call()
method of a new Person
object is called directly:
new Person().call(); // Hello!
Although it isn’t common, an anonymous object is useful when you need an object no more than once in your program. You can still call on its methods as if they are static methods.
The following shows how you can use anonymous objects to call on their methods:
class Person {
void call(String name) {
System.out.println("Hello, " + name + "!");
}
int sum(int x, int y) {
return x + y;
}
}
new Person().call("Jane"); // Hello, Jane!
int calculation = new Person().sum(7, 20);
System.out.println(calculation); // 27
Since Java 10, you can also create and store an anonymous object during instantiation by saving the instance with the var
keyword.
In the code below, a new Object
is created and referenced from myObj
reference variable:
boolean result = true;
var myObj = new Object() {
void greetings() {
System.out.println("Hello World!");
}
boolean success = result;
};
System.out.println(myObj.success); // true
myObj.greetings(); // Hello World!
The var
keyword in Java 10 will infer the type of the variable based on the surrounding context.
Inside the new Object()
body, you can define variables and methods that the object instance will have.
After that, you can call and use the instance variables and methods just like a normal object.
Now you’ve learned how to create and use anonymous objects in Java. Good work! 👍