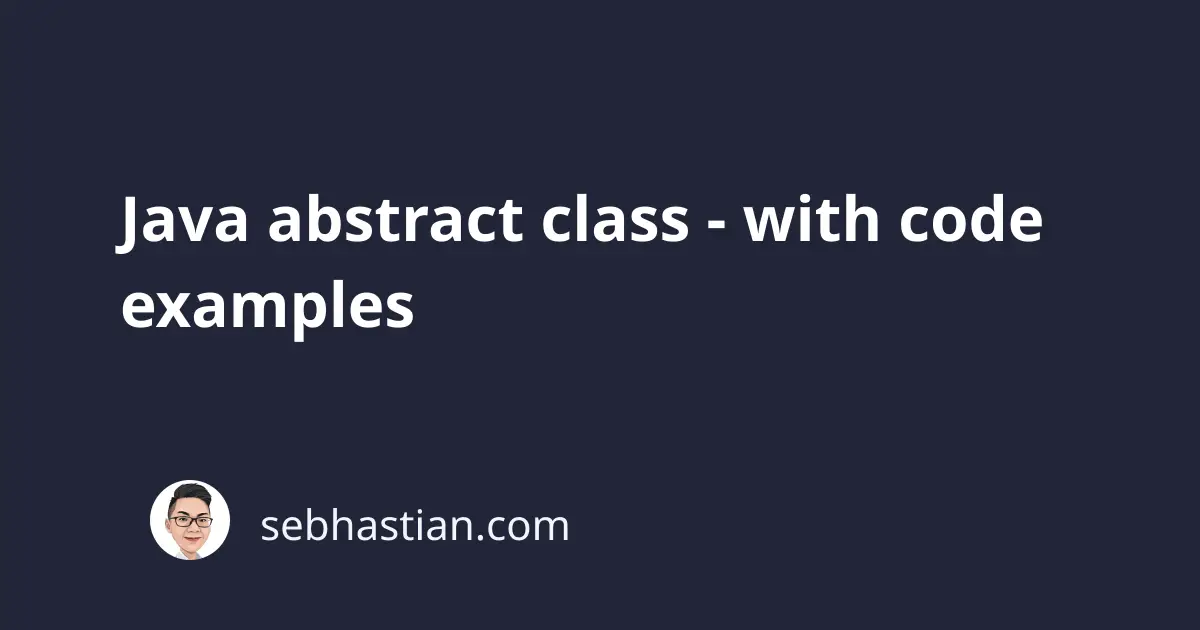
In Java, an abstract class
is a reference type used for adding an abstraction layer to your program.
An abstract class
can’t be used to create objects directly. Rather, you need to extend the class in a regular (concrete) java class to utilize it.
The code example below shows how you can extend an abstract class named Human
to a class named Programmer
:
abstract class Human {
public void greetings() {
System.out.println("Hello World!");
}
}
class Programmer extends Human {}
Programmer nathan = new Programmer();
nathan.greetings(); // Hello World!
When you create an instance of the Programmer
class, you can call the greetings()
method defined in the Human
abstract class.
Trying to create an instance of an abstract class
will cause Java to throw an error as shown below:
Human nathan = new Human();
// Error: 'Human' is abstract;
// cannot be instantiated
An abstract class
can have both abstract and non-abstract methods. A regular class can only have non-abstract methods.
An abstract
method needs to be implemented by the subclass, or Java won’t compile as shown below:
abstract class Human {
public void greetings() {
System.out.println("Hello World!");
}
abstract void work();
}
class Programmer extends Human {}
// Error: Class 'Programmer' must either be declared abstract
// or implement abstract method 'work()' in 'Human'
To make the code above work, you need to implement the work()
method in the Programmer
class:
class Programmer extends Human {
@Override
void work() {
System.out.println("Developing apps with Java!");
}
}
All methods declared as abstract
in the abstract class
must be implemented by the class that inherits it.
In all other regards, an abstract class
is the same as a regular class:
- an
abstract class
can have constructors and static methods - an
abstract class
can have final methods and attributes - an
abstract class
can extend another abstract or regular class
An abstract class
is similar to an interface
, but there are key differences between the two that you need to understand.
Learn more here: Java Interface vs Abstract Class
Now you’ve learned how an abstract class
can be created in Java. Good work! 👍