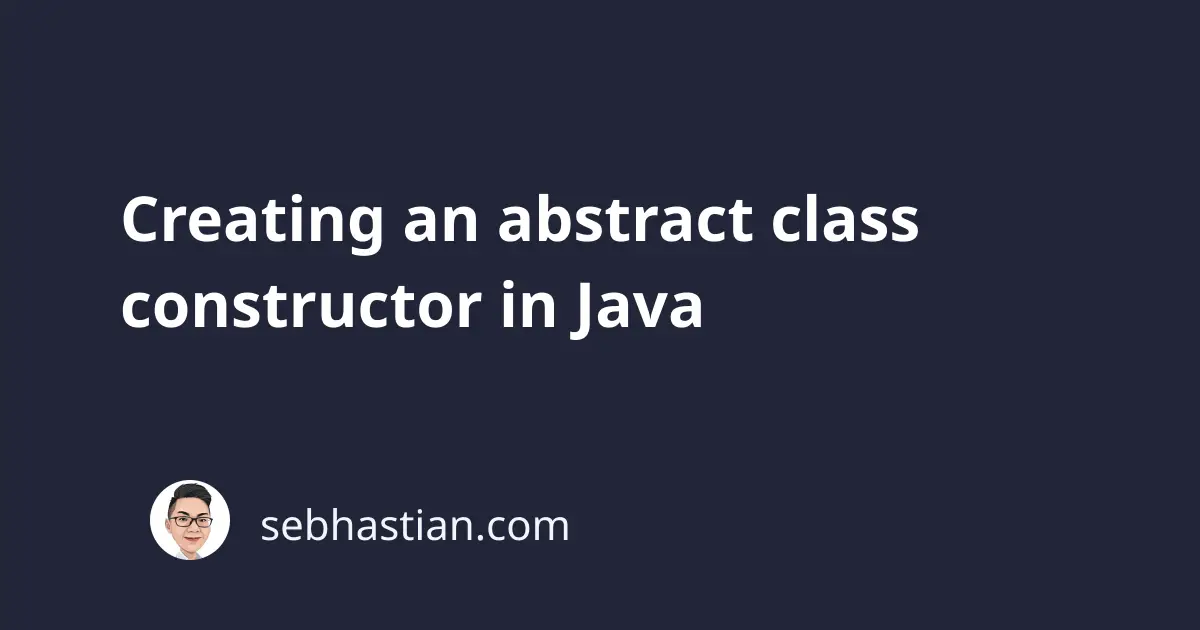
When you define a Java class
construct, the Java compiler automatically creates a constructor with no argument during the execution of the program.
This means that by default, even an abstract class
in Java has a default constructor that does nothing.
An abstract class
can’t be instantiated directly, so you need to call super()
from the subclass constructor to execute the abstract constructor.
For example, suppose you have an abstract class named Dog
as shown below:
abstract class Dog {
final String breed;
public Dog(String breed){
this.breed = breed;
}
}
In the above Dog
abstract class, a constructor is defined to initialize the final
variable breed
.
This constructor can be called from any class
that extends the Dog
class.
Consider the Terrier
class below. It calls the Dog
constructor by calling the super()
method in its constructor:
class Terrier extends Dog {
public Terrier(String breed) {
super(breed);
}
}
By creating an abstract class
that has a constructor, you ensure that its subclasses will have the final
variable initialized.
The variable is initialized when an instance of the subclass is created and then can’t be changed.
There is one condition when you might want to define a constructor in an abstract class, and that is when you want to enforce that all subclasses of the superclass have specific member variables initialized.
The variables don’t have to be final
variables, but if your abstract class has one or more variables, and you want them to be initialized on instantiation, then you need to create the constructor.
Finally, an abstract class constructor behaves just like any other class type.
The only difference is that you can’t instantiate an abstract class, so you need to extend it and call the constructor using super()
.
So yes, you can create an abstract class constructor in Java when you need to. 😉