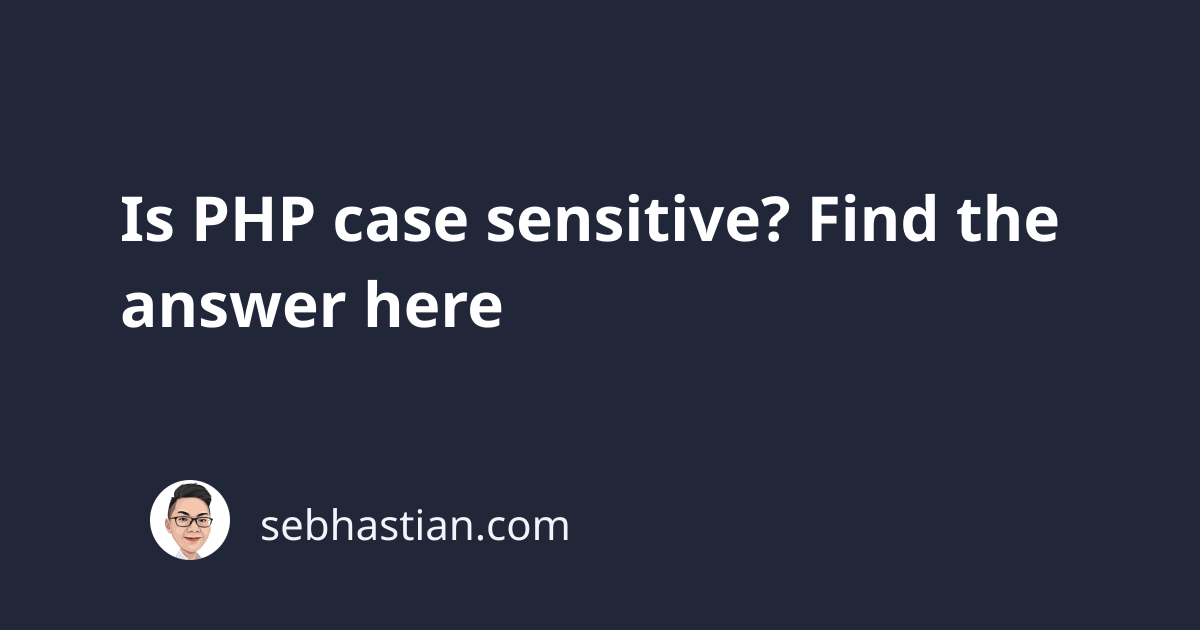
PHP is a unique programming language in terms of case sensitivity.
In PHP, variables and constants are case sensitive, while functions are not case sensitive.
PHP classes are a mix between variables and functions, so they are partially case-sensitive.
Let’s see some practical example below:
// 👇 you can create two variables like this:
$num = 99;
$NUM = 20;
echo $num; // 99
echo "\n".$NUM; // 20
// 👇 but you can't have two functions like this:
function greetings(){
echo "Hello World!";
}
// 👇 Fatal error: Cannot redeclare GREETINGS()
function GREETINGS(){
echo "Hello World!";
}
As you can see in the example above, the variables $num
and $NUM
can have different values.
But when you declare two functions with the same name, PHP produces a fatal error: cannot redeclare the function. This happens even though the function names use different letter cases.
However, PHP will ignore casing when calling a function:
function greetings(){
echo "Hello World!";
}
// 👇 this works
GreeTingS();
PHP system constants like $GLOBALS
, $_SERVER
, $_GET
, $_POST
are variables, so they are also case sensitive:
<?php
$name = "Nathan";
// 👇 the $globals array are different than $GLOBALS
$globals["name"] = "Jack";
echo $GLOBALS["name"]; // Nathan
echo "\n".$globals["name"]; // Jack
The PHP class
is partially sensitive because the class declaration is not case sensitive:
class Human {}
// 👇 Fatal error: Cannot declare class human
class human {}
Class instantiations are not case sensitive as well:
class Human {
function greet(){
echo "Hi!";
}
}
// 👇 this instantiation works
$h = new HUMAN();
$h->greet();
But class properties are case sensitive, so you can create two different properties with the same name, but different cases:
class Human {
public $name = "Nathan";
public $NAME = "Jack";
}
$h = new HUMAN();
echo $h->name; // Nathan
echo "\n".$h->NAME; // Jack
So here’s the summary of PHP case sensitivity:
- Variables are case-sensitive. This includes system variables
- Functions are not case sensitive
- Classes are a mix of variables and functions, so they are partially case sensitive
- Class declaration, instantiation, and methods are not case sensitive
- Class properties are case sensitive
These are the basic rules of case sensitivity in PHP.
If you have studied other programming languages before, you may feel weird about PHP’s case-insensitive quirks in functions and classes. This is different from other languages like JavaScript or Python.
As to why it’s like that, I think it’s a language design mistake when functions are first introduced.
In PHP/FI v2 functions were released as case insensitive1. Changing this behavior may break many applications running on PHP.
Don’t worry too much about case sensitivity though, it won’t cause any issue if you follow the PHP coding standard.
Thanks for reading! I hope this tutorial has been useful for you 🙏
PHP/FI function names are not case sensitive ↩︎