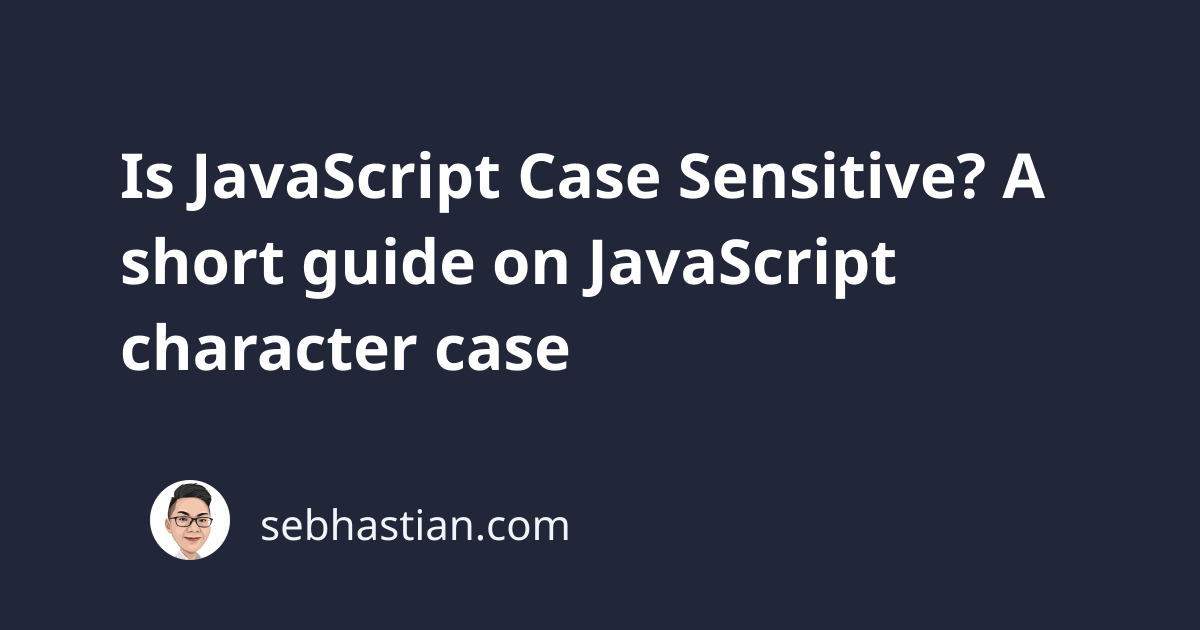
JavaScript is a case-sensitive language, which means you can declare multiple variables using the same word with different cases. For example, the following declarations are considered different variables for JavaScript:
let myvariable = "myvariable";
let MyVariable = "MyVariable";
let MYvariable = "MYvariable";
let MYVARIABLE = "MYVARIABLE";
let myvariablE = "myvariablE";
This also means that you need to use JavaScript keywords with the matching cases too. The let
keyword cannot be written as “LET” or “Let”:
LET myVariable; // SyntaxError: Unexpected identifier
If(true){
// SyntaxError: Unexpected token '{'
console.log("Hello");
}
JavaScript also has naming conventions, which means there are certain expectations for naming variables, functions, and classes that are widely accepted as the norm by JavaScript developers. Most of them are common sense, but here’s a short guide:
Names should be descriptive
Any name that you defined, either it’s a variable, a function, or a class should be descriptive enough that other developers and even you yourself in the future won’t misinterpret the variable as anything else.
For example, when you create a variable that holds the name of a user, use name
as the variable:
let val = "Jack"; // bad
let data = "Jack"; // bad
let name = "Jack"; // good
When your naming contains more than one word, you need to use the camelCase
naming convention, where the first word starts with a lower-case character, and the rest starts with an upper-case character:
let firstName = "Jack"; // right
let FirstName = "Jack"; // wrong
let myFirstName = "Nathan"; // right
JavaScript has no limit to the length of a variable name, but it’s recommended to use three words maximum to name things:
let thisIsFirstName = "Jack"; // too many words than neccessary
let aVeryLongVariableNameThatDescribesName = "Jack"; // really bad idea
The rule of thumb is to use just one very descriptive word to name things. Make it two when you need to, but never more than three.
Naming rule for JavaScript booleans type
boolean
variables are usually named using the prefix “is” or “has” to distinguish it from string
and number
types.
These examples should give you an idea:
let isActive = true;
let isExist = false;
let hasBillingAddress = true;
let isMember = true;
Naming rule for JavaScript constants
Constants are variables with immutable values, meaning that you can’t change the value of these variables after initialization. Constants in JavaScript are declared using the const
keyword followed by the name in capital letters. Yes, you need to use all upper-case words:
const KEYWORD = "Red";
When you have more than one word, you need to separate the name using the underscore (_
) as follows:
const WEBSITE_LANGUAGE = "en-us";
Naming rule for JavaScript functions
JavaScript functions use the same camelCase
naming convention like variables, but you’re also recommended to add a verb describing what the function actually do as a prefix to the name.
For example:
// not bad, but doesn't really describe what the function do
// the function concatenate the names, not getting them from a source
function getNames(firstName, lastName) {
return `${firstName} ${lastName}`;
}
// right on point
function concatNames(firstName, lastName) {
return `${firstName} ${lastName}`;
}
Naming rule for JavaScript classes
JavaScript classes use the PascalCase
naming convention, where the first character of each word use the upper-case character.
class UserProfile {}
class Car {}