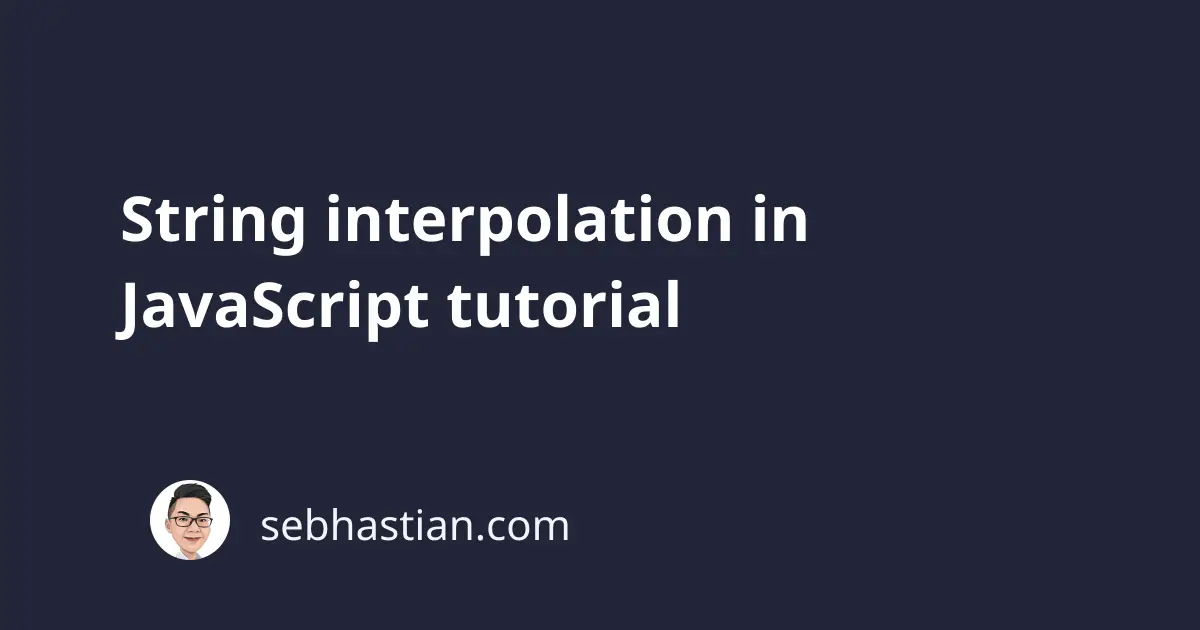
When you need to add JavaScript variable values and expressions to complete a string, your first intuition might be to concatenate the string using unary operators +
as in the following example:
const name = "Nathan";
const age = 29;
console.log("My name is " + name + " and I'm " + age + " years old.");
// "My name is Nathan and I'm 29 years old."
But JavaScript ES6 actually provides you with a cleaner method to add expressions to a string. Instead of using single quotes ''
or double quotes ""
to form the string, you can use the backticks \``\
and the placeholder symbol ${expression}
to form your string.
Forming a string using backticks is known as template literals or template string, and here’s how it works:
const name = "Nathan";
const age = 29;
console.log(`My name is ${name} and I'm ${age} years old.`);
// "My name is Nathan and I'm 29 years old."
A template string works just like ordinary string literals (single quote and double quote mark) with the addition of embedding JavaScript expressions into the string.
In the code example above, the printed string has the variable name
and age
values embedded into it, but you can actually perform some JavaScript expression evaluation as follows:
console.log(`10 - 5 equals ${10 - 5}`); // "10 - 5 equals 5"
You can even use ternary operator to evaluate conditions as follows:
let name;
console.log(`Hello, ${name ? name : "World"}!`);
// "Hello, World!"
name = "John";
console.log(`Hello, ${name ? name : "World"}!`);
// "Hello, John!"
Finally, when you need to write a literal placeholder syntax "${}"
into your template string, you need to escape the placeholder syntax by putting the backslash \
symbol before the syntax as follows:
console.log(`My name is \${John Smith}`);
// "My name is ${John Smith}"
Without the backslash, JavaScript will consider the text "${John Smith}"
as an expression instead of literal values.
String interpolation from template string is one of the most useful features of ES6 JavaScript. Modern web applications tend to be complex and have conditional outputs, So when you need to log an error or certain variable values into the screen, the template string will definitely help you out.