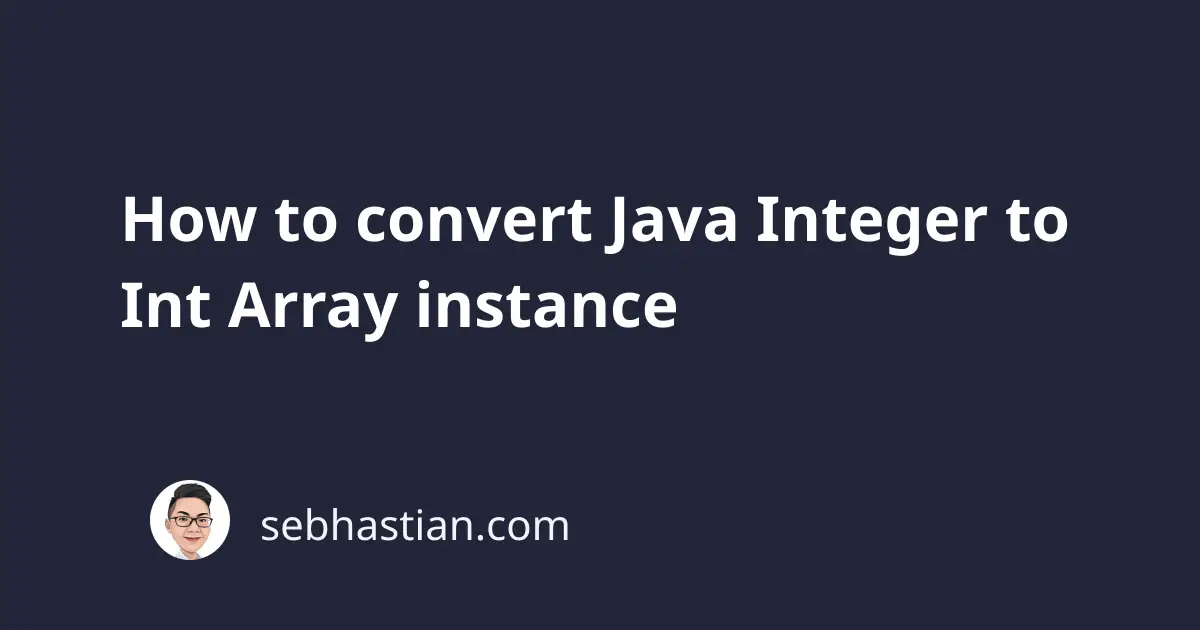
To convert an Integer
or int
type data into an int[]
array type, you need to do the following steps:
- First, convert your
Integer
orint
into aString
- Then create a new
int[]
instance and use the value ofString.length()
method as the size of the array - Use a
for
loop to put eachString
character inside the array
Let’s see an example of converting an int
value into an int[]
.
First, create a String
representation of the int
type using Integer.toString()
method:
public static void main(String[] args)
{
int num = 12345;
String temp = Integer.toString(num);
}
Then, create a new int[]
where you will put the integers later.
Use the String.length()
method to determine the size of the array:
public static void main(String[] args)
{
int num = 12345;
String temp = Integer.toString(num);
int[] numbers = new int[temp.length()];
}
Finally, create a for
loop to iterate through the String
and assign each character inside the String
into the array.
Take a look at the code below:
for (int i = 0; i < temp.length(); i++) {
numbers[i] = Character.getNumericValue(temp.charAt(i));
}
You need to use the combination of Character.getNumericalValue()
and String.charAt()
methods to get the right integer value.
This is because the String.charAt()
method returns the number as a String
, and you will get the Unicode value of the numbers without using the Character.getNumericalValue()
method.
The code below shows the result of calling only the charAt()
method:
for (int i = 0; i < temp.length(); i++) {
numbers[i] = temp.charAt(i);
}
System.out.println("Variable numbers content: " + Arrays.toString(numbers));
// Variable numbers content: [49, 50, 51, 52, 53]
That’s why you need to wrap the value returned by charAt()
method with getNumericalValue()
method.
Below is the full code for converting an int
to an int[]
using Java:
public static void main(String[] args)
{
int num = 12345;
String temp = Integer.toString(num);
int[] numbers = new int[temp.length()];
for (int i = 0; i < temp.length(); i++) {
numbers[i] = Character.getNumericValue(temp.charAt(i));
}
System.out.println("Variable numbers type: "
+ numbers.getClass().getSimpleName());
// Variable numbers type: int[]
System.out.println("Variable numbers content: "
+ Arrays.toString(numbers));
// Variable numbers content: [1, 2, 3, 4, 5]
}
Feel free to modify the code above as you require 😉