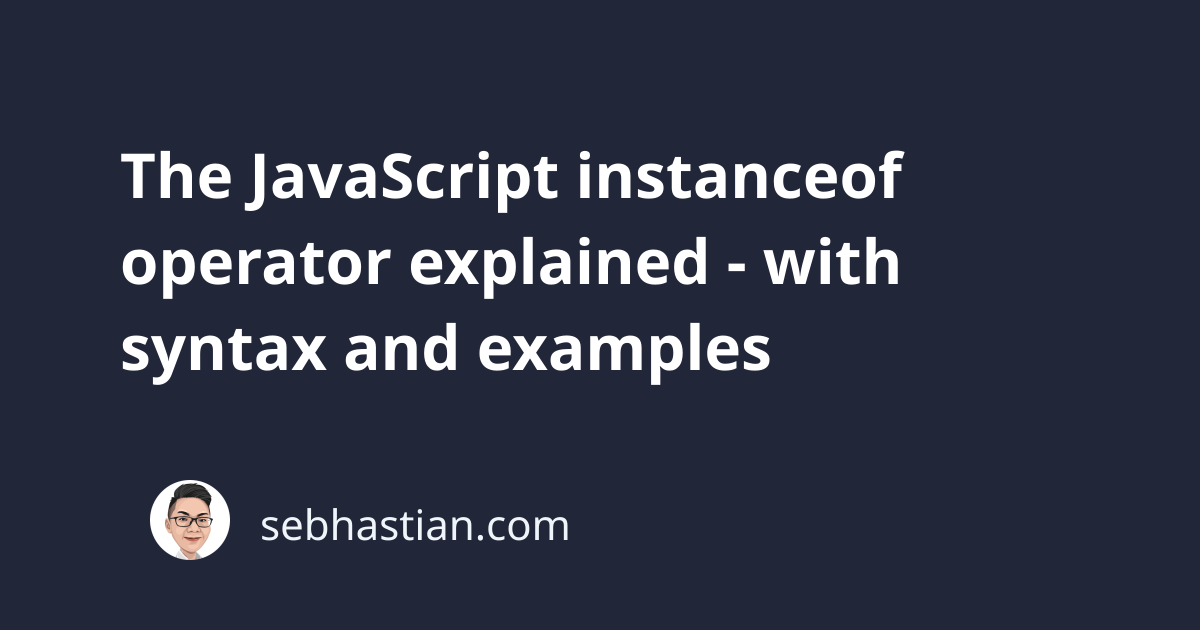
The JavaScript instanceof
operator is used to find out the type of objects or values you have in your code.
The instanceof
syntax is as follows:
object instanceof Object;
// returns a boolean: true or false
The instanceof
will test if the Object
constructor is present in the object
’s prototype chain.
The example code below shows how you can use the instanceof
method to test the Person
object instance:
class Person {}
let person = new Person();
console.log(person instanceof Person); // true
console.log(person instanceof Object); // true
console.log(person instanceof Boolean); // false
Because the person
variable’s value is an instance of both Person
and Object
classes, the instanceof
operator returns true
.
But the person
object is not an instance of the Boolean
class, so the operator returns false
.
The instanceof
operator also works when you create an object from a function
as shown below:
function Dog() {}
let dog = new Dog();
console.log(dog instanceof Dog); // true
As you can see, the dog
variable is an instance of Dog
, so the instanceof
operator returns true
.
JavaScript instanceof vs typeof
JavaScript also has the typeof
operator that can be used to check the type of values you define in your code.
The typeof
operator returns a string
representing the type of the value. It is commonly used for built-in JavaScript types as shown below:
console.log(typeof "Hello"); // "string"
console.log(typeof 123); // "number"
The instanceof
and typeof
operators work in different ways.
The instanceof
returns either true
or false
while the typeof
returns the actual type name of the value.
In general, the instanceof
is used for testing advanced and custom types, while typeof
is used for common JavaScript types.
The code below shows the difference between the two operators:
class Person {}
let person = new Person();
console.log(person instanceof Person); // true
console.log(typeof person); // "object"
console.log("Hello" instanceof String); // false
console.log(typeof "Hello"); // "string"
console.log(typeof "Hello" == "string"); // true
console.log(true instanceof Boolean); // false
console.log(typeof true); // "boolean"
console.log(true instanceof "boolean"); // ERROR
Now you’ve learned how the instanceof
operator works in JavaScript, as well as the difference between the instanceof
and typeof
operators.
I hope this tutorial has been useful for you. 🙏