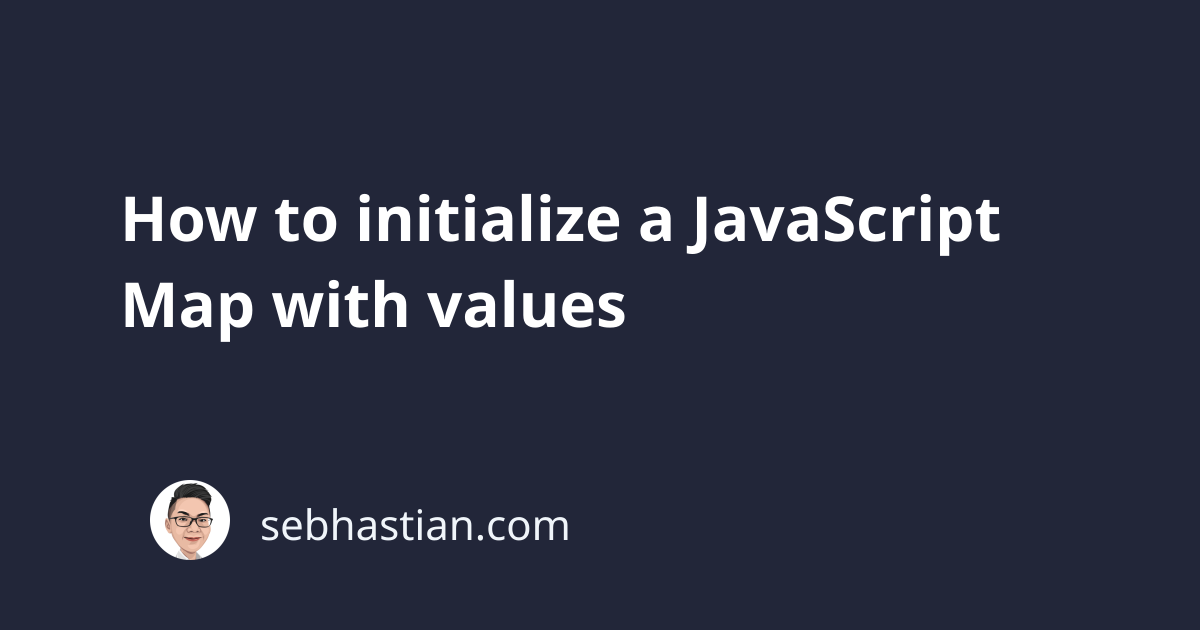
When creating a Map
object in JavaScript, the usual way to insert a key-value pair is by using the set()
method of the object.
Consider the following code example:
const map1 = new Map();
map1.set("x", 10);
map1.set("y", 20);
map1.set("z", 30);
console.log(map1);
// π {'x' => 10, 'y' => 20, 'z' => 30}
As you can see, using the set()
method from the Map
instance is a bit redundant.
You can actually initialize a Map
object with values by passing an array to the Map()
constructor.
The array will contain another array that holds the key-value pair to be inserted into the Map
.
The example code below produces the same result as the above:
const map1 = new Map([
["x", 10],
["y", 20],
["z", 30],
]);
console.log(map1);
// π {'x' => 10, 'y' => 20, 'z' => 30}
Alternatively, you can also initialize a map with values from a JavaScript Object
like this:
const myObj = { name: "Nathan", age: 29 };
const map2 = new Map(Object.entries(myObj));
console.log(map2);
// π {'name' => 'Nathan', 'age' => 29}
This is possible because the Object.entries()
method returns an array of arrays containing the properties of the object.
And thatβs how you can initialize a Map
with values in JavaScript. π