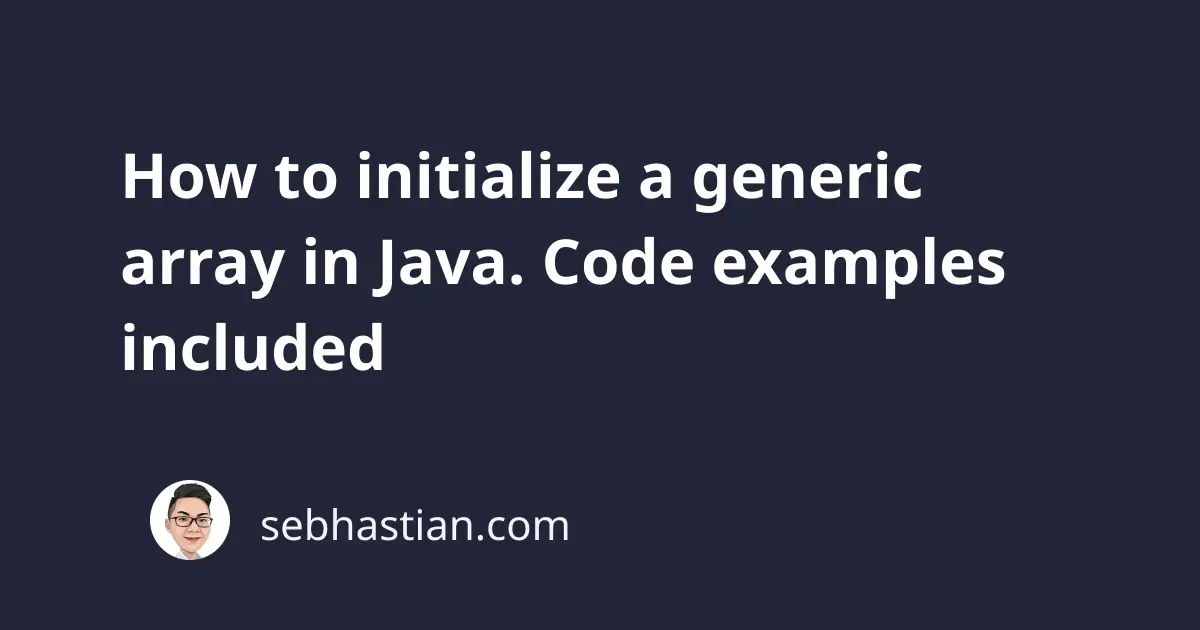
In Java, an array with a specific type can be initialized by adding square brackets notation next to the type.
Here’s an example of initializing a String
array:
String[] names = new String[4];
If you want to create an array of int
values, then you only need to change the String
type:
int[] numbers = new int[4];
Knowing this, it seems that to initialize a generic array, you only need to type a generic like this:
T[] names = new T[4];
But the code above will produce compile error because Java doesn’t know what you mean with the placeholder T
type above.
The build will fail with the cannot find symbol class T
error thrown.
When you need a generic array, you can’t use the Java array notation T[]
or E[]
when initializing it.
Instead, you need to use the ArrayList
class to initialize a generic array:
ArrayList elements = new ArrayList(10);
elements.add("Nathan");
elements.add(22);
elements.add(false);
elements.add(null);
System.out.println(elements);
// [Nathan, 22, false, null]
When you initialize a generic array with the ArrayList
class, you can add values of any type into the array.
Another way to initialize a generic array is to create your own implementation of the Array
class that initializes an Object
type array like this:
class Array<T> {
private final Object[] obj_array;
public final int length;
// Initialize the array in the constructor
public Array(int length) {
// instantiate a new Object array
obj_array = new Object[length];
this.length = length;
}
}
In the above Array
class constructor, a new Object
array is initialized with the length specified as the constructor’s parameter.
After the constructor, you need to define the get()
and set()
methods to set and retrieve the array elements:
T get(int i) {
@SuppressWarnings("unchecked")
final T element = (T)obj_array[i];
return element;
}
void set(int i, T element) {
obj_array[i] = element;
}
Now you can create a new Array
instance as shown below:
// Define the Array class
class Array<T> {
private final Object[] obj_array;
public final int length;
public Array(int length) {
obj_array = new Object[length];
this.length = length;
}
T get(int i) {
@SuppressWarnings("unchecked")
final T element = (T) obj_array[i];
return element;
}
void set(int i, T element) {
obj_array[i] = element;
}
}
// Test the Array class
public class Main {
public static void main(String[] args) {
Array elements = new Array(5);
elements.set(0, "Nathan");
elements.set(1, 22);
elements.set(2, false);
System.out.println(elements.get(0)); // Nathan
System.out.println(elements.get(1)); // 22
}
}
The custom Array
class implementation above is very minimum, and you might face issues in the future for using it.
Unless you are willing to maintain and improve the Array
class as you develop your application, it’s recommended to use the ArrayList
class provided by Java instead.
The ArrayList
has many methods that will help you in manipulating its instance.
And that’s how you can initialize a generic array in Java.
I hope this tutorial has been useful for you. 🙏