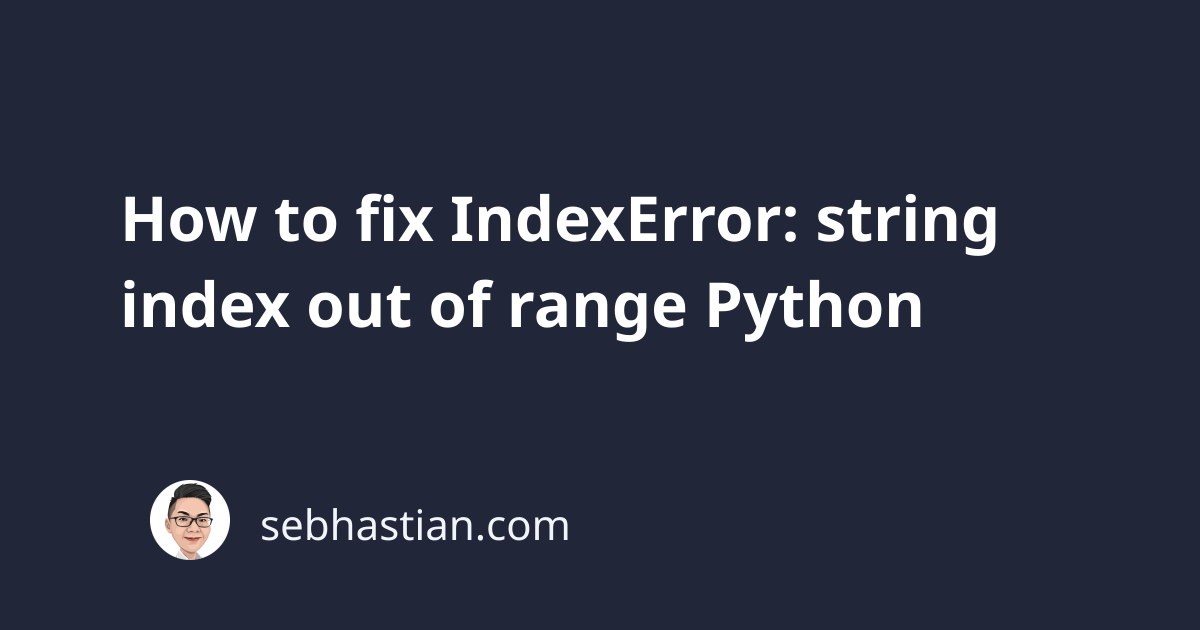
When working with Python strings, you might encounter the following error:
IndexError: string index out of range
This error occurs when you attempt to access a character in a string with an index position that’s out of the available range.
This tutorial will show you an example that causes this error and how to solve it in practice.
How to reproduce this error
Every character in a Python string is assigned an index number to mark the position of that character in the string.
The index number starts from 0 and is incremented by 1 for each character in the string.
Suppose you create a string containing ‘Hello’ as follows:
my_string = "Hello"
The index numbers of the string are shown in the following illustration:
Following the illustration, this means that the maximum index number is 4 for the character ‘o’:
my_string = "Hello"
print(my_string[4])
print(my_string[5])
Output:
o
Traceback (most recent call last):
File "main.py", line 4, in <module>
print(my_string[5])
IndexError: string index out of range
As you can see, the character ‘o’ is printed when we access the string with 4 as the index position.
When we try to access the character at index 5, the error is raised. This is because there’s no character stored at that index number.
How to fix this error
To resolve this error, you need to avoid accessing the string using an index number that’s greater than the available indices.
One way to do this is to check the index number if it’s less than the length of the string as follows:
my_str = "Hello"
n = 5
if n < len(my_str):
print(my_str[n])
else:
print("Hey! Index out of range")
This code works because the index positions available in a string will always be less than the length of that string.
The len()
function counts the number of characters in a string from 1, while the index starts from 0.
By using an if-else
statement and comparing if the index number you want to use is less than the length, you won’t receive the error.
As an alternative, you can also use a try-except
block as follows:
my_str = "Hello"
try:
print(my_str[9])
except:
print("Hey! Index out of range")
By using a try-except
block, the error will be handled with a print()
statement so that your code execution doesn’t stop.
Conclusion
The IndexError: string index out of range
occurs when the index position you want to access is not reachable. Usually, the index position you specified is greater than or equal to the length of the string itself.
To resolve this error, you need to make sure that you’re using an index number that’s available within the string. You can use an if-else
or try-except
block to do so.
I hope this tutorial is helpful. Happy coding! 👍