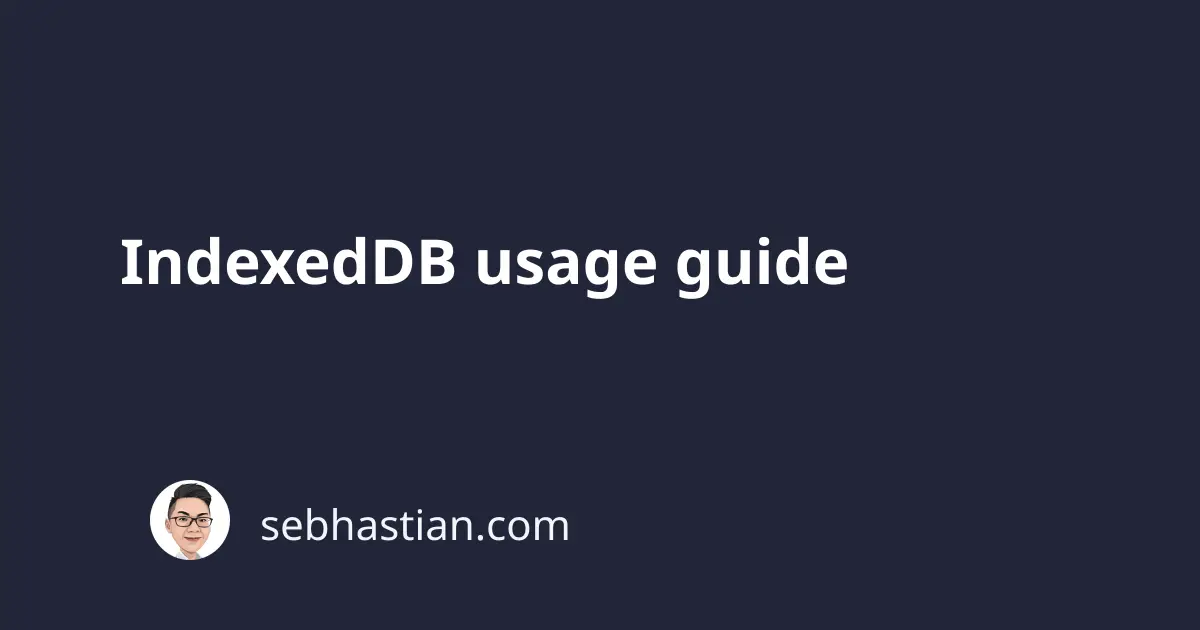
IndexedDB is the most updated web storage that can be used as a database similar to local storage. It was developed to solve some of local storage problems, mainly the limitation of 5MB storage and the synchronous execution. So yes, IndexedDB storage is unlimited and it’s API call is executed asynchronously.
IndexedDB accepts object as its data type.
The API guide
Just like local storage, IndexedDB is already implemented on most popular browsers like Chrome, Firefox and Safari. You can check if your current browser already support IndexedDB by running the following code on the console:
if (indexedDB) {
window.alert('IndexedDB is ready for some action!');
} else {
window.alert(
"Your browser doesn't support a stable version of IndexedDB. Such and such feature will not be available."
);
}
Create and open a database
IndexedDB use open
method to create, open and connect to the database. It accepts two argument — the database name and version
let request = indexedDB.open('mydatabase', 1);
If you have no IndexedDB named mydatabase
, it will be created and connected to your website. The open
method returns an open db object that can be used to create object store, add, update and remove data. We’re going to use the returned object to first create object store, which is the name of the object holding all our data.
request.onerror = function(event) {
// Handle errors.
};
request.onupgradeneeded = function(event) {
var db = event.target.result;
// the ObjectStore is just like a table in SQL type database
// we can data as the object store property
var objectStore = db.createObjectStore('developers');
var objectStore = db.createObjectStore('designers');
};
Now we need to open transaction before we can add, update or remove data from the database
Opening transaction
An IndexedDB transaction lifetime is tied to the event loop, meaning if there is no further command issued after opening the transaction, it will close itself and you have to open another one. To keep transaction active, you have to issue another command immediately after opening.
transaction
accepts 2 arguments: the array list of object store that can be accessed by the transaction and the optional permission. Permission defaults to read
, so if you want to add, update or remove data, you can use readwrite
permission.
You can then add new data by using the add
method. It accepts 2 arguments: object
type to store as data and string
type as key.
var db = request.result;
var transaction = db.transaction(['developers'], 'readwrite');
var objectStore = transaction.objectStore('developers');
// add a new data to developers object store
objectStore.add({ name: 'Nathan', email: '[email protected]' }, 'dev01');
In Chrome, you can inspect the newly saved data from the Applications tab
You can also shorten the code above by chaining transaction
and objectStore
methods.
var objectStore = db
.transaction(['developers'], 'readwrite')
.objectStore('developers');
objectStore.add({ name: 'Andrew', email: '[email protected]' }, 'dev02');
Get saved data
Simply use the get
method with the key
as argument, like:
// you can also chain get or add with onsuccess event handler
db
.transaction('developers')
.objectStore('developers')
.get('dev02')
.onsuccess = function(event) {
alert('Name for dev02 is ' + event.target.result.name);
};
Updating data
First, retrieve data using get
, update data by simple property assignment, then use put
method:
// you can also chain get or add with onsuccess event handler
var objectStore = db
.transaction('developers', 'readwrite')
.objectStore('developers');
objectStore.get('dev02').onsuccess = function(event) {
var data = event.target.result;
// change name
data.name = 'Dean';
objectStore.put(data, 'dev02').onsuccess = function(event) {
// the data is updated. Do the next step required here
};
};
If the data isn’t changed when you inspect it using the developer tool, try to hit the refresh button
Removing data
To delete saved data, simply use the delete
method from objectStore
object, which accepts key
argument for deleting:
var request = db
.transaction(['developers'], 'readwrite')
.objectStore('developers')
.delete('dev01');
request.onsuccess = function(event) {
// data with key dev01 has been deleted!
};
Conclusion
IndexedDB is a great client side database for storing more complex data than local storage. But since users can request to wipe data, and even delete it from the browser console, it can’t be used as a source of truth for most complex use cases. Just like local storage, IndexedDB might be best used for quick experiment and prototyping. But then again, if you need to go through the trouble of learning IndexedDB operation, why not just learn server side database like MongoDB, Firebase or MySQL? For one, they can’t be inspected through browser console and deleted by users.
This summary is sourced from MDN’s IndexedDB documentation, so if you want to learn more, feel free to dig in that article.