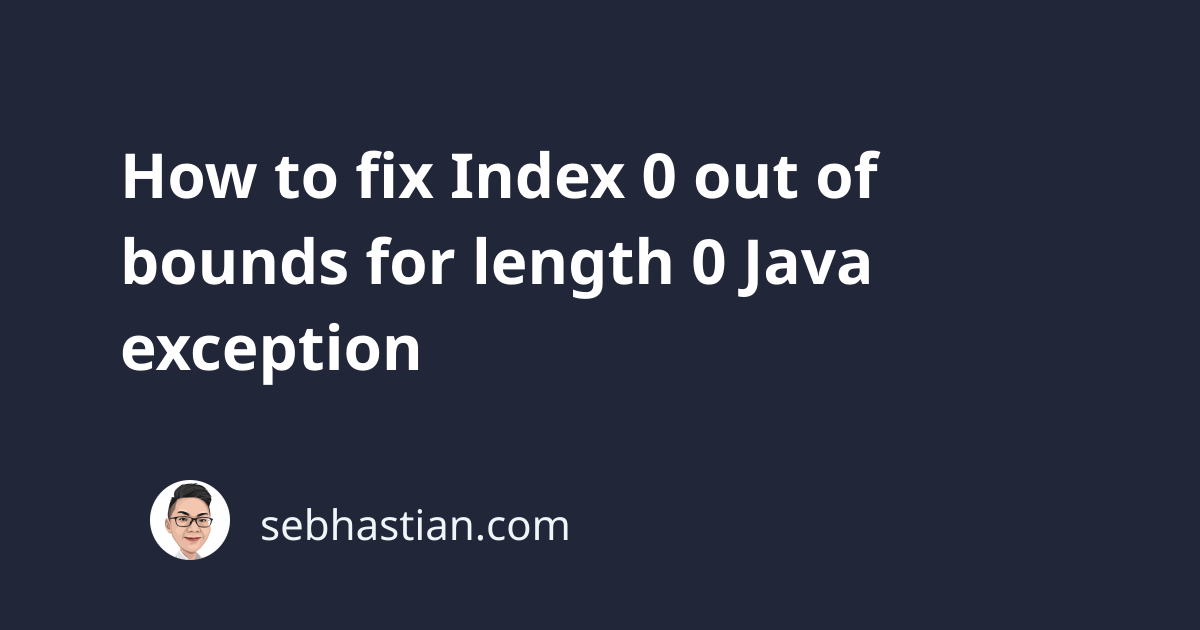
When running Java code, you might encounter the following error:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException:
Index 0 out of bounds for length 0
at Scratch.main(scratch.java:4)
This error occurs when you attempt to access an array at index 0, but the array is actually empty, so even the index 0 is out of bounds.
This tutorial will show you an example that causes this error and how to fix it in practice.
How to reproduce this error
Suppose you create an array of int
in your main()
method as follows:
public static void main(String[] args) {
int[] myArray = new int[0];
}
This myArray
variable has a length of zero because the initialization uses new int[0]
.
If you try to assign an element at index 0 as follows:
public static void main(String[] args) {
int[] myArray = new int[0];
myArray[0] = 2000;
}
You get the following error:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException:
Index 0 out of bounds for length 0
The error indicates that the array has a length of 0, so accessing the element at index 0 raised this exception.
How to fix this error
To resolve this error, you need to make sure that you’re accessing an element that is within the bounds of the array length.
When declaring an array, you need to determine the right length of that array because Java doesn’t allow you to resize the array later.
For example, here’s how to create an array with a length of 3:
int[] myArray = new int[3];
You can then assign elements to this array from index 0 to 2:
int[] myArray = new int[3];
myArray[0] = 92; // ✅
myArray[1] = 93; // ✅
myArray[2] = 94; // ✅
myArray[3] = 95; // ❌ out of bounds
When assigning to index 3, Java will throw the exception Index 3 out of bounds for length 3
.
To help you avoid this error, you can check the length
attribute of the array to determine its size:
public static void main(String[] args) {
int[] myArray = new int[3];
System.out.println(myArray.length);
double[] newArray = new double[10];
System.out.println(newArray.length);
}
Output:
3
10
The index number you can access in an array is the length
number - 1. You need to follow this rule to avoid the error.
Another way you can prevent this error is by adding a try-catch
block when accessing the array’s element:
public static void main(String[] args) {
int[] myArray = new int[3];
try {
// assigning an element at index 5
myArray[5] = 123;
} catch (Exception e) {
System.err.println("Error assigning element");
System.err.println("The array size is: " + myArray.length);
System.err.println("Don't assign or access element greater than or equal to the length");
}
System.out.println("Code finished running!");
}
When you attempt to assign or access an element that’s out of bounds, you’ll get this message:
Error assigning element
The array size is: 3
Please don't assign or access element greater or equal to the length
That should help you figure out what’s wrong with your code and resolve it properly.
Conclusion
The Java exception Index x out of bounds for length x
occurs when you specify an index number that’s greater than the array’s length
- 1.
To resolve this error, you need to make sure that you’re accessing an element using an index number that’s inside the bounds of the array’s length.
I hope this tutorial helps. Happy coding! 👍