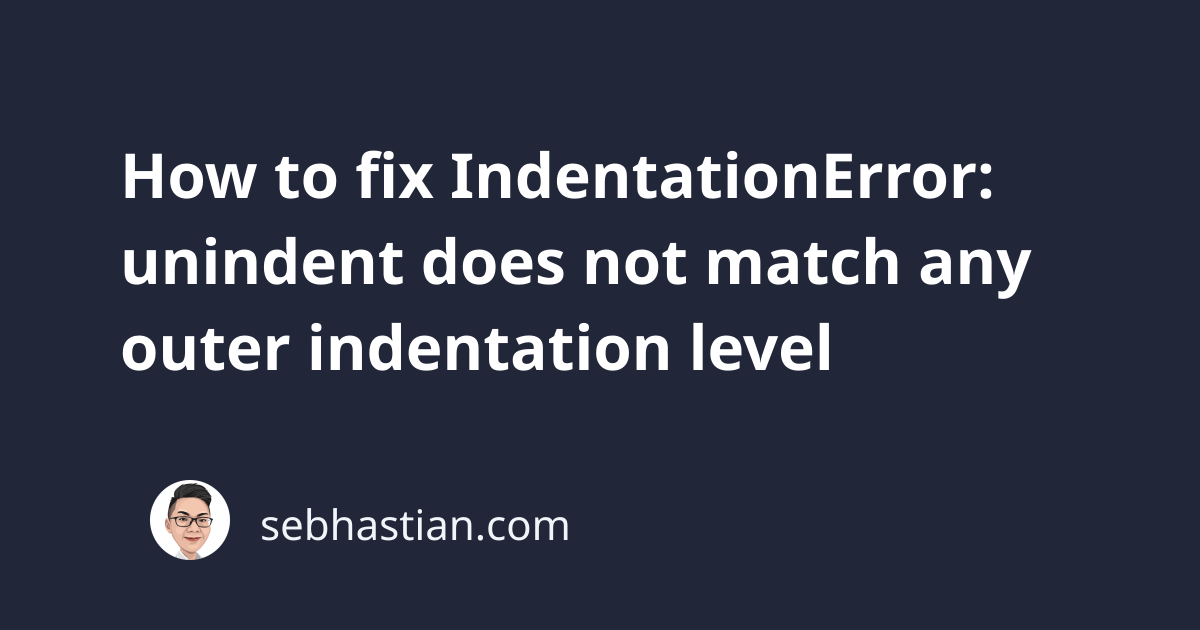
While working with Python source code, you might get the following error:
IndentationError: unindent does not match any outer indentation level
This error usually occurs because of two possible scenarios:
- You have different indentation levels for lines in the same block
- You mix the indentation with tabs and spaces
This tutorial will show you how to fix the error in both scenarios above.
1. You have different indentation levels for lines in the same block
Suppose you defined a function named walking()
with the following content:
print('top-level indent')
def walking():
print("Go left")
print("Go right") # ❌
The first print()
line uses two spaces to indent the line, while the second uses a single space.
When you run the script, you’ll get the following error:
File "main.py", line 5
print("Go right")
^
IndentationError: unindent does not match any outer indentation level
The error occurs because there’s no outer indentation level that matches the second print line. As a result, Python doesn’t know whether the second line is a part of the function or not.
In Python, code lines in the same block need to have the same indentation. If you use two spaces for the first line of a function, then all lines in the function must use two spaces as well.
To resolve this error, make the indentation level consistent as follows:
print('top-level indent')
def walking():
print("Go left")
print("Go right")
Notice how we don’t receive the error when running the code this time.
2. You mix the indentation with tabs and spaces
While you can use either tabs or spaces for code indentation, you can’t use both in one file because Python won’t be able to figure out the correct structure if you do.
Suppose you have a function named walking()
written as follows:
def walking():
print("Go left")
print("Go right")
The first print
line uses a tab while the second line uses a single space.
Mixing the indentation like this causes the following error:
File "main.py", line 3
print("Go right")
^
IndentationError: unindent does not match any outer indentation level
To avoid this error, you need to be consistent when indenting your source code.
In modern code editors like VSCode and Sublime Text, you will be notified of this problem by the red squiggly lines in your code as shown below:
You can also subtly see the different markings for the indentation, where the first line has a right arrow (→
) but the second line has a dot (·
) d for the indent.
If you didn’t see the markings, then open the command palette (Shift + Command + P
or Ctrl + Shift + P
) and search for render whitespace as follows:
You should be able to see the markings for the indentation now, helping you to resolve the indentation error.
I hope this tutorial is helpful. See you in other tutorials! 😉