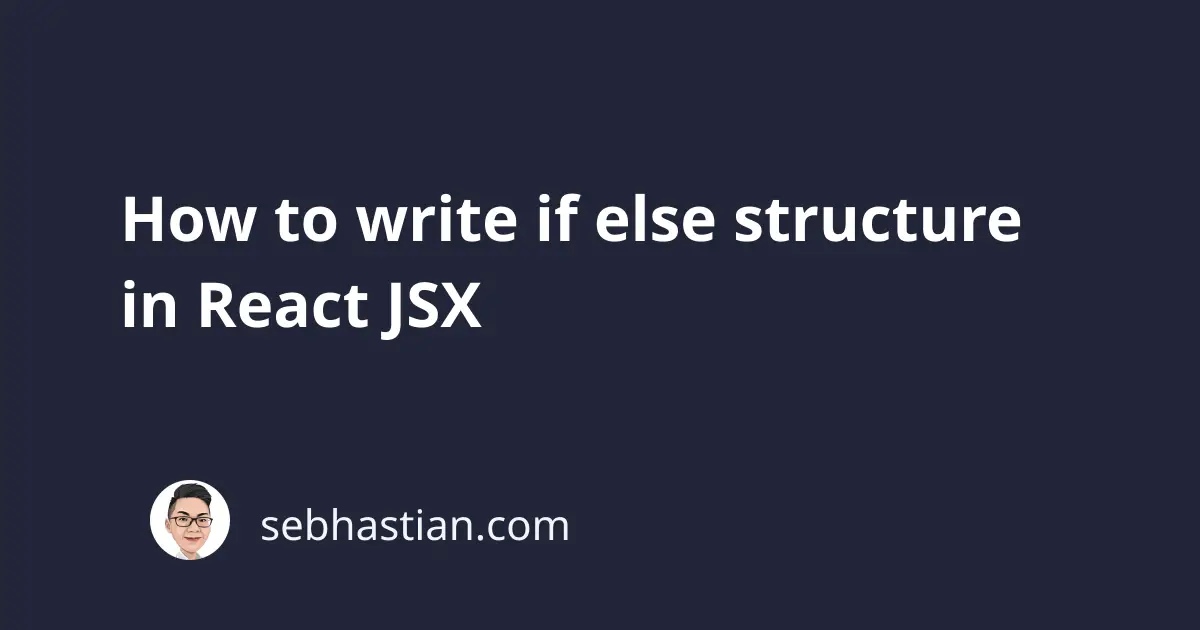
You can control what output is being rendered by your React component by implementing a conditional rendering in your JSX. For example, let’s say you want to switch between rendering login and logout button, depending on the availability of the user
state:
import React from "react"
function App(props) {
const {user} = props
if (user) {
return <button>Logout</button>
}
return <button>Login</button>
}
export default App
You don’t need to add an else
into your component, because React will stop further process once it reaches a return
statement. In the example above, React will render the logout button when user
value is truthy, and the login button when user
is falsy.
Partial rendering with a regular variable
There might be a case where you have want to render a part of your UI dynamically in a component. For example, you might want to render a button below a header element:
import React from "react"
function App(props) {
const {user} = props
let button = <button>Login</button>
if (user) {
button = <button>Logout</button>
}
return (
<>
<h1>Hello there!</h1>
{button}
</>
)
}
export default App
Instead of writing two return statements, you just need to store the dynamic UI element inside a variable (in this case, the button
variable)
This is convenient because you just have to write the static UI element only once.
Inline rendering with && operator
It’s possible to render a component only if a certain condition is met and render null otherwise. For example, let’s say you want to render a dynamic message for users when they have new emails in their inbox:
import React from "react"
function App() {
const newEmails = 2
return (
<>
<h1>Hello there!</h1>
{newEmails > 0 &&
<h2>
You have {newEmails} new emails in your inbox.
</h2>
}
</>
)
}
export default App
Inline rendering with conditional (ternary) operator
It’s also possible to use a ternary operator in order to render the UI dynamically. Take a look at the following example:
import React from "react"
function App(props) {
const {user} = props
return (
<>
<h1>Hello there!</h1>
{ user? <button>Logout</button> : <button>Login</button> }
</>
)
}
export default App
Instead of using a variable to hold the button element, you can simply use the ternary operator on the user
value and render Logout button when it’s falsy, or Login button when it’s truthy.
And that’s how you can do conditional rendering in React 👍