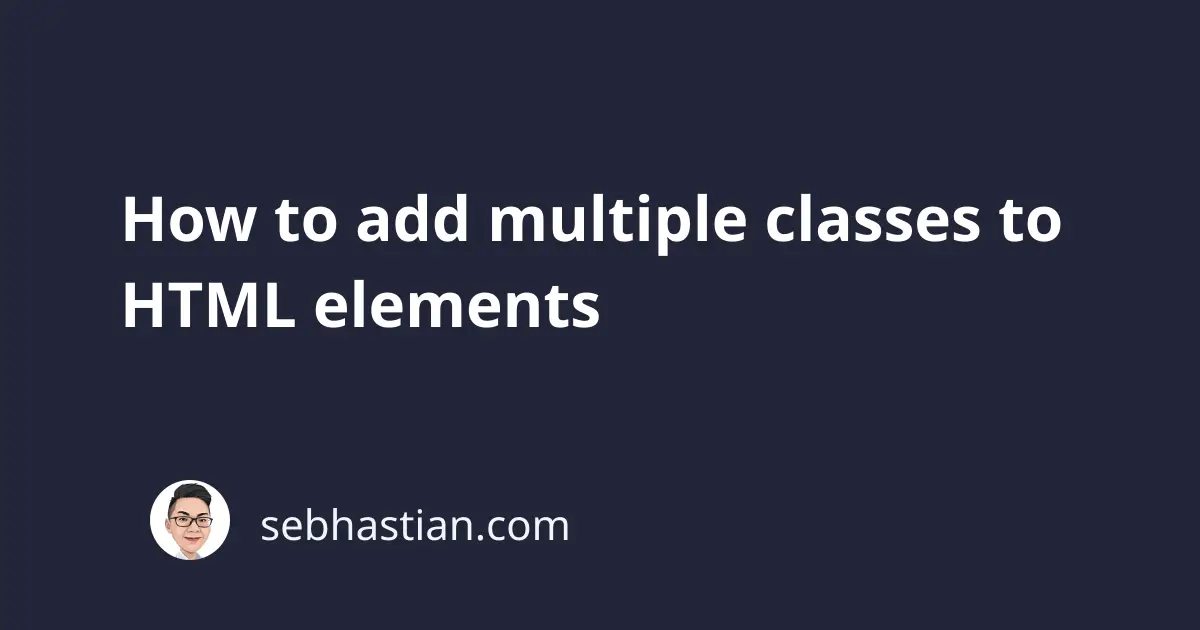
Any HTML element can have as many different classes as needed to style the element using CSS effectively.
To assign multiple classes to a single HTML element, you need to specify each class name inside the class
attribute separated with a blank space.
For example, the following <p>
element is assigned one class called heading
:
<p class="heading">
A paragraph with heading class
</p>
The following example below shows how to assign two classes: heading
and font-large
to the same paragraph:
<p class="heading font-large">
A paragraph with heading class
</p>
A class name that has more than one word is commonly joined with a dash (-
) this is also known as the kebab-case.
There is no limit to how many class names you can add to a single HTML element. But keep in mind that you need to keep the class names intuitive and descriptive.
I’d recommend you put 10 class names at maximum in a single HTML element to keep your project maintainable.
HTML multiple classes for styling purpose
HTML classes are used for styling the elements rendered on the browser.
By creating multiple classes that serve different styling purposes, you can reuse the classes in many different elements without having to repeat the styling.
For example, you can have a class called font-small
to adjust the font-size
property to smaller than regular font size, while the font-large
class will make the font-size
larger than regular.
Suppose you have a style sheet with the following CSS rules:
.font-large {
font-size: 20px;
}
.font-small {
font-size: 12px;
}
.font-color-grey {
color: grey;
}
.font-style-italic {
font-style: italic;
}
.heading {
font-weight: 700;
margin-bottom: 10px;
}
.bg-color-lime {
background-color: lime
}
You can add the CSS classes above to your HTML elements.
Here’s an example of adding the classes to a <div>
element:
<div class="bg-color-lime font-style-italic font-large">
Applying classes to a div element example
</div>
Here’s a rule of two that have helped me to write good class names for HTML elements:
- What property will this class modify? Use it as the first part of your class name.
- What is the value of the property being modified by this class? Use it as the second part of your class name
For example, if you’re changing the background-color
property to grey
, then the class name .bg-color-grey
would be a good choice.
If the value of the property is not exact, then you can use a word that would describe the output relative to a regular element.
For example, font-small
describes that the font size for the assigned element will be smaller than a regular element, so you can use that instead of font-size-12
although the latter is more precise.
Sometimes you can also abbreviate small
as sm
and create an xs
style for extra-small
:
.font-sm {
font-size: 12px;
}
.font-xs {
font-size: 8px;
}
Writing good HTML class names takes practice and time because classes can be used by both CSS and JavaScript to manipulate the presentation and the interactivity of HTML pages.
You’re going to get better as you write more code, and the rule of two above will help you start on the right mindset 👍
Now you’ve learned how to assign multiple classes to a single HTML element. Nice work!