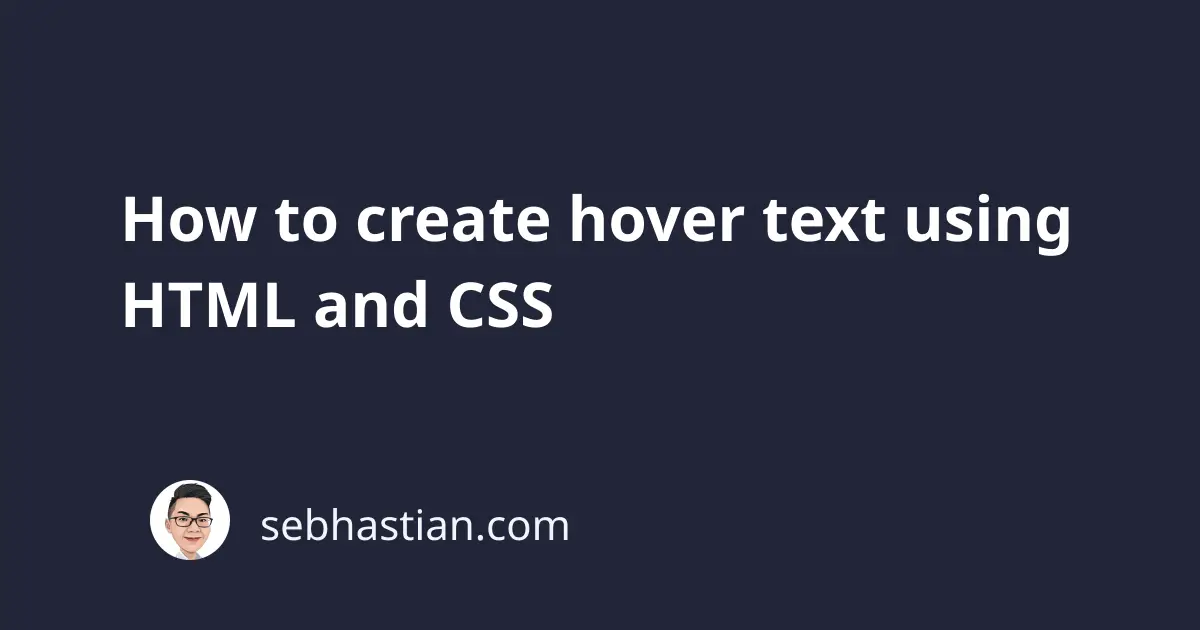
A hover text (also known as a tooltip text) is used to display additional descriptions over an HTML element. The text only appears when the mouse cursor hovers over an object.
There are two ways you can create a hover text for your HTML elements:
- Adding the global
title
attribute for your HTML tags - Creating a tooltip CSS effect using
:before
selector
This tutorial will show you how to use both methods.
Let’s start with learning how to create a hover text by adding the title
attribute to your HTML elements.
Create hover text by adding the title attribute
The global title
attribute is an attribute that you can add to any valid HTML tag to provide extra information about the element being rendered by the tag.
For example, the following title
attribute is being added to both <h1>
and <h2>
tags:
<body>
<h1 title="the message">Hello World</h1>
<h2 title="the sender">From Nathan</h2>
</body>
You can see a small hover text shown when the mouse hovers over the elements below:
As you can see from the example above, the title
attribute will be shown when the user hovers over the rendered elements using the mouse. You can add the title
attribute to any valid HTML element.
The hover text created from the title
attribute is set by the browser, which means you can’t customize the style of the display.
If you want a better looking hover text, then you need to create your own using CSS.
Create a hover text using HTML and CSS :before selector
The CSS :before
selector creates and inserts a pseudo-element before the content of the selected element, which is perfect for adding a hover text effect for your HTML elements.
To create a hover text using HTML and CSS, you need to group the display text and the hover text in one container element first:
<body>
<span class="hovertext" data-hover="Hello, this is the tooltip">
Try hover over me
</span>
</body>
The example above puts the hover text inside the data-hover
attribute, replacing the need to use the title
attribute to store the hover text.
Now you need to add CSS style to hide the hover text. The following CSS will do the trick:
.hovertext {
position: relative;
border-bottom: 1px dotted black;
}
.hovertext:before {
content: attr(data-hover);
visibility: hidden;
opacity: 0;
width: 140px;
background-color: black;
color: #fff;
text-align: center;
border-radius: 5px;
padding: 5px 0;
transition: opacity 1s ease-in-out;
position: absolute;
z-index: 1;
left: 0;
top: 110%;
}
.hovertext:hover:before {
opacity: 1;
visibility: visible;
}
The content
of the hovertext:before
element will be derived from the data-hover
attribute as specified in the selected element.
First, the position
property of the .hovertext:before
selector is set to absolute
so that it can be displayed outside of the document flow.
The absolute
element will be positioned relative to the closest container tag with the position:relative
value.
This is why the .hovertext
position property is changed to relative
so that the .hovertext:before
will be placed relative to the .hovertext
element.
Next, the visibility
of the .hovertext:before
is set to hidden to hide the text. The visibility
will be changed to visible
when the user hovers over the .hovertext
element.
The rest of the style for .hovertext:before
is used to improve the aesthetics of the hover text:
- A one second
transition
for the opacity of the.hovertext
element is added to improve the aesthetic of the hover text - The
z-index
is set to1
so that the hover text appears above the other text - The
top
andleft
property displays the edges of the hover text element
The resulting hover text will be as shown below:
By wrapping the hover text using the <span>
tag, you can place the element inside a <p>
or a <div>
tag:
<div>
Hello there!
<span class="hovertext" data-hover="Hello, this is the tooltip">
Try hover over me
</span>
</div>
Create dynamically resized hover text label
Finally, you can also dynamically resize the hover text label according to the size of your content.
To do so, you need to use max-content
as the value of the width
property in the .hovertext:before
rule.
Set your CSS as follows:
.hovertext:before {
content: attr(data-hover);
visibility: hidden;
opacity: 0;
width: max-content;
background-color: black;
color: #fff;
text-align: center;
border-radius: 5px;
padding: 5px 5px;
transition: opacity 1s ease-in-out;
position: absolute;
z-index: 1;
left: 0;
top: 110%;
}
The result will be as shown below:
And that’s how you can create hover text using CSS and HTML. You can customize the CSS style above as you need.