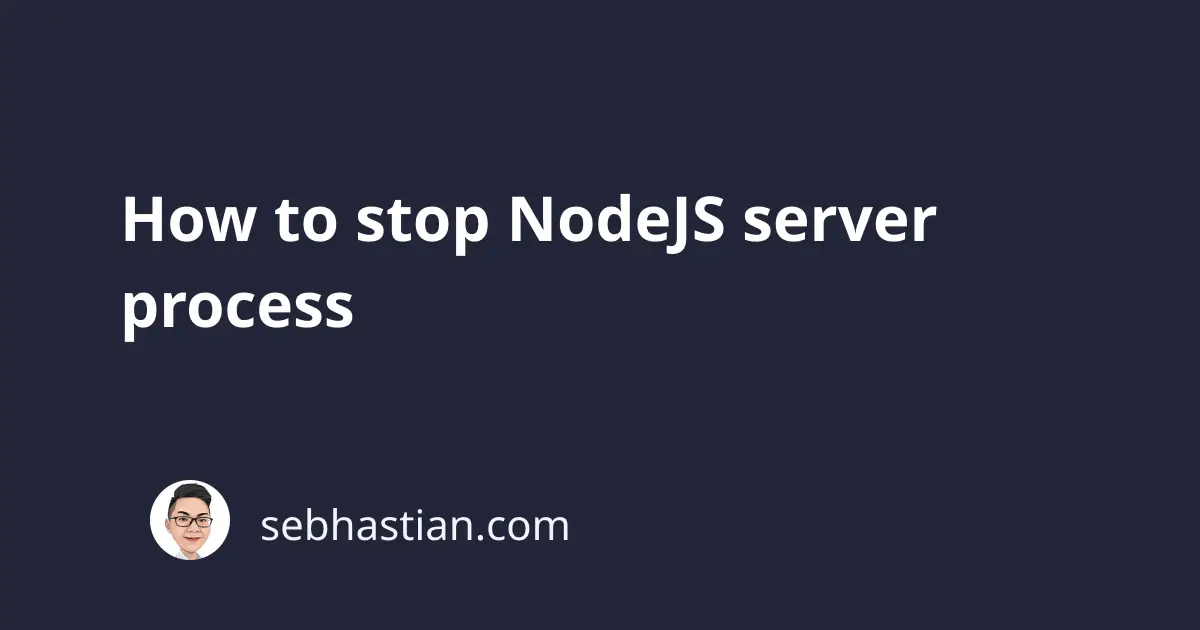
When you’re working with a full-stack JavaScript application, you will most likely start a NodeJS server and listen to a specific address.
For example, running the above NodeJS code will start a server that listens to localhost:3000
:
const http = require("http");
const server = http.createServer(function (req, res) {
const url = req.url;
if (url === "/") {
// do a 200 response
res.writeHead(200, { "Content-Type": "text/html" });
res.write("<h1>Hello World!<h1>");
res.end();
}
});
server.listen(3000, function () {
console.log("server started at port 3000");
});
Once the server starts listening, it will never stop until the interrupt signal or a code error crash the program.
To stop your NodeJS server from running, you can use the ctrl+C
shortcut which sends the interrupt signal to the Terminal where you start the server.
At other times, you may also want to stop your NodeJS program from running programmatically. This can be achieved by using the exit()
method from the process
module:
process.exit();
The code above will cause NodeJS to terminate all requests and stop the running server. For example, let’s create a new file named server.js
and call the exit()
method when the URL entered by the user matches "/close"
as follows:
const http = require("http");
const server = http.createServer(function (req, res) {
const url = req.url;
if (url === "/") {
// do a 200 response
res.writeHead(200, { "Content-Type": "text/html" });
res.write("<h1>Hello World!<h1>");
res.end();
}
if (url === "/close") {
console.log("Exiting NodeJS server");
process.exit();
}
});
server.listen(3000, function () {
console.log("server started at port 3000");
});
Run the code using node server
or node server.js
command, then navigate to localhost:3000/close
from your browser. The server will log "Exiting NodeJS server"
on the console and then shut itself down.
Keep in mind that turning off a NodeJS server using process.exit()
will interrupt all running connections and terminate them.
If you want to prevent the HTTP server from accepting new connections but still process all existing connections, you need to terminate your server by using the server.close()
method.
The server.close()
method will cause NodeJS to stop accepting new connections but finish all existing process before shutting down.
But since you can’t use the server
variable inside the createServer()
callback function, you need to use the process.send()
method to send a signal and the process.on()
method to receive the signal.
Here’s an example of a working NodeJS code:
const http = require("http");
const server = http.createServer(function (req, res) {
const url = req.url;
if (url === "/") {
// do a 200 response
res.writeHead(200, { "Content-Type": "text/html" });
res.write("<h1>Hello World!<h1>");
res.end();
}
if (url === "/close") {
process.send("STOP");
}
});
server.listen(3000, function () {
console.log("server started at port 3000");
});
process.on("STOP", function(){
console.log("Exiting NodeJS server");
server.close();
})
As you can see from the code above, the process.send()
method will send an event signal to the running NodeJS server. This signal can be pre-defined signals like 'beforeExit'
or 'disconnect'
, or something you self-defined.
On the code above, I send a self-defined signal named "STOP"
that causes NodeJS to look for the signal receiver that uses process.on()
method. When the signal is received by the process
module, The callback function passed to the on()
method will be executed.
And with that, you will be able to stop NodeJS server from running without killing any existing connections. Feel free to modify the code above as you need 😉