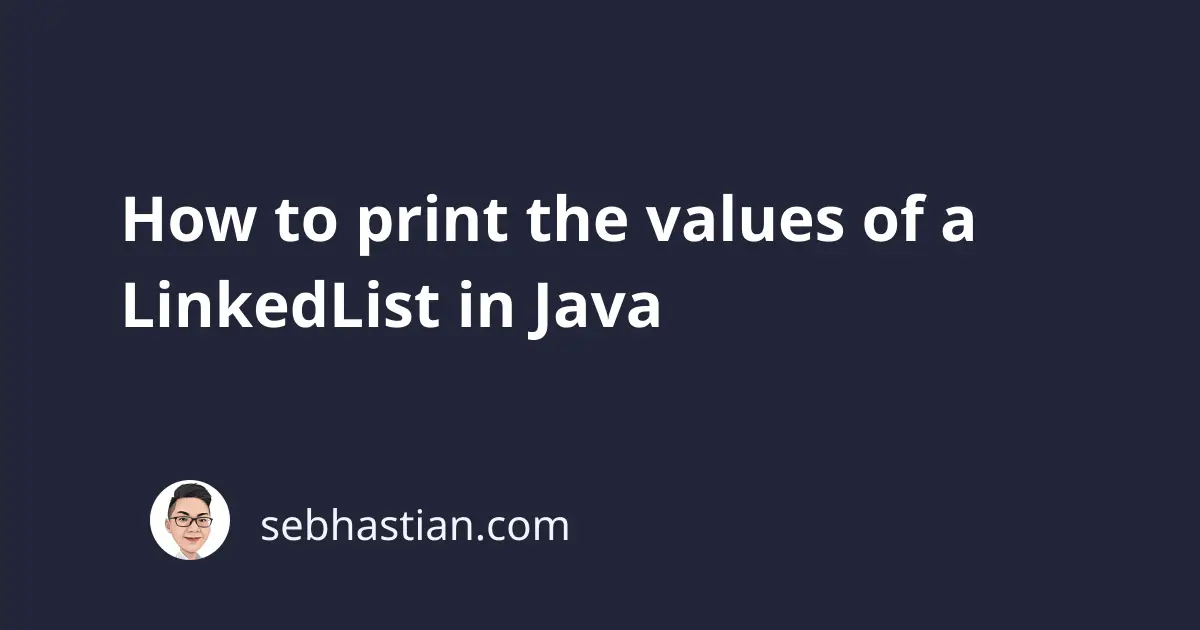
The Java LinkedList
class allows you to create a variable of LinkedList
type.
A LinkedList
instance can be created by calling the LinkedList()
constructor.
You can then add values to the instance by calling the add()
method as shown below:
LinkedList myList = new LinkedList();
myList.add("String");
myList.add(2);
myList.add(3);
When you need to display the values, you can print the LinkedList
instance using println()
method as shown below:
System.out.println(myList);
// [String, 2, 3]
To print only one of the values stored in the list, you can call the get()
method and pass the index where the value is stored as follows:
System.out.println(myList.get(0)); // String
System.out.println(myList.get(1)); // 2
System.out.println(myList.get(2)); // 3
Java also allows you to use the Iterator
object to print the values of a LinkedList
instance.
First, you need to create the Iterator object by calling the iterator()
method from the instance.
Then, you need to use the while
loop to let the Iterator
print all the elements stored in the list:
LinkedList myList = new LinkedList();
myList.add("String");
myList.add(2);
myList.add(false);
Iterator it = myList.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
The output in the Java console should look as follows:
String
2
false
Finally, you can also use an enhanced for
loop to print all the values of the list as shown below:
for (Object item : myList) {
System.out.println(item);
}
Now you’ve learned how to print a linked list instance values in Java. Good work! 👍