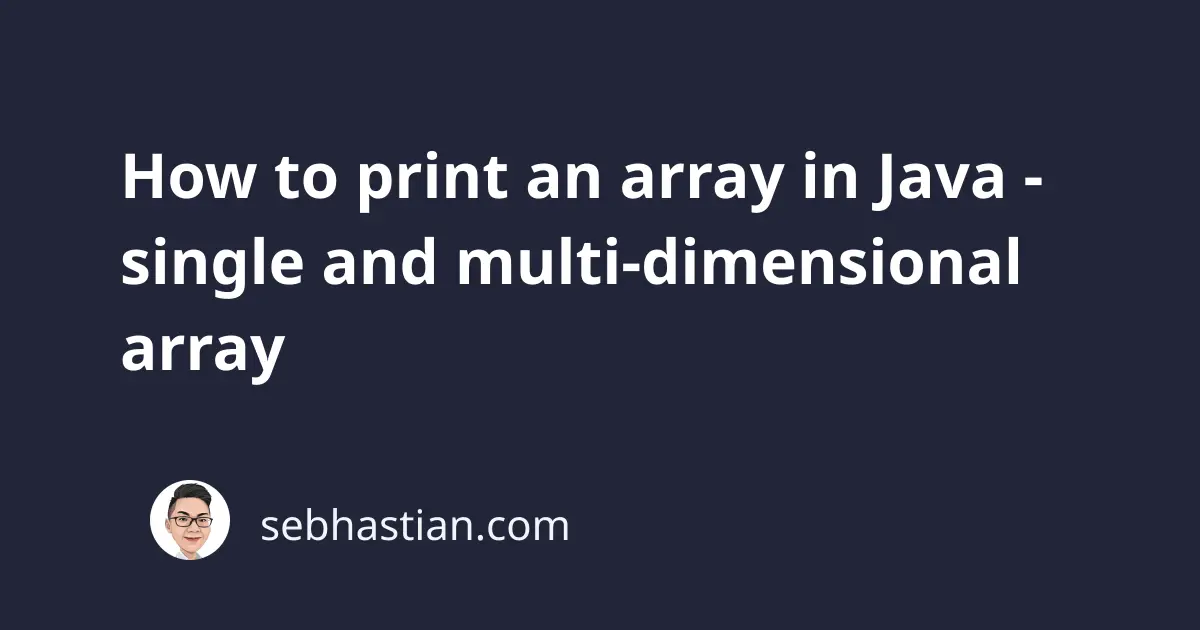
A Java array object can’t be printed directly to the console.
When you try to print an array in Java using the println()
method, the object hash code will be printed instead:
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4};
System.out.println(numbers);
// [I@1f32e575
}
To print the content of the array to the console, you need to call the Arrays.toString()
method from java.util.Arrays
class.
Pass the array you want to print as an argument to the Arrays.toString()
method as shown below:
import java.util.Arrays;
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4};
System.out.println(Arrays.toString(numbers));
// [1, 2, 3, 4]
}
However, keep in mind that the Arrays.toString()
method can’t be used to print a multi-dimensional array. Even a two-dimensional array will cause the method to print the hash code.
Instead of the toString()
method, you need to call the Arrays.deepToString()
method:
import java.util.Arrays;
public static void main(String[] args) {
int[][] numbers = {{1, 2}, {3, 4}};
System.out.println(Arrays.toString(numbers));
// [[I@1f32e575, [I@279f2327]
System.out.println(Arrays.deepToString(numbers));
// [[1, 2], [3, 4]]
}
The deepToString()
method will convert a multi-dimensional array into its string representation with no problem.
The reason you need to use the toString()
method for a one-dimensional array is that deepToString()
method will throw an error when used on a one-dimensional array.
Print a Java array using the for loop
Alternatively, you can also print a Java array using the for
loop as shown below:
public static void main(String[] args) {
String[] myArr = {"John", "Jane", "Doe"};
for (String element: array) {
System.out.println(element);
}
}
The output of the program above will be as follows:
John
Jane
Doe
When using a for
loop, each element of the array will be printed on a separate line and without the bracket notation for the array []
surrounding the elements.
For multi-dimensional arrays, you need to use multiple for
loops as much as the dimensions you have in your array.
For a two-dimensional array, you need two for
loops as shown below:
public static void main(String[] args) {
String[][] myArr = {{"John", "Doe"}, {"Jane", "Doe"}};
for (String[] elements: myArr) {
for(String element: elements){
System.out.println(element);
}
}
}
// Output:
// John
// Doe
// Jane
// Doe
Because the first loop will find an array of String
types, the String[]
type is used to type the elements
variable.
The second for
loop will be where the array element is stored, so you call the println()
in that second loop.
For other array types, you only need to match the type inside the for
loop to make it work:
public static void main(String[] args) {
int[][] myArr = {{1, 2}, {3, 4}};
for (int[] elements: myArr) {
for(int element: elements){
System.out.println(element);
}
}
}
// Output:
// 1
// 2
// 3
// 4
Now you’ve learned how to print an array in Java, both single and multi-dimensional arrays.
Good job! 👍