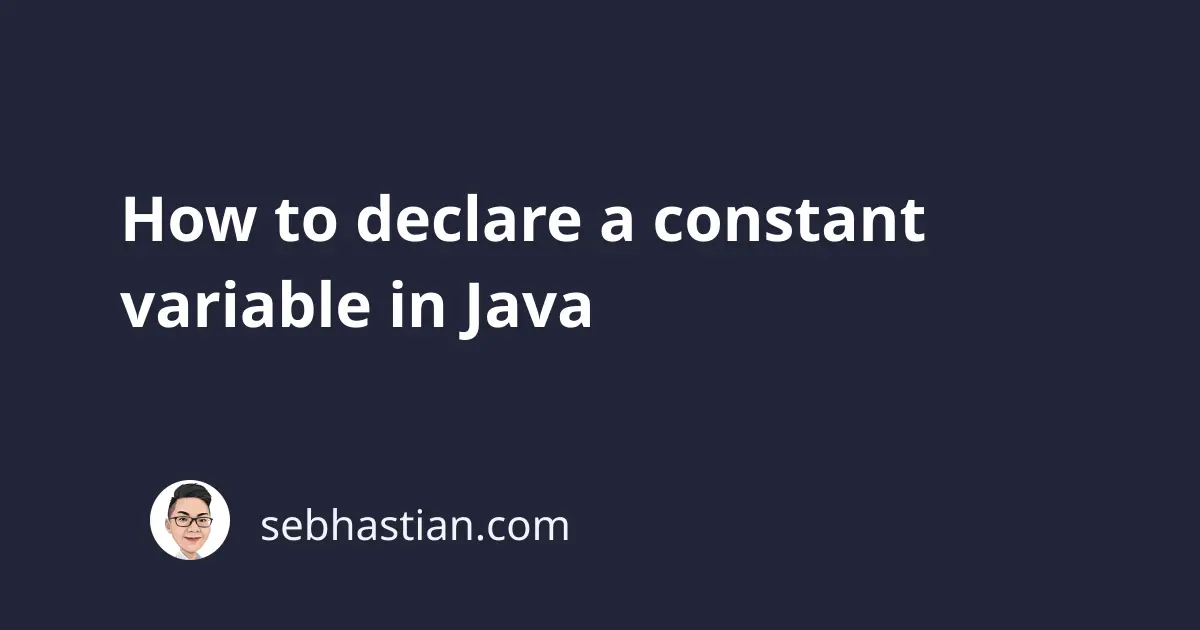
To declare a constant variable in Java, you need to use the final
and (or) static
modifiers when declaring your variable.
The Java final
modifier is used to create a variable that is fixed. You can’t change the value stored by a final
variable after it has been created.
Meanwhile, the static
modifier is used to make a variable accessible without the need of instantiating the class that owns it.
To create a constant variable, you need to use the final
modifier when initializing the variable as shown below:
class Main {
public static void main(String[] args) {
final String COLOR_RED = "#FF0000";
COLOR_RED = "(255,0,0)";
//^ ERROR: Cannot assign a value to final variable 'COLOR_RED'
}
}
In the example above, a constant String
type variable is defined with the name of COLOR_RED
. It contains the red color code in hex format.
Below the initialization, the COLOR_RED
variable is re-assigned with the red color code in decimal format.
When you try to compile the code above, Java will throw the Cannot assign a value to final variable 'COLOR_RED'
error.
By Java convention, a constant name should be all uppercase with words separated by an underscore.
Adding the static modifier to a Java constant
While the final
modifier is enough to make a constant variable, the static
modifier is usually added to make the variable accessible without having to generate the class
instance.
For example, suppose the constant variable is declared in a class
named Helper
as shown below:
class Helper {
final String COLOR_RED = "#FF0000";
}
When you try to call the variable from the main()
method, an error will occur:
class Main {
public static void main(String[] args) {
System.out.println(Helper.COLOR_RED);
//^ Non-static field 'COLOR_RED'
// cannot be referenced from a static context
}
}
To use the variable, you need to create an instance of the holding class
first like this:
class Main {
public static void main(String[] args) {
Helper helper = new Helper();
System.out.println(Helper.COLOR_RED); // valid
}
}
To avoid having to create an instance of the Helper
class, you need to define the COLOR_RED
variable as static.
When you do so, the variable should be accessible directly from the Helper
class:
class Helper {
static final String COLOR_RED = "#FF0000";
}
class Main {
public static void main(String[] args) {
System.out.println(helper.COLOR_RED);
}
}
But you are free to omit the static
modifier if you need the class
to be instantiated first before accessing the variable.
Now you’ve learned how to declare a constant variable in Java. Good job! 😉