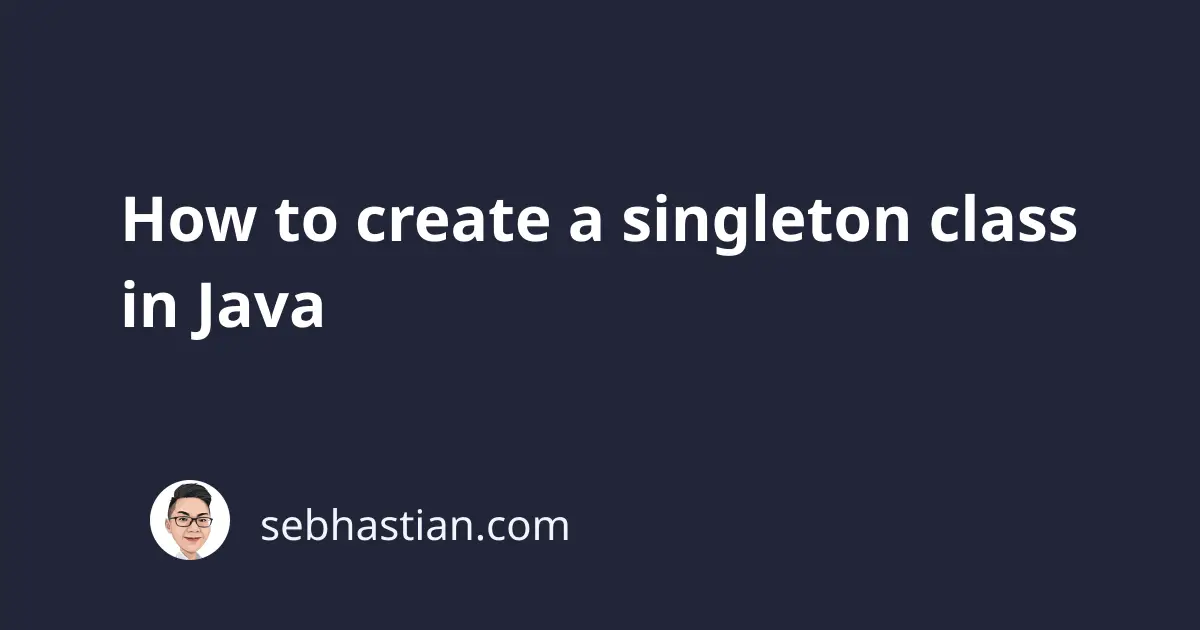
A singleton class is a class that has only one object instance created during the lifetime of the program.
In Java, a singleton class is a normal class that implements the singleton design pattern, allowing only one instance of the class to be created.
Here are the design patterns of a singleton class in Java:
- The class must provide a global access point to get the instance of the class. This is usually done with a
public static
method that returns an instance of the class - The class must have only one
private
constructor. Aprivate
constructor can only be accessed by the class, so this ensures that the class controls the instance creation - The class must have a
private static
attribute that stores the class instance
The code below shows an example of a singleton class named Single
:
class Single {
// Create an instance of the Single class
// save it inside a private static attribute
private static Single singleton = new Single();
// A private Constructor
// ensures only one instance created
private Single() {}
// Access to the class instance here
public static Single getInstance() {
return singleton;
}
}
The code above is a singleton class basic structure implemented with the early instantiation strategy.
When you run a program with a singleton class as defined above, a new instance of the class will be created as soon as the class is loaded.
If you want to optimize the singleton class even further, you can adopt the lazy instantiation strategy.
The instance will be created when the getInstance()
method is called for the first time:
class Single {
private static Single singleton = null;
private Single() {}
// Lazy instantiation
public static Single getInstance() {
if (singleton == null) {
singleton = new Single();
}
return singleton;
}
}
The singleton
instance will be initialized as null
. The instance will be created when the getInstance()
method is called for the first time.
To access the class instance, you call the getInstance()
method of the class.
In the example below, a
and b
will refer to the same object:
Single a = Single.getInstance();
Single b = Single.getInstance();
A method that creates an instance of the class is also known as a factory method. The getInstance()
method above is an example of a factory method.
You can add attributes and methods to a singleton class just like any other normal Java class:
class Single {
private static Single singleton = null;
private Single() {
}
public static Single getInstance() {
if (singleton == null) {
singleton = new Single();
}
return singleton;
}
// Add attributes and methods
String name = "Nathan";
void greetings(){
System.out.println("Hello World!");
}
}
You can access the attributes and methods of a singleton class as follows:
Single objInstance = Single.getInstance();
objInstance.addressee = "John";
objInstance.greetings(); // Hello, John!
And that’s how you create a singleton class in Java.
A singleton class is commonly defined when you need to control the access to the class instance, limiting the object creation number to one.