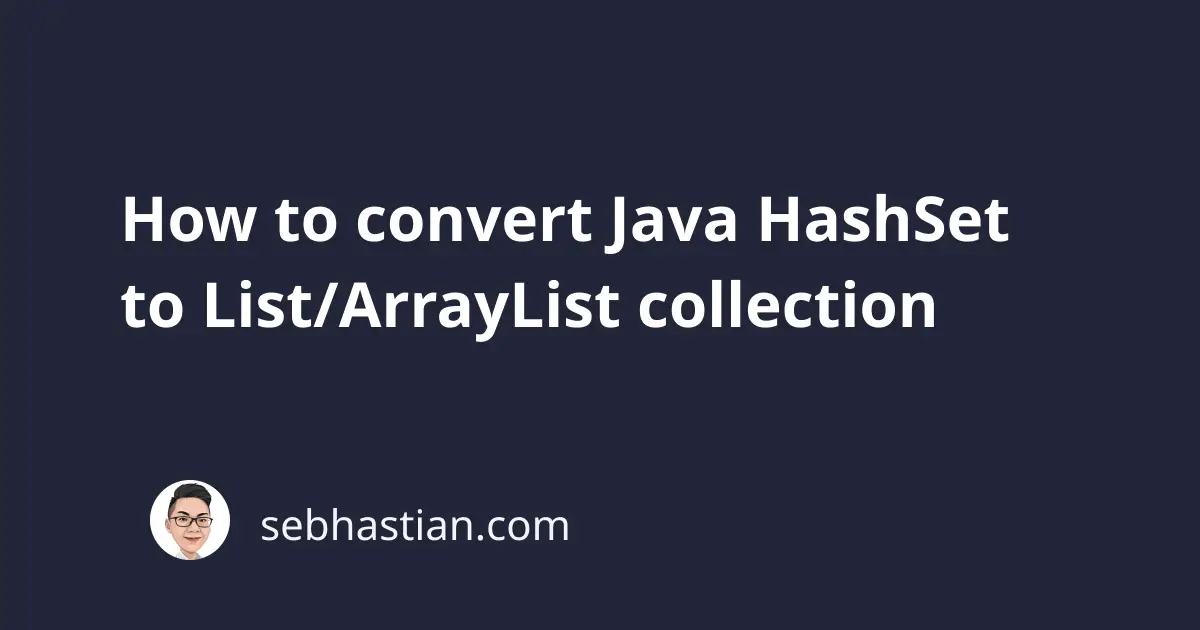
You can convert a HashSet
collection to an ArrayList
collection by passing the HashSet
instance as an argument to the ArrayList
constructor call.
Suppose you have the following HashSet
instance:
public static void main(String[] args)
{
HashSet<String> animalSet = new HashSet<>();
animalSet.add("Tiger");
animalSet.add("Lion");
animalSet.add("Hyena");
}
You can convert the animalSet
above by initializing an ArrayList
and passing the set as an argument to the constructor call as shown below:
public static void main(String[] args)
{
HashSet<String> animalSet = new HashSet<>();
animalSet.add("Tiger");
animalSet.add("Lion");
animalSet.add("Hyena");
ArrayList<String> arrList = new ArrayList<>(animalSet);
System.out.println("arrList content: " + arrList);
// arrList content: [Lion, Hyena, Tiger]
}
And that’s how you can convert a HashSet
to an ArrayList
.
If you want to convert the HashSet
into a List
to avoid using class implementation, you can swap the arrList
variable type from ArrayList
to List
as shown below:
public static void main(String[] args)
{
HashSet<String> animalSet = new HashSet<>();
animalSet.add("Tiger");
animalSet.add("Lion");
animalSet.add("Hyena");
List<String> list = new ArrayList<>(animalSet);
System.out.println("list content: " + list);
// list content: [Lion, Hyena, Tiger]
}
The result variable will be the same.