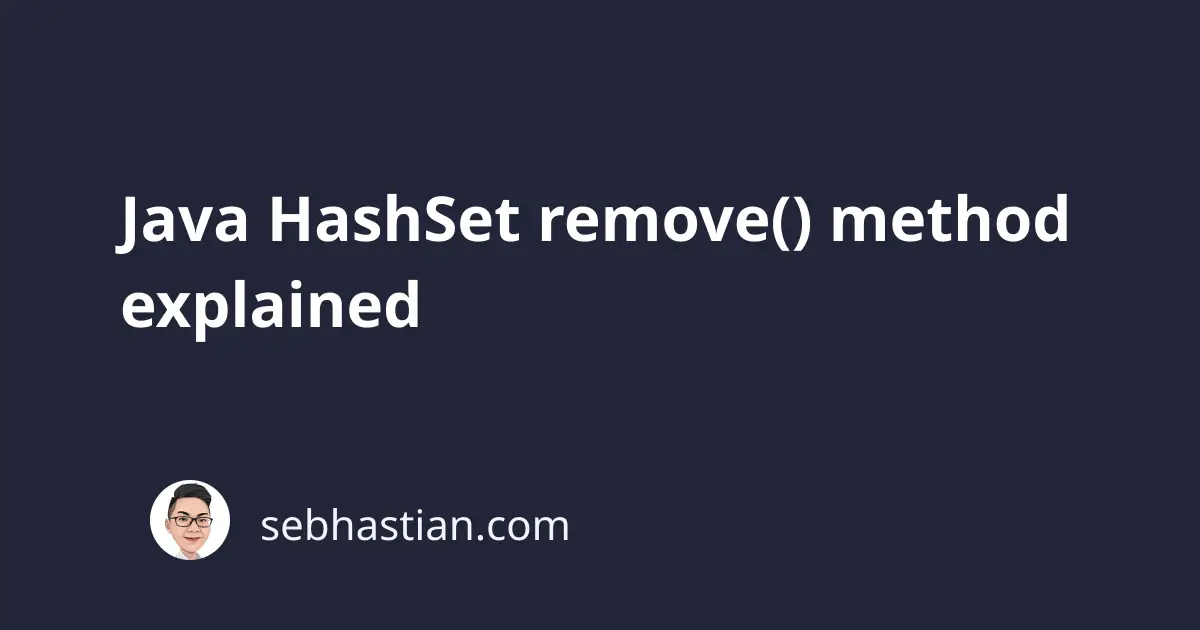
The Java HashSet
class implements the remove()
method from the Set
interface that you can use to remove an element from your HashSet
instance.
You need to specify the element to remove as the parameter to the method:
HashSet.remove(Object O);
The parameter you passed to the method assumes the Generic type Object
you passed to the HashSet
instance during initialization.
The remove()
method will return true
when an element is found and removed, or false
when it fails.
For example, suppose you create a HashSet
of <String>
type as shown below:
public static void main(String args[])
{
HashSet<String> mySet = new HashSet<>();
mySet.add("Tiger");
mySet.add("Lion");
mySet.add("Hyena");
}
Then you can remove an element from mySet
variable as follows:
public static void main(String args[])
{
HashSet<String> mySet = new HashSet<>();
mySet.add("Tiger");
mySet.add("Lion");
mySet.add("Hyena");
mySet.remove("Tiger");
System.out.println("HashSet content: " + mySet);
}
The output will be as shown below:
HashSet content: [Lion, Hyena]
Additionally, Java won’t throw an error when you pass an Integer
as the parameter of the remove()
method even though you use the <String>
Generic Type during initialization.
Java will return false
for any value not found inside the HashSet
even when the parameter type is different than your Generic Type:
public static void main(String args[])
{
HashSet<String> mySet = new HashSet<>();
mySet.add("Tiger");
mySet.add("Lion");
mySet.add("Hyena");
System.out.println(mySet.remove("Lion")); // true
System.out.println(mySet.remove("Horse")); // false
System.out.println(mySet.remove(92)); // false
}
You can use the return value of the remove()
method to inform the user whether the specified element is removed or not.