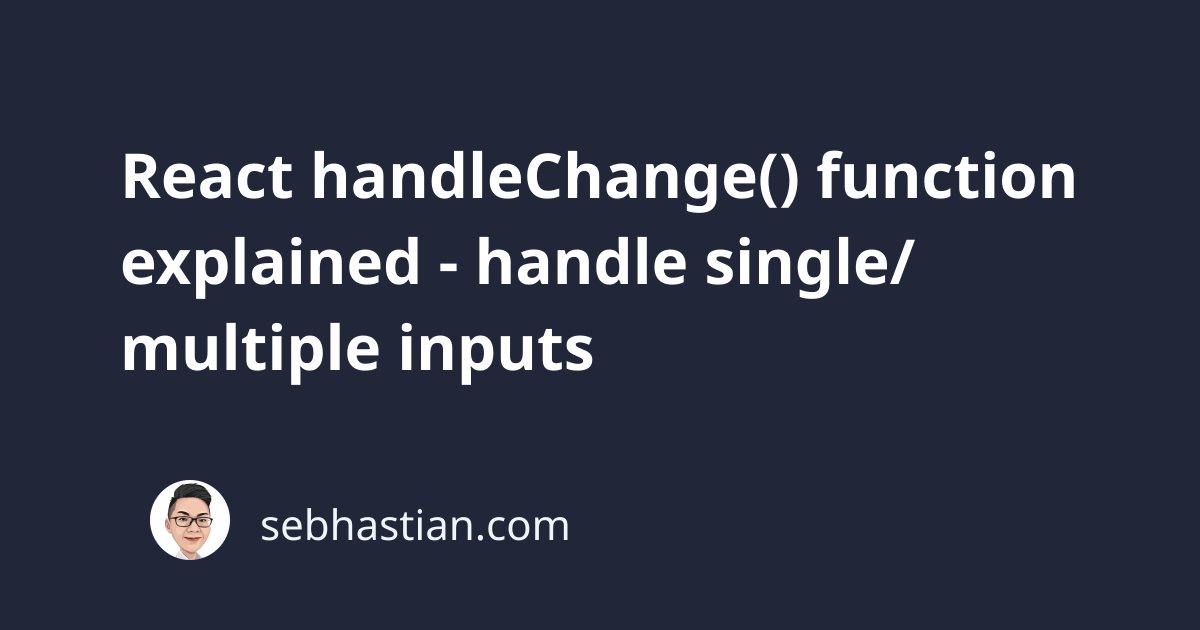
The handleChange()
function that you see in many React component examples is a regular function created to handle input change.
It’s commonly passed into an <input>
element’s onChange
property to intercept user’s input.
When the user types on your <input>
element, the onChange
event handler function will create an event
object and pass it to the handleChange()
function.
In that handleChange()
function, you can call your update state function to update the state of your component according to the value from event.target.value
property.
This tutorial will help you learn how to handle single or multiple inputs change using the handleChange()
function.
Let’s start with handling single input change
React handleChange() for single input change
Here’s an example of using handleChange()
function for a single <input>
element:
import React, { useState } from "react";
function App() {
const [name, setName] = useState("");
function handleChange(event) {
setName(event.target.value);
}
return (
<input
type="text"
name="name"
onChange={handleChange}
value={name} />
);
}
Any keyboard stroke inside the <input>
element will trigger the onChange
event handler, and the onChange
handler will call the handleChange()
function.
You can change the handleChange()
function name to other names. As long as the function is assigned to the onChange
property, then the function will be called anytime a change is triggered.
And that’s how you can handle the change from a single input element.
Let’s see how you can handle the change from multiple input elements in one state object.
React handleChange() for multiple input elements change
The event
object holds the detail about the event, but you only need to focus on two properties:
event.target.name
event.target.value
The event.target.name
value reflects the name
attribute that you specified in your <input>
element, while the event.target.value
property will reflect the latest value from the <input>
element.
By using both event.target.name
and event.target.value
property, you can group multiple <input>
elements value in one object state and use one handleChange()
function to properly update the state value.
First, create your <input>
elements and specify their name
attributes. The following example declares three <input>
elements inside the App
component:
import React, { useState } from "react";
function App() {
return (
<div>
<input
type="email"
name="email"
onChange={handleChange}
value={email} />
<br />
<input
type="text"
name="username"
onChange={handleChange}
value={username} />
<br />
<input
type="password"
name="password"
onChange={handleChange}
value={password} />
</div>
);
}
export default App;
Next, call the useState()
function and pass an object
that contains all your <input>
elements default value.
In the following example, the state is stored under formValue
variable:
function App() {
const [formValue, setFormValue] = useState({
email: "",
username: "",
password: "",
});
// return statement omitted for brevity...
}
Finally, create the handleChange()
function and pass the event
object as its parameter.
You can use the destructuring assignment to extract the name
and value
property from the event.target
object:
const handleChange = (event) => {
const { name, value } = event.target;
};
Call the setFormValue()
function below the destructuring assignment above, returning an object containing the expanded previous state value prevState
merged with the new value you get from the event.target
property.
The code for handleChange()
function is as shown below:
const handleChange = (event) => {
const { name, value } = event.target;
setFormValue((prevState) => {
return {
...prevState,
[name]: value,
};
});
};
With that, your handleChange()
function is ready to handle multiple inputs.
It will update the right state object property because you specify the state property names that match the <input>
elements name
attributes.
The full code for handling multiple input elements change is as follows:
import React, { useState } from "react";
function App() {
const [formValue, setFormValue] = useState({
email: "",
username: "",
password: "",
});
const handleChange = (event) => {
const { name, value } = event.target;
setFormValue((prevState) => {
return {
...prevState,
[name]: value,
};
});
};
const { email, username, password } = formValue;
return (
<div>
<input
type="email"
name="email"
onChange={handleChange}
value={email} />
<br />
<input
type="text"
name="username"
onChange={handleChange}
value={username} />
<br />
<input
type="password"
name="password"
onChange={handleChange}
value={password} />
</div>
);
}
export default App;
Feel free to use and tweak the example above for your React project 😉