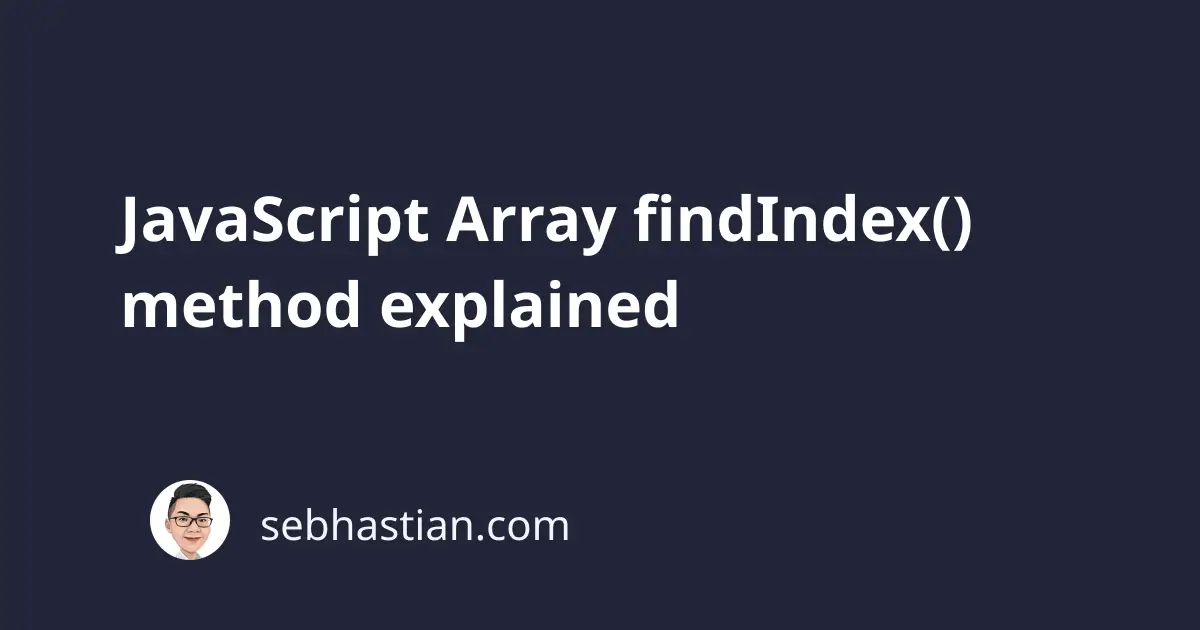
The JavaScript Array findIndex()
method is used to get the index of the first element that passed the function you specified as its argument.
The method returns -1
when there is no matching element.
The code below shows an example of using the findIndex()
method:
const numbers = [-3, 2, 5, 7, 25];
const idx = numbers.findIndex(function (element) {
// returns the first element that's greater than 3
return element > 3;
});
console.log(idx); // 2
Since the findIndex()
method is defined in the Array.prototype
constructor, you can call it from any valid array you defined in your code.
In the example above, the first element in the array that’s greater than 3
is 5
.
The value is positioned at index 2
, so the number 2
is returned by the findIndex()
method.
The findIndex()
method will iterate over the function
you defined as its argument, sending each element to test against the function
.
You can pass an anonymous function as in the example above, or you can also pass a named function as shown below:
function largerThanThree(element) {
return element > 3;
}
console.log([4, 5, 6].findIndex(largerThanThree)); // 0
console.log([1, 2, 3].findIndex(largerThanThree)); // -1
The benefit of a named function is that you can reuse it on as many arrays as you need.
The full syntax of the findIndex()
method is as follows:
Array.findIndex(function (element, index, array) {
/* test function body */
}, thisArg);
The findIndex()
method accepts two arguments:
- The
function
to test your Array elements against - The
thisArg
to replace thethis
reference inside the test function.
The code below shows the difference between using the thisArg
argument and without:
function greaterThanZero(element) {
console.log(this);
return element > 0;
}
[1, 2, 3].findIndex(greaterThanZero, "Hello!");
// Output is "Hello!"
[1, 2, 3].findIndex(greaterThanZero);
// Output is the Window object
Without the thisArg
argument, the this
keyword inside the test function will be the global object of the JavaScript environment.
Finally, three arguments are always passed into the test function by the findIndex()
method:
- The current
element
being processed by the method - The
index
of the currentelement
- The full
array
where thefindIndex()
method was called from
You are free to use or ignore the arguments in your function as shown below:
// These are all valid findIndex syntax
Array.findIndex(function (element) { });
Array.findIndex(function (element, index) { });
Array.findIndex(function (element, index, array) { });
The test function
will be executed on each value in the array until the value true
is returned, signaling that an element has passed the test.
If no true
value is returned by the function after the last element in the array has been tested, the method will return -1
to signal that no matching element is found.
findIndex() method caution
When you define a conditional statement using the findIndex()
method, keep in mind that the evaluation will return false
if the matching element is at index 0
.
Consider the following example:
function greaterThanZero(element) {
return element > 0;
}
if ([1, 2].findIndex(greaterThanZero)) {
console.log("Element found!");
} else {
console.log("Element NOT found!");
}
// output: Element NOT found!
The above example will log "Element NOT found!"
because the findIndex()
method returns 0
, which is evaluated as false
in JavaScript conditionals.
You need to explicitly check if the returned value is equal to -1
when using the findIndex()
method:
function greaterThanZero(element) {
return element > 0;
}
const idx = [1, 2].findIndex(greaterThanZero);
if (idx != -1) {
console.log("Element found!");
} else {
console.log("Element NOT found!");
}
// output: Element found!
Since the findIndex()
method always returns -1
when no matching element is found, you can easily check the result with the not equal operator (!= -1
)
Now you’ve learned how the findIndex()
method of the Array
object works in JavaScript. Great work! 😉