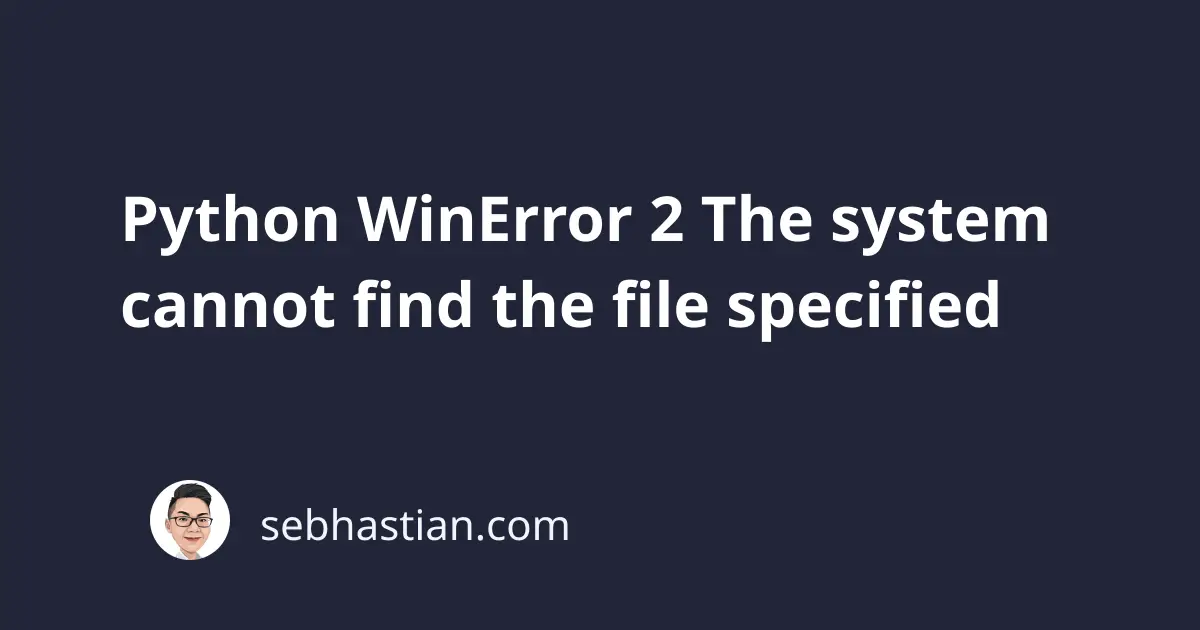
When interacting with the Operating System in Python, you may encounter the following error:
FileNotFoundError: [WinError 2] The system cannot find the file specified
This error usually occurs when you try to access a file in Windows Operating System.
One code example that causes this error is when you call the os.rename()
method as follows:
import os
os.rename('file.txt', 'output.txt')
The following sections show examples that cause the error and how to fix it.
1. You specify the wrong file name
Suppose you have the following files in your current directory:
.
├── file1.txt
└── main.py
Next, suppose you want to change the file name from file1.txt
to output.txt
.
You then write the following code in main.py
file:
import os
os.rename('file.txt', 'output.txt')
Because there’s no file named file.txt
in the current working directory, Python responds with the following error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
os.rename('file.txt', 'output.txt')
FileNotFoundError: [WinError 2] The system cannot find the file specified:
'file.txt' -> 'output.txt'
To fix this error, you need to make sure that you’re specifying the old file name correctly.
In the example above, file.txt
needs to be replaced with file1.txt
:
import os
os.rename('file1.txt', 'output.txt') # ✅
With this, the error should disappear and the file renamed successfully.
2. The file is in another directory
When renaming files with os.rename()
, it’s possible that the file you want to rename is in a different directory.
Suppose you have the following directory structure:
.
├── assets
│ └── image.png
└── main.py
The image.png
file is inside the assets/
folder, so if you run the following code in main.py
:
import os
os.rename('image.png', 'photo.png')
You’ll get the same error as before! You need to specify the right relative path from the directory where you run main.py
to reach the file you want to rename.
The right code to rename the file is as follows:
import os
os.rename('assets/image.png', 'assets/photo.png')
You need to specify the relative path to the file both in the old_name
and new_name
arguments for the os.rename()
method.
3. You don’t have Administrator access
Sometimes, this error occurs when you try to create a virtual environment with the venv
module.
Suppose you run the following command from the Command Prompt:
python venv my_env
The error response is as follows:
Error: [WinError 2] The system cannot find the file specified:
'C:\Users\sebhastian\documents\my_env'
This error usually happens because the Command Prompt has no permission to create files and folders.
To fix this, open the Command Prompt as Administrator and run the script again.
4. Sublime Text: Can’t find python.exe
If you’re running Python scripts using Sublime Text, you might get this error when Sublime Text can’t find the python.exe
file from your PATH environment.
The error is as follows:
[WinError 2] The system cannot find the file specified
[path: C:\Users\Home\AppData\Local\Programs\Python\Python39\;
C:\Users\Home\AppData\Local\Programs\Python\Python39\Scripts\;
C:\Users\Home\AppData\Local\Programs\Python\Python39\]
Open the path shown above in your Windows Explorer. If you can see the python.exe
file exists, then the problem might be with the Build System for Python in Sublime Text.
If you use a custom Build System, try this simple configuration first:
{
"cmd": ["python", "$file"]
}
If it works, then you probably misconfigured the Build System for Python.
For more information on creating Build Systems, please see the Sublime Text Build Systems documentation
Conclusion
You’ve seen four different examples that can cause the [WinError 2] The system cannot find the file specified
in Python and how to fix it.
Most likely, this error occurs when a file that’s needed doesn’t exist. It’s possible that the file has been renamed, deleted, or moved from the directory where you expect to find it.
I hope this tutorial is helpful. Thanks for reading! 🙏