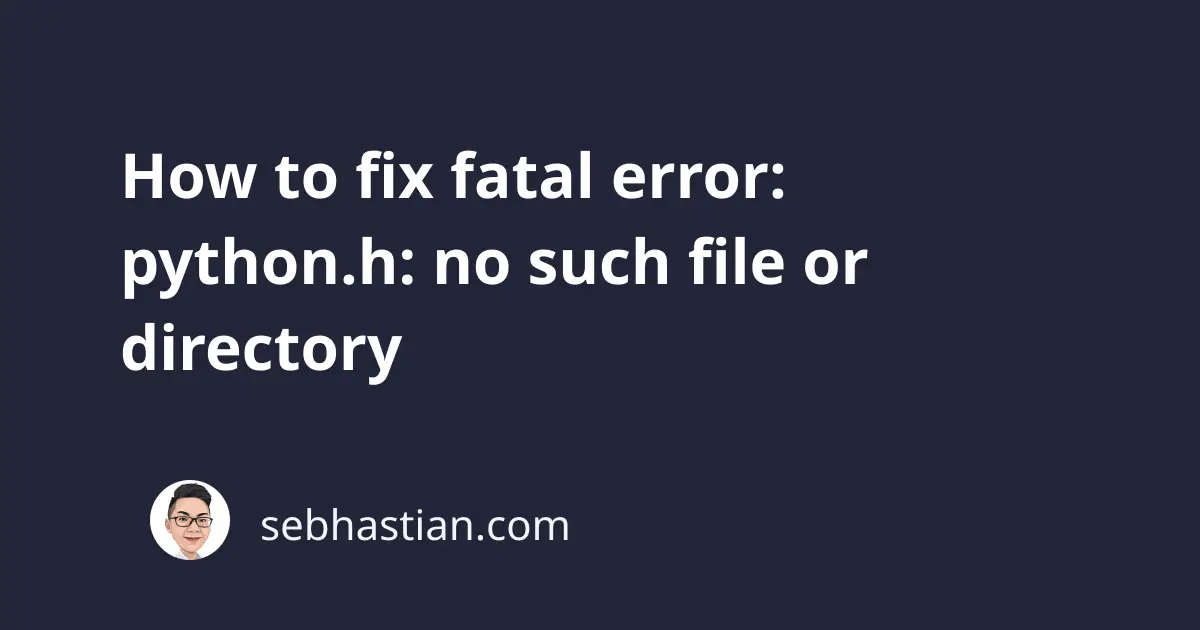
When you compile C and C++ code using GCC, you might see the following error:
fatal error: Python.h: No such file or directory
This error usually occurs when you add the Python header file to your C code, but that file can’t be found on your system.
This tutorial shows examples that cause this error and how you can fix it.
How to reproduce this error
Suppose you have a file named main.c
with the following content:
#include<Python.h>
#include<stdio.h>
int main()
{
printf("Hello World!\n");
return 0;
}
You instructed the program to include the Python.h
header file, then compile it using GCC:
gcc main.c
When GCC can’t find the Python.h
file in your system, it shows the following error:
main.c:1:9: fatal error: Python.h: No such file or directory
1 | #include<Python.h>
| ^~~~~~~~~~
compilation terminated.
To fix this error, you need to make GCC able to find the Python header file.
How to fix this error
If you use Ubuntu OS, the Python.h
file is included in the python-dev
package.
You can use one of the following commands to install the python-dev
package.
If you have Python 3, then install the python3-dev
package:
#For apt (Ubuntu, Debian...):
sudo apt-get install python-dev
sudo apt-get install python3-dev
#For yum (CentOS, RHEL...):
sudo yum install python-devel
sudo yum install python3-devel
# For dnf (Fedora...):
sudo dnf install python2-devel
sudo dnf install python3-devel
#For zypper (openSUSE...):
sudo zypper in python-devel
sudo zypper in python3-devel
# For apk (Alpine...):
sudo apk add python2-dev
sudo apk add python3-dev
#For apt-cyg (Cygwin...):
apt-cyg install python-devel
apt-cyg install python3-devel
Once you install the Python-dev package, you could find the Python.h
file in /usr/include/pythonx.xx
with x.xx
being the version of the Python you installed.
You can also use the locate
command to find the file:
$ locate Python.h
/usr/include/python3.10/Python.h
Now that you have the location of Python.h
, you need to include the path to that file by adding the -I
flag when running GCC.
Here’s an example:
gcc main.c -I/usr/include/python3.10
Notice you no longer get the fatal error. You can run the output file a.out
to run the compiled C program.
Fix for macOS
If you use macOS, then the header file should be included when you install Python on your computer.
If you use Homebrew to install Python, the header file should be somewhere in /opt/homebrew/Cellar/
for Apple Silicon Macs or in /usr/local/Cellar
for Intel Macs.
I found my header file in /opt/homebrew/Cellar/[email protected]/3.10.10/Frameworks/Python.framework/Versions/3.10/include/python3.10
.
You can use the Search bar in Finder to find the file. Find the location by right-clicking on the file and select Get Info.
You can highlight and copy the path shown in the Where info.
Next, you need to include the path when compiling your code with GCC like this:
gcc main.c -I/opt/homebrew/Cellar/[email protected]/3.10.10/Frameworks/Python.framework/Versions/3.10/include/python3.10
As an alternative, you can also use the python-config --includes
command to generate the include option.
For example:
$ python3-config --include
-I/opt/homebrew/opt/[email protected]/Frameworks/Python.framework/Versions/3.11/include/python3.11
You can add the command when calling gcc
to compile your C program as follows:
gcc main.c `python-config --include`
The compilation no longer gives a fatal error, and you can run the compiled program.
Conclusion
The fatal error: Python.h: No such file or directory
occurs when GCC can’t find the Python.h
file you included in your code.
You might not have the python-dev
package installed on your system, or the path to Python.h
file isn’t included in the PATH variable.
You can add the -I
flag when compiling with GCC to include the path to the directory that holds the header file.
I hope this tutorial is helpful. Happy coding! 😉