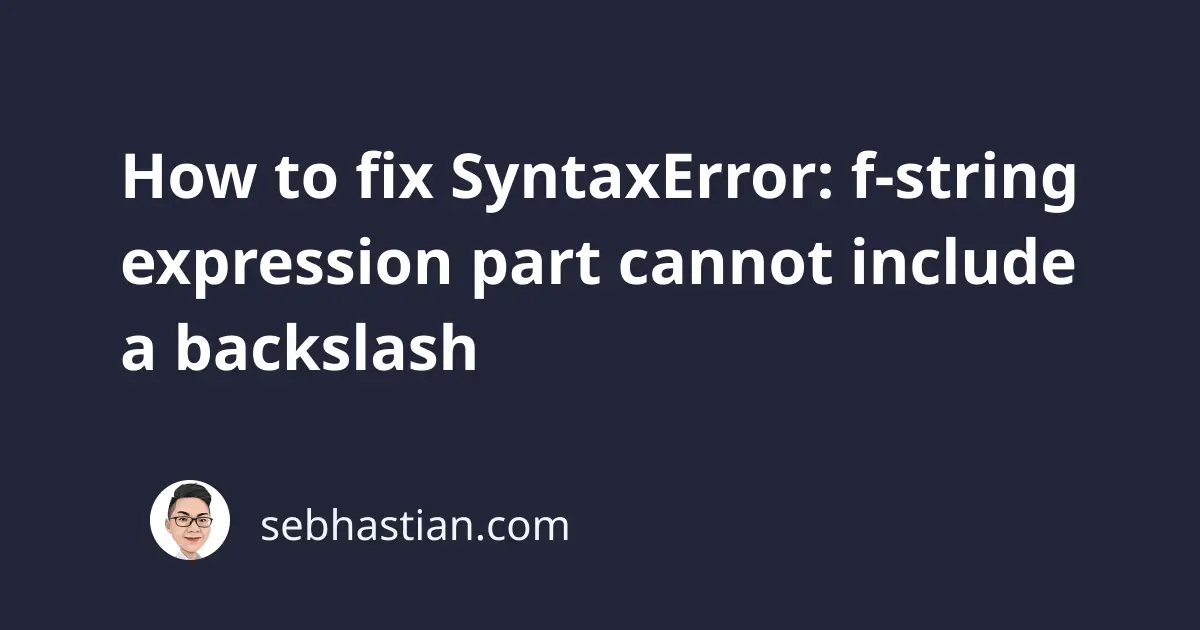
When using the f-string format in Python, you might encounter the following error:
SyntaxError: f-string expression part cannot include a backslash
This error occurs when you include a backslash character between the curly brackets in the f-string content.
You’re probably trying to include escape characters such as \n
and \t
inside your f-string.
This tutorial will show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you have two string variables for a name as shown below:
first_name = "Nathan"
last_name = "Sebhastian"
Now suppose you want to print these two variables using the f-string format. A tab \t
is included to separate the strings as follows:
print(f"{first_name + '\t' + last_name}")
When you run the code, you’ll get this error:
File "/Users/nsebhastian/Desktop/DEV/python/main.py", line 4
print(f"{first_name + '\t' + last_name}")
SyntaxError: f-string expression part cannot include a backslash
This error occurs because the escape character for tab \t
is included inside the curly brackets, which is the expression part of the f-string format.
The problem is that an f-string doesn’t allow you to put a backslash character even when you’re not adding an escape character. Even a single backslash will cause an error:
print(f"{first_name + '\\' + last_name}") # ❌ SyntaxError
How to fix this error
To resolve this error, you need to put the escape character outside of the curly braces like this:
first_name = "Nathan"
last_name = "Sebhastian"
print(f"{first_name}\t{last_name}") # ✅
Output:
Nathan Sebhastian
Notice that you don’t receive any error this time.
When you want to include escape characters such as \t
or \n
in an f-string format, you need to make sure that you’re placing the character outside of the curly braces.
If you really need to include the characters inside the curly braces, then you need to use a variable to do so.
For example, here’s how to add the tab \t
character using a variable:
first_name = "Nathan"
last_name = "Sebhastian"
# Create a variable for the escape character
tab = '\t'
print(f"{first_name + tab + last_name}") # ✅
By using a variable, you can circumvent the limitation and include a backslash character in the f-string format.
Here’s another example:
first_name = "Nathan"
last_name = "Sebhastian"
backslash = "\\"
print(f"{first_name + backslash + last_name}") # ✅
Output:
Nathan\Sebhastian
Notice that the backslash
variable is embedded in the expression part of the f-string format just fine.
This solution also works when you need to join a list using a newline character to format the output.
Consider the example below:
friends = ['John', 'Jane', 'Andy']
newline = "\n"
print(f"Friend list: \n{newline.join(friends)}")
Here, we used newline.join()
instead of '\n'.join()
to avoid the error. The output will be:
Friend list:
John
Jane
Andy
As you can see, using a variable is a convenient way to get around the f-string limitation.
Conclusion
Python shows the SyntaxError: f-string expression part cannot include a backslash
when you attempt to include a backslash inside the expression part of the f-string format.
The expression part is everything inside the curly braces in the string. To resolve this error, you can assign the backslash character as a variable and put that variable in the expression part.
I hope this tutorial helps. Happy coding! 👍