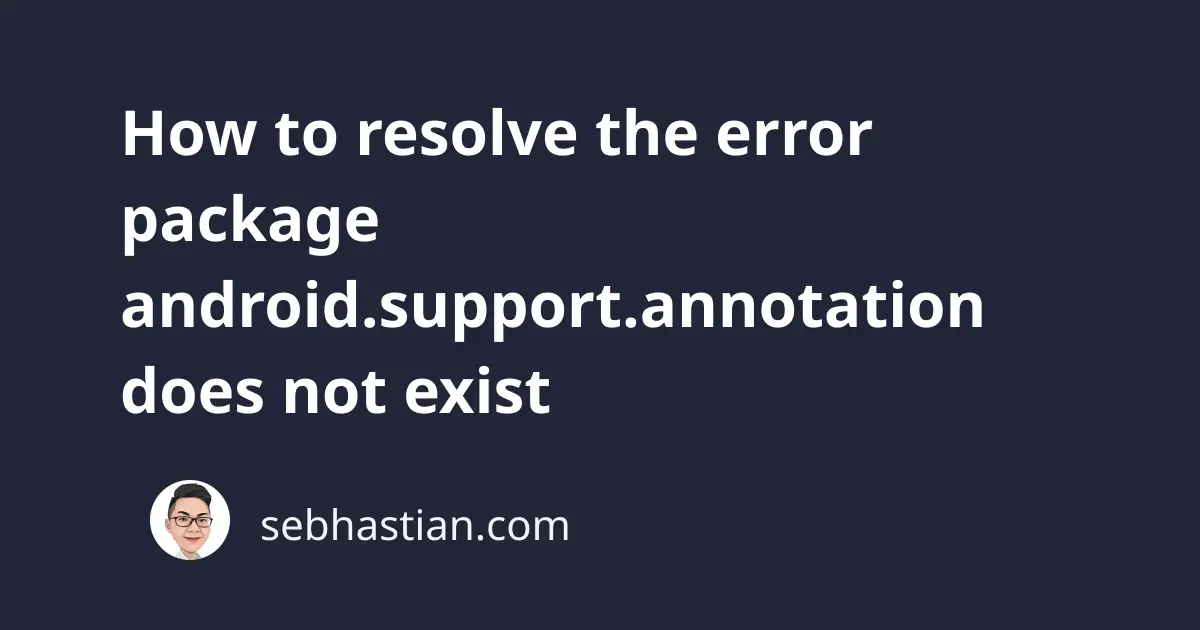
When building your Android application, you might find an error saying that the package android.support.annotation
does not exist.
Here’s an example of the error:
error: package android.support.annotation does not exist
error: cannot find symbol class NonNull
Error:Execution failed for task ':app:compileDebugJavaWithJavac'.
Usually, the error above happens when you migrate your Android application to use androidx
libraries.
androidx
is the namespace used by Android Jetpack libraries. It’s a group of Android support libraries that help you in developing an Android application.
The androidx
packages fully replace the android.support
packages, and new features will be added to androidx
.
When you use androidx
, you need to update your Android source code and replace all import
statements for android.support.annotation
package with androidx.annotation
package.
Here’s an example of replacing the import
statement that uses FloatRange
annotation:
// import android.support.annotation.FloatRange;
import androidx.annotation.FloatRange;
public class Color {
@FloatRange(from=0.0,to=1.0)
public float makeOpaque() {
return (float) 0.5;
}
}
Instead of replacing the import
lines one by one, you can try to use the Android Studio’s AndroidX migration feature to help you automate the process.
Before you migrate to AndroidX, please update your annotation library dependencies to version 28.0
first:
dependencies {
implementation 'com.android.support:support-annotations:28.0.0'
}
This is required because AndroidX version 1.0.0
is equivalent to support library version 28.0.0
.
If you use lesser support library versions, other errors may occur when building your application after migration.
To start the migration, add the following two lines to your gradle.properties
file:
android.useAndroidX=true
android.enableJetifier=true
Next, select Refactor > Migrate to AndroidX from your Android Studio top menu.
Once you complete the review and let Android Studio do the refactor, the required androidx
dependencies should be added to your build.gradle
file.
You should also see the import
lines being replaced with the new ones.
If you still see the build error after migrating to AndroidX, then you need to replace the import
statements manually.
Right-click on your application package to open the context menu, then select the Replace in Files… menu as shown below:
In the pop-up window, aim to replace android.support.annotation
with androidx.annotation
for all of your files.
The following screenshot should help:
Click Replace if you want to review the refactor one by one, or click on Replace All to refactor all occurrences at once.
The issue with the annotations library should now be resolved.
Resolve annotation error in React Native applications
If you’re building an Android application using React Native, then you need to also run the jetifier
package after doing all of the steps above.
Note: You need to migrate the React Native Android application using Android Studio by opening the android/
folder in Android Studio.
When you migrate your native Java code using Android Studio, the React Native modules in the node_modules
folder may still contain code that uses the Android support library.
The jetifier
module refactors Java code included in your React Native application to use androidx
instead of android.support
namespaces.
After migrating to AndroidX using Android Studio, run the following steps with NPM:
npm install --save-dev jetifier
npx jetify
Or if you use Yarn:
yarn add jetifier
yarn jetify
The import
statements in your node_modules
folder that uses old Android support libraries will be replaced with androidx
.
Once done, you should be able to run your React Native app:
npx react-native run-android
You might also want to include the call to jetify
in your postinstall
script:
"scripts": {
"postinstall": "jetify"
}
When you update your Node module dependencies with npm install
or npm update
, you need to run the jetify
command again.
Adding jetify
as the postinstall
command will let Node automatically run it after installation.
Now you’ve learned how to fix the android.support.annotation
package does not exist error in your Android application.
Thanks for reading! I hope this tutorial has been useful for you. 🙏