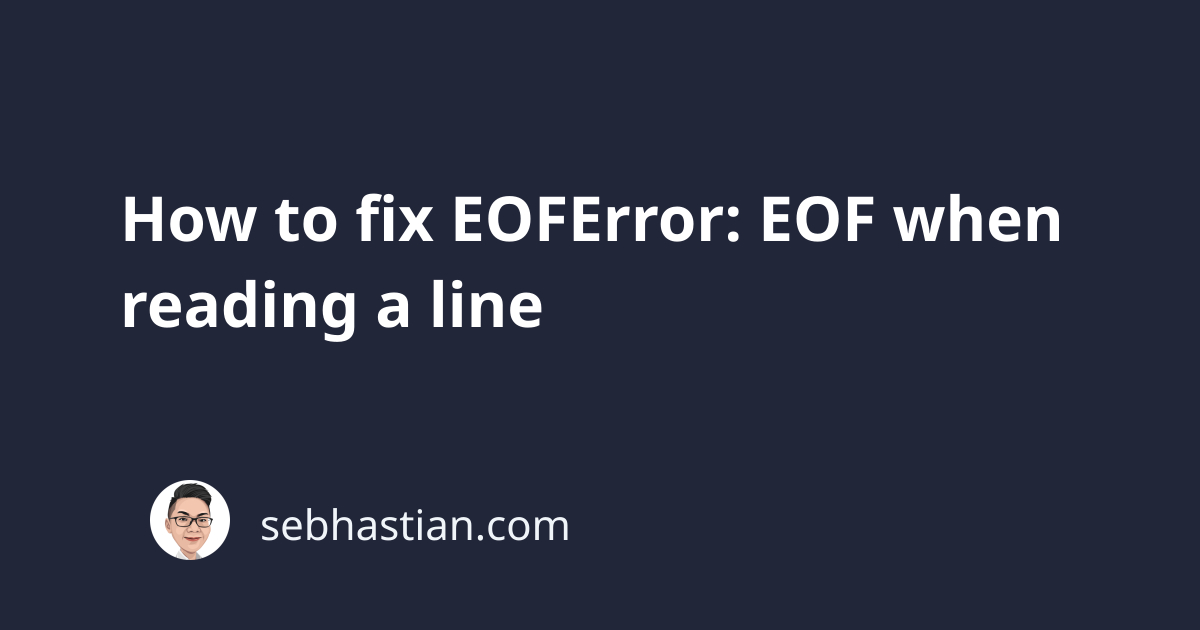
When using the input()
or raw_input()
function in Python, you might see the following error:
EOFError: EOF when reading a line
This error usually occurs when the input()
function reached the end-of-file (E0F) without reading any input.
There are two scenarios where this error might occur:
- When you interrupt the code execution with
CTRL + D
while aninput()
function is running - You called the
input()
function inside awhile
loop - You’re using an Online Python IDE and you don’t provide any input in STDIN
This tutorial will show how to fix this error in each scenario.
1. Interrupting code execution with CTRL + D
Suppose you have a Python code as follows:
my_input = input("Enter your name: ")
print("Hello World!")
Next, you run the program, and while Python is asking for input, you pressed CTRL + D
. You get this output:
Enter your name: Traceback (most recent call last):
File "main.py", line 1, in <module>
my_input = input("Enter your name: ")
EOFError
This is a natural error because CTRL + D
sends an end-of-file marker to stop any running process.
The error simply indicates that you’re not passing anything to the input()
function.
To handle this error, you can specify a try-except
block surrounding the input()
call as follows:
try:
my_input = input("Enter your name: ")
except EOFError as e:
print(e)
print("Hello World!")
Notice that there’s no error received when you press CTRL+D
this time. The try-except
block handles the EOFError
successfully.
2. Calling input()
inside a while statement
This error also occurs in a situation where you need to ask for user input, but you don’t know how many times the user will give you input.
For example, you ask the user to input names into a list as follows:
names = list()
while True:
names.append(input("What's your name?: "))
print(names)
Next, you send one name to the script from the terminal with the following command:
echo "nathan" | python3 main.py
Output:
What's your name?: ['nathan']
What's your name?: Traceback (most recent call last):
File "main.py", line 11, in <module>
names.append(input("What's your name?: "))
EOFError: EOF when reading a line
When the input()
function is called the second time, there’s no input string to process, so the EOFError
is raised.
To resolve this error, you need to surround the code inside the while
statement in a try-except
block as follows:
names = list()
while True:
try:
names.append(input("What's your name?: "))
except EOFError as e:
print(names)
break
This way, the exception when the input string is exhausted will be caught by the try
block, and Python can proceed to print the names
in the list.
Suppose you run the following command:
echo "nathan \n john" | python3 main.py
Output:
What's your name?:
What's your name?:
What's your name?: ['nathan ', ' john']
When Python runs the input()
function for the third time, it hits the end-of-file marker.
The except
block gets executed, printing the names
list to the terminal. The break
statement is used to prevent the while
statement from running again.
3. Using an online IDE without supplying the input
When you run Python code using an online IDE, you might get this error because you don’t supply any input using the stdin box.
Here’s an example from ideone.com:
You need to put the input in the stdin box as shown above before you run the code, or you’ll get the EOFError
.
Sometimes, online IDEs can’t handle the request for input, so you might want to consider running the code from your computer instead.
Conclusion
This tutorial shows that the EOFError: EOF when reading a line
occurs in Python when it sees the end-of-file marker while expecting an input.
To resolve this error, you need to surround the call to input()
with a try-except
block so that the error can be handled by Python.
I hope this tutorial is helpful. Happy coding! 👍