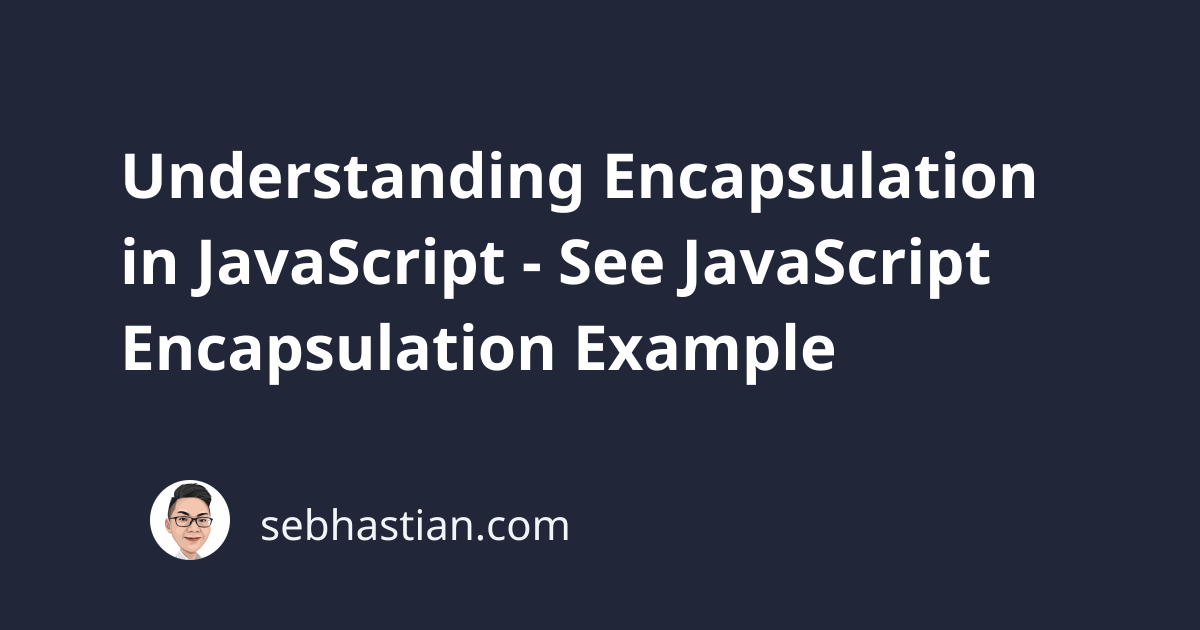
In object oriented programming, encapsulation is the process of bundling data and methods that change the data as one, making access to the data impossible without using the methods intended for changing that data.
The method for accessing the data is also known as the getter method. And the method for changing the data is the setter method.
Encapsulation in JavaScript is like making the properties of an object private. You expose only the methods used to get or modify the object properties.
The main benefit of encapsulation is that it helps you to create objects without having their internals modified in unintended ways.
When you use encapsulation, you can be sure that the object will be modified in a predictable way. This makes your code more robust against errors.
Generally, objects you created in JavaScript are not encapsulated as you can access and change their properties.
For example, the following person
object has a name
property:
let person = {
name: "Nathan"
};
console.log(person.name); // Nathan
person.name = "Sebhastian";
console.log(person.name); // Sebhastian
As you can access and change the name
property of the person
object directly, the person
object is not encapsulated.
Encapsulation can be implemented in a JavaScript class
or function
.
Let’s see how you can implement encapsulation in a JavaScript class
first.
Encapsulation in a Javascript class
You can create a class
that acts as the blueprint of the person
object above.
To encapsulate the class properties, you need to declare the properties as private using the hashtag symbol #
.
Consider the following Person
class code closely:
class Person {
#name = "Nathan";
getName() {
return this.#name;
}
setName(name) {
this.#name = name;
}
}
The name
variable above is declared using the hashtag #
symbol in front of it.
The hashtag is a symbol for private modifier in JavaScript classes, allowing you to create private properties and methods.
When you try to access the private field directly, a syntax error will be thrown by JavaScript:
let person = new Person();
person.name; // undefined
person.#name; // SyntaxError
person.getName(); // Nathan
To access the private property, you need to use the provided getName()
method. To change it, you need to use the provided setName()
method.
And that’s how you can implement encapsulation in JavaScript. Keep in mind the #
symbol for private properties and methods is a part of ECMAScript 2019 (ES10) specifications.
Currently, the feature is supported by all major browsers except Internet Explorer. Node.js supported the feature since version 12.
If you need to support older JavaScript versions, then you need to use the closure function
technique.
JavaScript encapsulation with closure functions
A closure function (also called inner function) is a function created inside another function.
The goal of a closure function is to enable access to private data defined in the outer function. In other words, to achieve encapsulation.
Before the release of JavaScript class
syntax, using a closure is the main method to implement encapsulation.
Here’s an example of a closure function from the createPerson()
function:
function createPerson() {
let name = "Nathan";
return {
getName: function () {
return name;
},
setName: function (newName) {
name = newName;
},
};
}
Both getName()
and setName()
functions are closure functions of the createPerson()
function.
Both functions have access to the name
variable defined outside of their scope, and you will get undefined
when you try to access the variable directly:
let person = createPerson();
person.getName(); // Nathan
person.name; // undefined
And that’s how you implement encapsulation using closures. You can create as many closure functions as you need on the return
statement of your JavaScript function.
Not only protecting data from outside access, you can also perform validation before changing the value inside your setter functions.
Now you’ve seen how to implement encapsulations with JavaScript. Great work! 👍