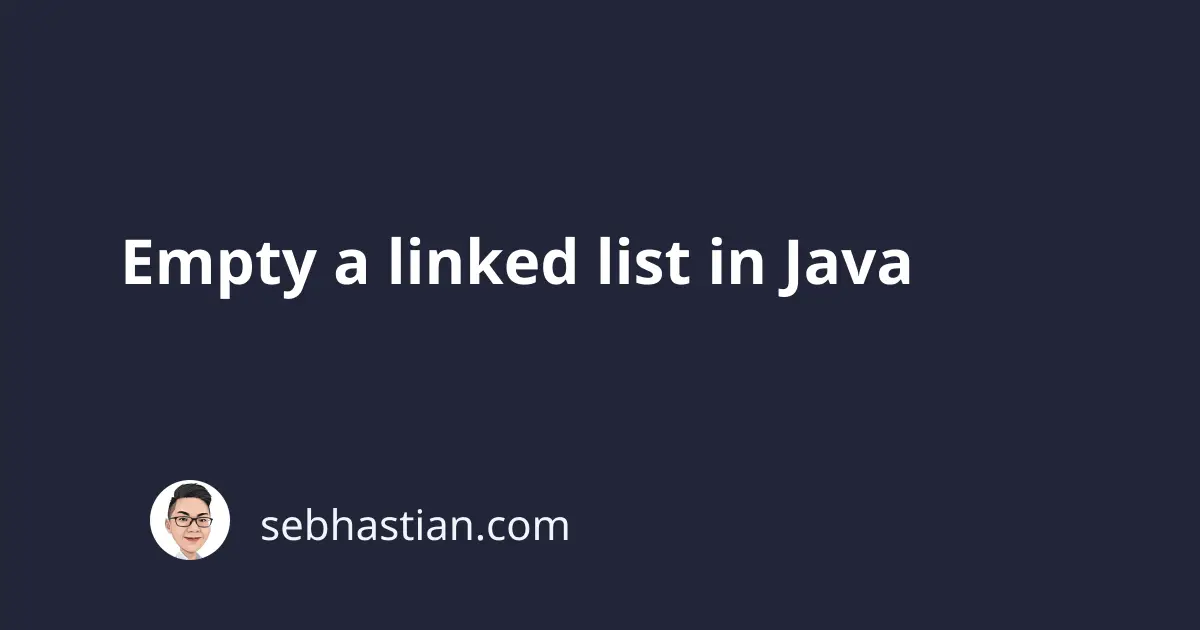
The Java LinkedList
class comes with the clear()
method that you can use to empty a linked list instance.
For example, suppose you initialize a LinkedList
with Integer
values as shown below:
LinkedList myList =
new LinkedList(Arrays.asList(1, 2, 3));
You can empty the myList
variable by calling the clear()
method like this:
System.out.println(myList);
myList.clear();
System.out.println(myList);
The output of the println()
call will be as follows:
[1, 2, 3]
[]
As you can see, the clear()
method empties the myList
instance, removing all values stored in the variable.
Alternatively, you can also create an empty LinkedList
instance during initialization as follows:
LinkedList emptyList = new LinkedList();
You can then add values to the emptyList
variable above by calling the add()
method:
emptyList.add(1);
emptyList.add(2);
emptyList.add(3);
System.out.println(emptyList); // [1, 2, 3]
Now you’ve learned how to empty a LinkedList
variable in Java. Good work! 👍