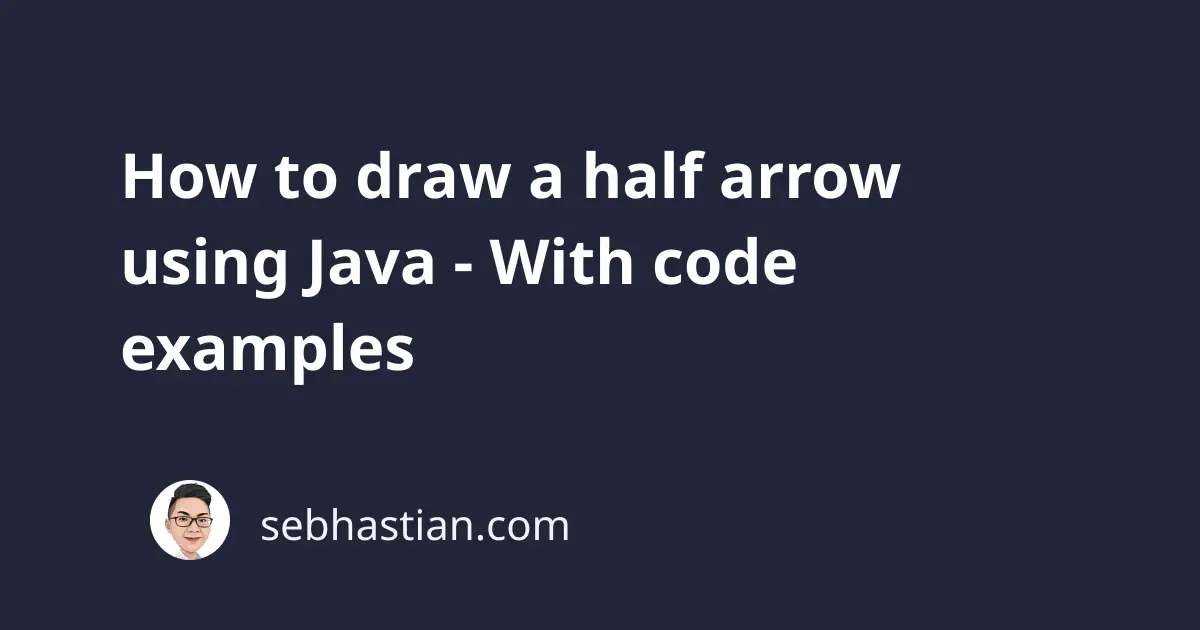
One of the most popular Java programming language exercises is to draw a half arrow using asterisks.
You are required to ask for the user input on the arrow’s base height, base width, and head width.
For example, suppose the user entered the following data to your Java program:
int arrowBaseHeight = 4;
int arrowBaseWidth = 3;
int arrowHeadWidth = 5;
The output of the program should be as follows:
*** // base width is 3
***
***
*** // 4 rows of arrow base height
***** // Head width starting at 5
****
***
**
*
This tutorial will help you learn how to solve the quiz above.
First, you need to use a loop in order to print the asterisk *
symbol according to the value of the variables.
Create a Java class named DrawHalfArrow
and define the following variables in the main()
method of that class:
class DrawHalfArrow {
public static void main(String[] args) {
int arrowBaseHeight = 4;
int arrowBaseWidth = 3;
int arrowHeadWidth = 5;
}
}
Next, create a loop that prints out the arrow base. Because you know the number of times you need to repeat the loop task, you can use the for
loop.
Here’s the code that will print the base of the half arrow:
for (int i = 0; i < arrowBaseHeight; i++) {
for (int j = 0; j < arrowBaseWidth; j++) {
System.out.print("*");
}
System.out.println();
}
The first for
loop will print the base height of the arrow.
For each row of the arrowBaseHeight
, the second for
loop will print out the asterisks as many times as the arrowBaseWidth
value.
Running the program will give you the following result:
***
***
***
***
As you can see, you get the 3
columns of arrow base width and 4
rows of arrow base height.
Next, you need to print the arrow head asterisks.
You can do this by using the same pattern of double for
loops just like with the base:
for (int k = arrowHeadWidth; k > 0; k--) {
for (int l = 0; l < k; l++){
System.out.print("*");
}
System.out.println();
}
First, you need a for
loop to print the number of rows for the head, which is going to be the same as the arrowHeadWidth
.
After that, you need a second for
loop that will print the asterisks. For each row, the asterisks will be decremented by one until there’s only one asterisk to print.
When you run the program, you’ll have the complete output:
***
***
***
***
*****
****
***
**
*
Now that you have the output correct, you need to make the program accept user inputs for the size of the arrow.
To do that, you need to use the Java Scanner
package, which gives you a command-line utility to accept user input.
Take a look at the code below:
import java.util.Scanner;
class DrawHalfArrow {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int arrowBaseHeight;
int arrowBaseWidth;
int arrowHeadWidth;
System.out.println("Enter arrow base height: ");
arrowBaseHeight = scanner.nextInt();
System.out.println("Enter arrow base width: ");
arrowBaseWidth = scanner.nextInt();
System.out.println("Enter arrow head width: ");
arrowHeadWidth = scanner.nextInt();
}
}
Instead of initializing the variables with arbitrary numbers, you let the user input the variable values with the scanner
object.
The nextInt()
method is used to let the scanner
know that you’re expecting an Int
value. If the value is not an Int
, then Java will throw an error and stop the program.
Finally, you need to make sure that the arrowHeadWidth
value is greater than the arrowBaseWidth
value.
This is because if you input an arrowHeadWidth
value that’s less than or equal to the arrowBaseWidth
value, then the arrow head will look wrong:
*** // arrowBaseWidth = 3
***
***
*** // arrowBaseHeight = 4
** // arrowHeadWidth = 2
*
The output above doesn’t look like an arrow, right?
To prevent the output above, you need to add a while
loop that’s going to run as long as the arrowHeadWidth
is less than or equal to the arrowBaseWidth
value:
while (arrowHeadWidth <= arrowBaseWidth) {
System.out.println("Arrow head width must be greater than base width!");
System.out.println("Enter arrow head width: ");
arrowHeadWidth = scanner.nextInt();
}
Here’s the complete code for the program:
import java.util.Scanner;
class DrawHalfArrow {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int arrowBaseHeight;
int arrowBaseWidth;
int arrowHeadWidth;
System.out.println("Enter arrow base height: ");
arrowBaseHeight = scanner.nextInt();
System.out.println("Enter arrow base width: ");
arrowBaseWidth = scanner.nextInt();
System.out.println("Enter arrow head width: ");
arrowHeadWidth = scanner.nextInt();
while (arrowHeadWidth <= arrowBaseWidth) {
System.out.println("Arrow head width must be greater than base width!");
System.out.println("Enter arrow head width: ");
arrowHeadWidth = scanner.nextInt();
}
for (int i = 0; i < arrowBaseHeight; i++) {
for (int j = 0; j < arrowBaseWidth; j++) {
System.out.print("*");
}
System.out.println();
}
for (int k = arrowHeadWidth; k > 0; k--) {
for (int l = 0; l < k; l++){
System.out.print("*");
}
System.out.println();
}
}
}
When you run the program, you will have an output as shown below:
Enter arrow base height:
5
Enter arrow base width:
4
Enter arrow head width:
4
Arrow head width must be greater than the arrow base width!
Enter arrow head width:
5
****
****
****
****
****
*****
****
***
**
*
With that, you have solved the Java exercise of writing a program that draws a half arrow. Good work!
Feel free to use the code in this tutorial for your projects. 😉