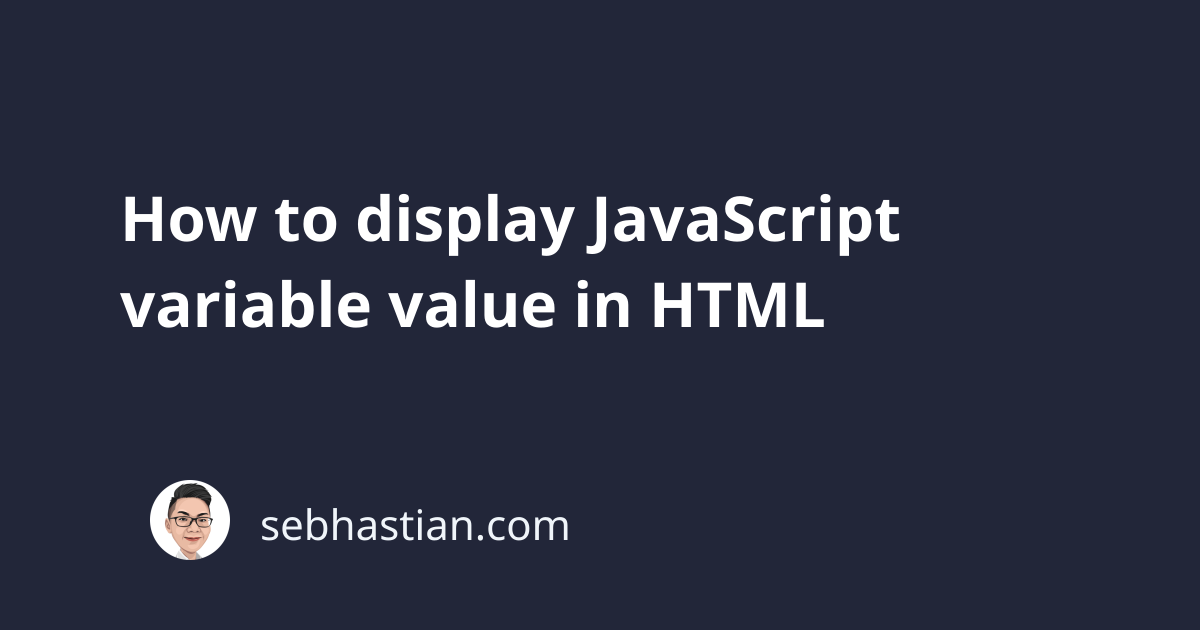
There are three ways to display JavaScript variable values in HTML pages:
- Display the variable using
document.write()
method - Display the variable to an HTML element content using
innerHTML
property - Display the variable using the
window.alert()
method
This tutorial will show you how to use all three ways to display JavaScript variables in HTML pages. Let’s start with using document.write()
method.
Display JavaScript variable using document.write()
method
The document.write()
method allows you to replace the entire content of HTML <body>
tag with HTML and JavaScript expressions that you want to be displayed inside the <body>
tag. Suppose you have the following HTML element:
<body>
<h1>Hello World</h1>
<p>Greetings</p>
</body>
When you run document.write("Hello")
method on the HTML piece above, the content of <body>
will be replaced as follows:
<body>
Hello
</body>
Knowing this, you can display any JavaScript variable value by simply passing the variable name as the parameter to document.write()
method:
let name = "Nathan";
document.write(name); // Writes Nathan to the <body> tag
let num = 999;
document.write(num); // Writes 999 to the <body> tag
The document.write()
method is commonly used only for testing purposes because it will delete any existing HTML elements inside your <body>
tag. Mostly, you would want to display a JavaScript variable beside your HTML elements. To do that, you need to use the next method.
Display JavaScript variable using innerHTML property.
Every single HTML element has the innerHTML
property which holds the content of that element. The browser allows you to manipulate the innerHTML
property by using JavaScript by simply assigning the property to a different value.
For example, imagine you have the following HTML <body>
tag:
<body>
<h1 id="header">Hello World</h1>
<p id="greeting">Greetings</p>
</body>
You can replace the content of <p>
tag by first retrieving the element using its identifier. Since the element <p>
has an id
attribute with the value of greeting
, you can use document.getElementById
method to retrieve it and change its innerHTML
property.
Here’s how you do it:
document.getElementById("greeting").innerHTML = "Bonjour";
The content of <p>
tag will be changed as follows:
<body>
<h1 id="header">Hello World</h1>
<p id="greeting">Bonjour</p>
</body>
Knowing this, you can simply wrap the space where you want your JavaScript variable to be displayed with a <span>
element as follows:
<body>
<h1>Hello, my name is <span id="name"></span></h1>
<script>
let name = "Nathan";
document.getElementById("name").innerHTML = name;
</script>
</body>
The code above will output the following HTML:
<h1>Hello, my name is <span id="name">Nathan</span></h1>
And that’s how you can display JavaScript variable values using innerHTML
property.
Display JavaScript variable using window.alert() method
The window.alert()
method allows you to launch a dialog box at the front of your HTML page. For example, when you try running the following HTML page:
<body>
<h1>Hello World</h1>
<script>
window.alert("Greetings");
</script>
</body>
The following dialog box should appear in your browser:
The implementation for each browser will slightly vary, but they all work the same. Knowing this, you can easily use the dialog box to display the value of a JavaScript variable. Simply pass the variable name to the alert()
method as follows:
<body>
<h1>Hello World</h1>
<script>
let name = "Nathan JS"
window.alert(name);
</script>
</body>
The code above will launch a dialog box that displays the value of the name
variable.
Conclusion
Displaying JavaScript variables in HTML pages is a common task for web developers. Modern browsers allow you to manipulate the HTML content by calling on exposed JavaScript API methods.
The most common way to display the value of a JavaScript variable is by manipulating the innerHTML
property value, but when testing your variables, you can also use either document.write()
or window.alert()
methods. You are free to use the method that suits you best.