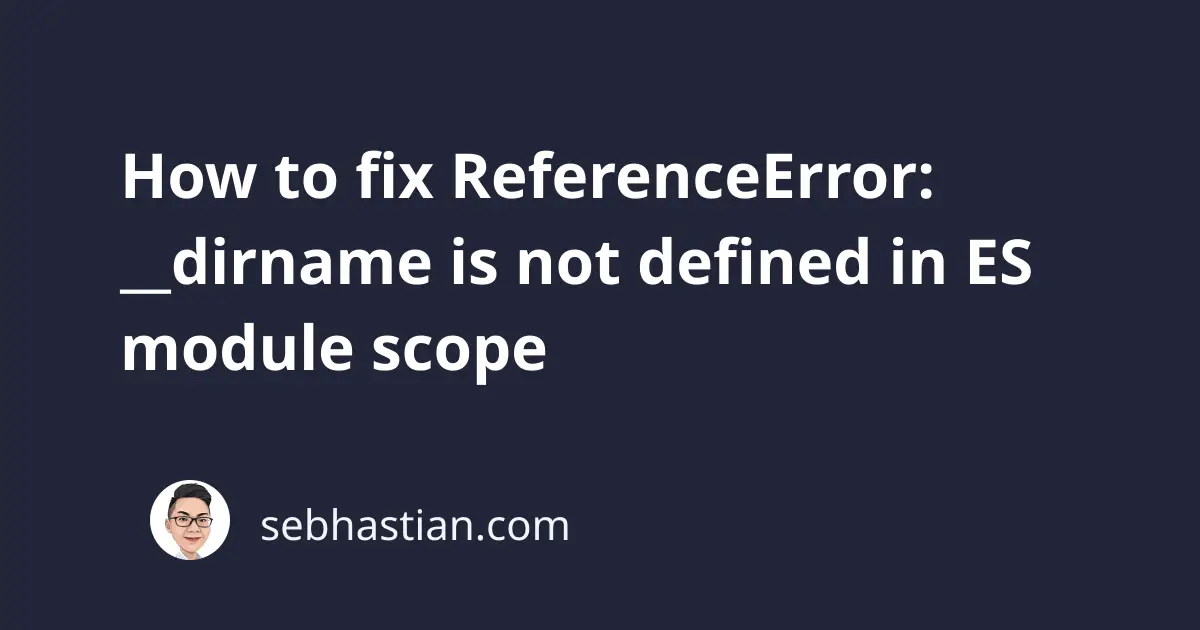
When running JavaScript code in ES modules system, you might get the following error:
ReferenceError: __dirname is not defined in ES module scope
The __dirname
variable is a special variable containing the path to the current module’s directory.
This error occurs because the __dirname
variable only exists in CommonJS modules and isn’t available under ES modules system.
This tutorial will show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you try to get the path to the current module’s directory by calling the console.log()
function on the __dirname
variable:
console.log(__dirname)
But in your package.json
file, the type
property is set to module
as follows:
{
"type": "module"
}
When you run the code with Node.js, the following error appears:
console.log(__dirname)
ReferenceError: __dirname is not defined in ES module scope
This error occurs because under the ES module, there’s no __dirname
variable.
How to fix this error
To resolve this error, you need to generate the __dirname
variable yourself by using the Node.js path
and url
module.
First, import the modules required to create the __dirname
variable:
import { fileURLToPath } from 'url'
import path from 'path'
Next, use the fileURLToPath()
to generate the path to the file you’re currently running.
Once you get the path to the file, create the path to the directory using the path.dirname()
method as follows:
const __filename = fileURLToPath(import.meta.url)
const __dirname = path.dirname(__filename)
The import.meta.url
is used to get the URL of the file location, which uses the file://
protocol. The fileURLToPath()
function returns the absolute path without the protocol.
The path.dirname()
method then remove the file name from the path so that you get the directory name.
If you’re using the __dirname
value frequently, you can create a helper function to get the value for you.
For example, you can create a helper.js
module under a libs/
directory. Inside the module, define a function named getDirName()
with the following contents:
import path from 'path'
import { fileURLToPath } from 'url'
const getDirName = function (moduleUrl) {
const filename = fileURLToPath(moduleUrl)
return path.dirname(filename)
}
export {
getDirName
}
Now whenever you need to get the __dirname
value, you just import the function and pass the import.meta.url
property as an argument to the function:
import { getDirName } from './libs/helper.js'
const __dirname = getDirName(import.meta.url)
console.log(__dirname) // ✅
Now the __dirname
constant will store the path to the current module’s directory, and you no longer receive the error. Nice work!
Conclusion
The ReferenceError: __dirname is not defined in ES module scope
occurs when you run JavaScript using Node.js under the ES modules system.
The variable’s value is only generated in the CommonJS modules system, so you need to manually get the value using path
and url
modules when under the ES modules system.
I hope this tutorial is useful. Happy coding! 🙌