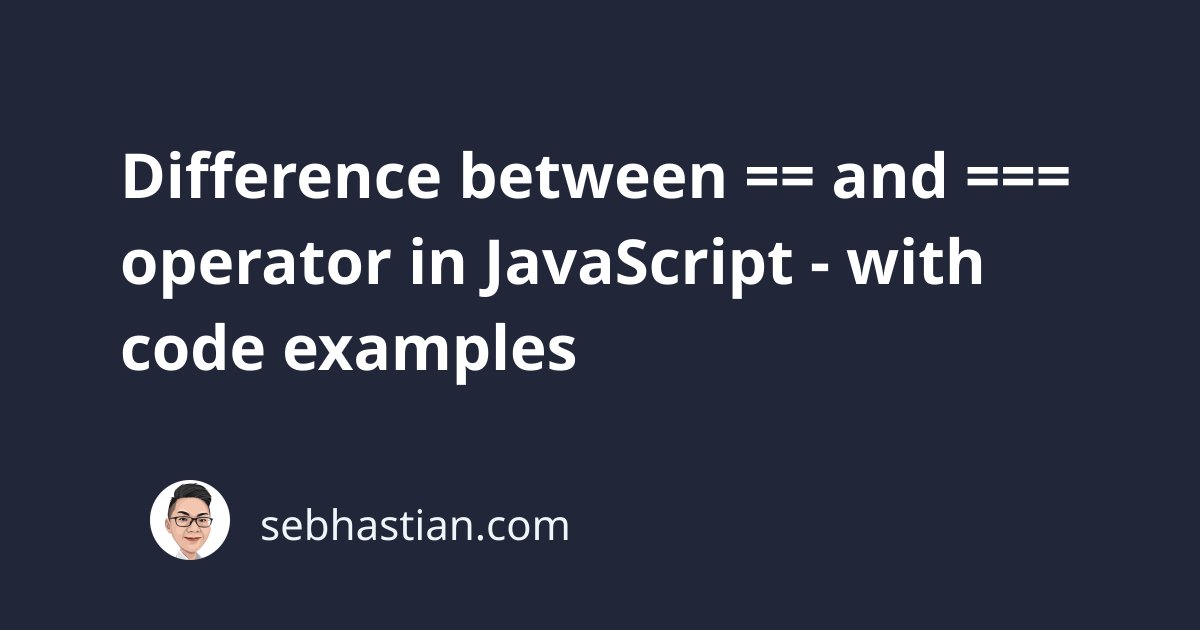
Both double equals ==
and triple equals ===
operator is used for comparing between two values on which the operator is used on.
The difference between the two operators is that the double equals ==
will compare the values loosely, meaning that it will try to convert values with different types before comparing them.
The triple equals ===
won’t convert values of different types. It will simply return false
when comparing values of different types.
To understand their differences, let’s try comparing two different values between the number
value 0
and boolean
value false
:
console.log(0 == false); // true
console.log(0 === false); // false
As you can see from the code above, the ==
operator returns true because the boolean
value false
is converted to a number
before comparing it with 0
:
console.log(Number(false)); // 0
This kind of conversion is done implicitly, meaning that when you store the values in variables and compare them, the variable values will be converted only during the comparison.
In the following example, the value of variable bool
after the comparison will remain false
and not 0
:
let num = 0;
let bool = false;
console.log(num == bool); // true
console.log(bool); // false not 0
When comparing values of different types, the implicit conversion rules are as follows:
- If one of the values is a
boolean
type, theboolean
value will be converted tonumber
value, withtrue
converted to1
andfalse
converted to0
- When comparing between
string
andnumber
type values, convert thestring
value to numeric value. - If one of the values is an
object
type (including arrays) then theobject
will be converted tostring
first
The following example shows the double equals ==
conversion rules in action:
console.log(1 == true); // 1 == 1 = true
console.log(5 == "5"); // 5 == 5 = true
console.log([1,2] == "1,2"); // "1,2" == "1,2" = true
console.log(23 == true); // 23 == 1 = false
On the other hand, the triple equals ===
will simply return false
for the same values as above because it doesn’t do conversion at all:
console.log(1 === true); // false
console.log(5 === "5"); // false
console.log([1,2] === "1,2"); // false
console.log(23 === true); // false
Any comparison between different types with ===
will always return false
.
Conclusion
To conclude, the differences between ==
and ===
operator is that the ==
operator will try to convert values of different types before comparing them, while the ===
operator always just compares them and return false
for values of different types.
The ==
operator is also known as double equals operator or loose equality operator.
The ===
operator is also known as triple equals operator or strict equality operator.