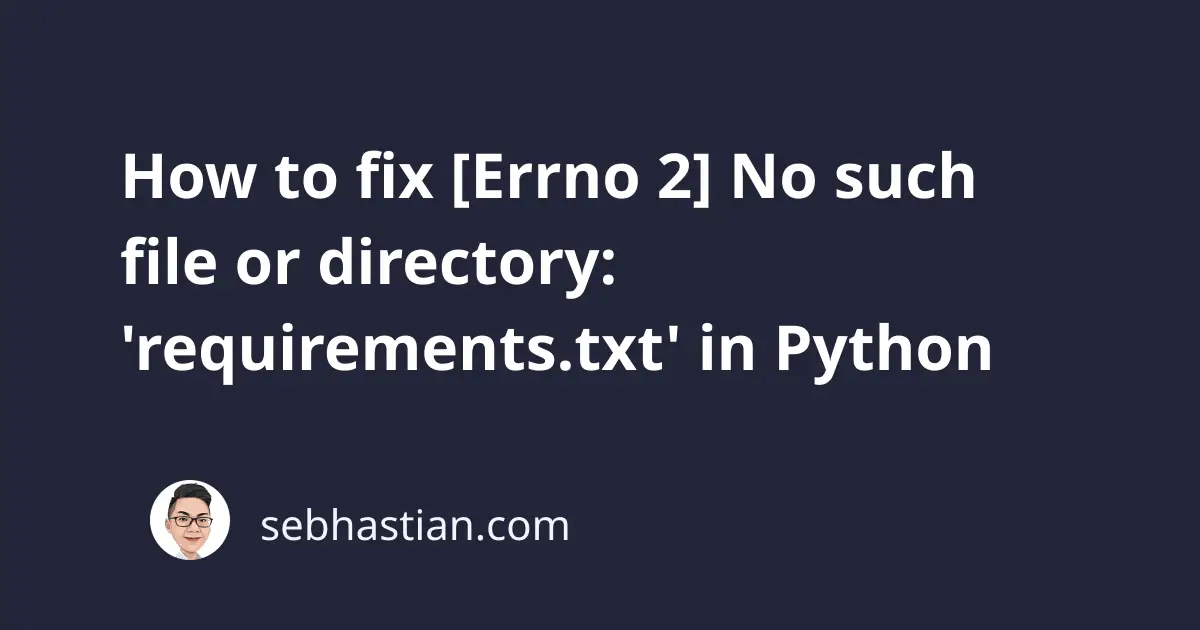
When trying to install Python dependencies using pip
and requirements.txt
file, you might encounter the following error:
ERROR: Could not open requirements file:
[Errno 2] No such file or directory: 'requirements.txt'
This error occurs when you run the pip install -r requirements.txt
command but the requirements.txt
file is not available in the current directory.
There are three possible methods you can use to resolve this error:
- Generate a requirements.txt file using
pip freeze
command - Generate and run
requirements.txt
file from a Docker instance - Find the location of
requirements.txt
and use the correct path when installing
I will show you how to fix this error in practice using the methods above.
1. Generate the requirements.txt file
You can resolve this error by generating a requirements.txt
file with the pip freeze
command:
pip freeze > requirements.txt
# For pip3 users:
pip3 freeze > requirements.txt
The freeze
command will generate a requirements.txt
file based on the dependencies you’ve installed using pip.
Once finished, you should be able to run the pip install command again without receiving this error.
2. How to generate and run requirements.txt from Docker
If you’re using Docker to run your Python code, then you can generate and install the requirements.txt
file by writing this command in your Dockerfile:
RUN pip freeze > requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
The first command uses pip freeze
to generate the text file, and the second command will install packages defined in the file.
3. How to find your requirements.txt file
If you think you already have the requirements.txt file somewhere in your project, then you can use the find
command to get the location of your requirements.txt
file.
From the root directory of your project, run the following command:
find . -regex '.*requirements.txt$'
You should see a response as follows:
./src/requirements.txt
You can then use this location when running the pip install command as shown below:
pip install -r ./src/requirements.txt
# or pip3:
pip3 install -r ./src/requirements.txt
pip can be used to install from a different path, but you need to provide the relative path from the current directory as shown below:
pip3 install -r ./path/to/file
If you already found the requirements.txt
file, you can also move that file to the root directory for easy access.
Conclusion
Python raises [Errno 2] No such file or directory: 'requirements.txt'
when it can’t find the requirements.txt
file needed by pip.
To resolve this error, you can generate a requirements.txt
file by running the pip freeze > requirements.txt
command.
You can also use the find
command to find the location of your requirements.txt file and use it in your pip install
command.
I hope this tutorial helps. See you in other tutorials! 👍