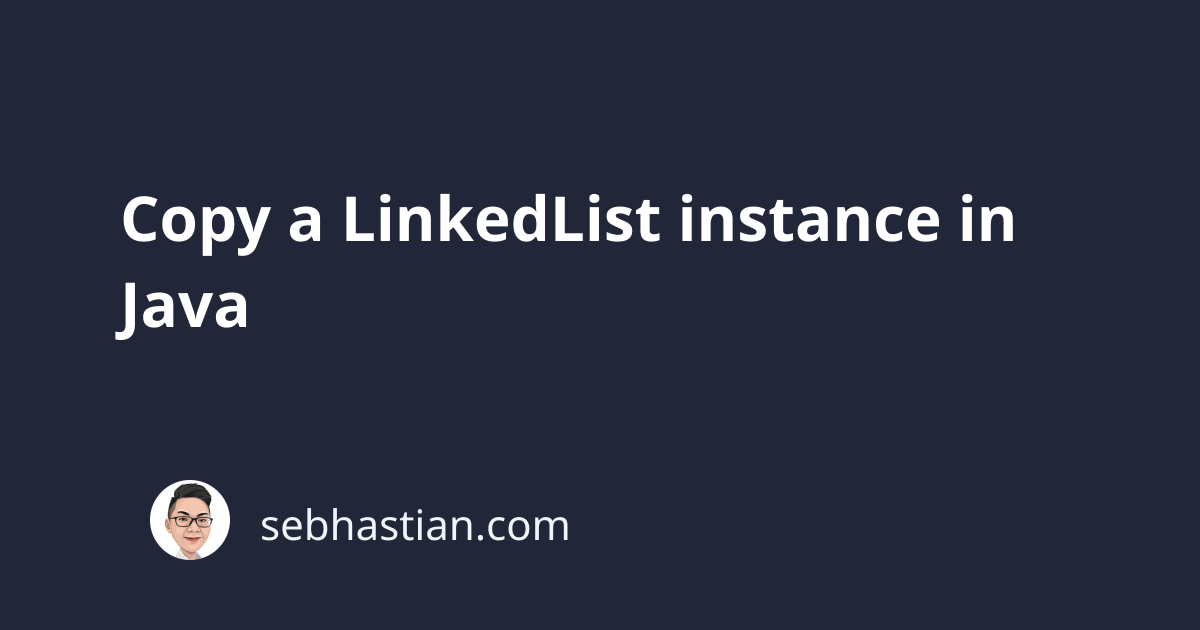
Java allows you to create a copy of a LinkedList
instance by simply calling the clone()
method from the instance.
For example, suppose you already have a LinkedList
instance named myList
as shown below:
LinkedList myList =
new LinkedList(Arrays.asList("Nathan", "Sebhastian"));
To create a copy of the myList
variable above, call the clone()
method and assign the result into a new variable like this:
LinkedList copyList = (LinkedList) myList.clone();
System.out.println(copyList);
The copyList
variable will have the same values as the myList
variable:
[Nathan, Sebhastian]
The clone()
method of the LinkedList
class creates a shallow copy of the LinkedList
instance where you call the method.
Alternatively, you can create a shallow copy of the instance by calling the LinkedList()
constructor and passing the instance as an argument:
LinkedList myList =
new LinkedList(Arrays.asList("Nathan", "Sebhastian"));
LinkedList copy = new LinkedList(myList);
System.out.println(copy);
The output of the println()
call will be as follows:
[Nathan, Sebhastian]
Both the clone()
method and the LinkedList(Collection c)
constructor return a shallow copy of the existing LinkedList
instance for you to use.
And that’s how you create a LinkedList
instance copy in Java. 😉