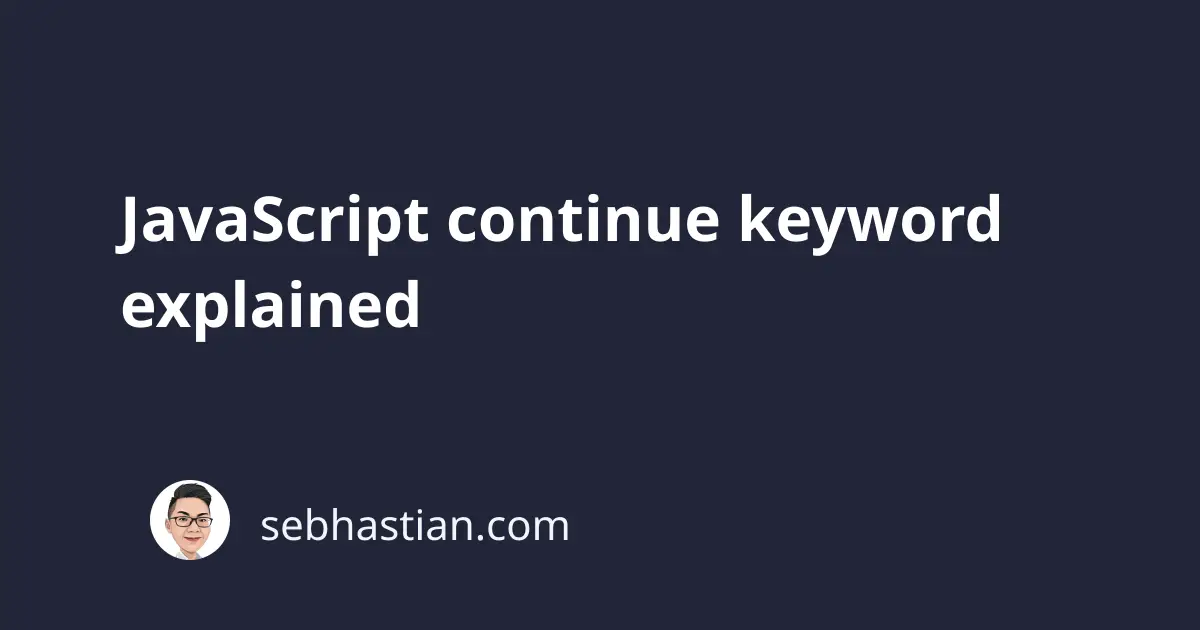
The continue
keyword in JavaScript is used to skip an iteration once. It’s commonly used inside a for
or while
loop when a specific condition is met.
For example, the following code will skip the iteration once when the value of i
variable is 2
:
for (let i = 0; i <= 5; i++) {
if (i === 2) {
console.log("continue");
continue;
}
console.log(`The current number is: ${i}`);
}
The output from the code above will be as shown below:
The current number is: 0
The current number is: 1
continue
The current number is: 3
The current number is: 4
The current number is: 5
The number 2
is not logged to the console because it got skipped with continue
.
When using the continue
keyword with a while
loop, you need to make sure that the variable you used to count the loop is incremented before the continue
statement is executed. The following code example will cause infinite loop because the variable i
won’t be incremented anymore once the value is equal to 2
:
let i = 0;
while (i <= 5) {
if (i === 2) {
continue;
}
console.log(`The current number is: ${i}`);
i += 1;
}
You need to move the incrementing expression i += 1
above the if
condition:
let i = 0;
while (i <= 5) {
i += 1;
if (i === 2) {
continue;
}
console.log(`The current number is: ${i}`);
}
This way, the counting variable will still be incremented after the continue
statement has been executed.