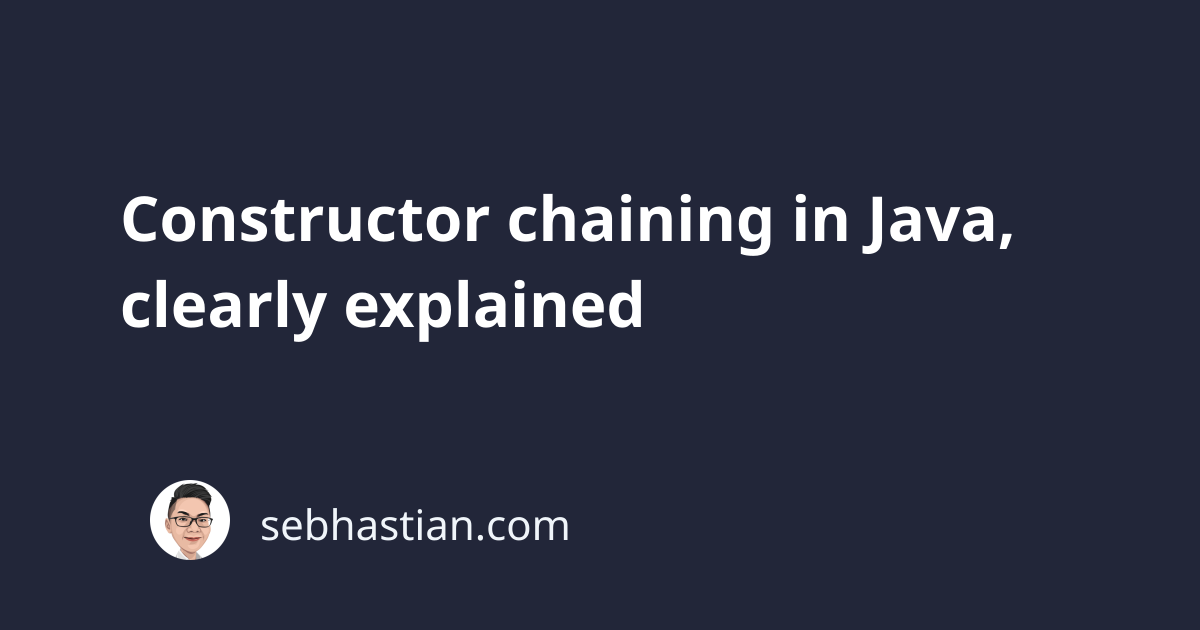
In Java, constructor chaining is the process of calling a constructor from another constructor within the same context object.
Constructor chaining is useful to avoid repetition in your code while providing multiple constructors used in different scenarios.
A constructor chaining can be done in two ways:
- You can call other constructors from the same class using
this()
function - You can call the super or base class constructor using the
super()
keyword
Let’s see an example of constructor chaining in action.
Suppose you have a Product
class with the following definitions:
class Product {
String name;
int price;
// constructor 1
Product() {
this.name = "Car";
this.price = 0;
}
// constructor 2
Product(String name) {
this.name = name;
this.price = 0;
}
// constructor 3
Product(String name, int price) {
this.name = name;
this.price = price;
}
}
There are 3 constructors defined in the Product
class above that give you flexibility in initializing a new Product
object.
All 3 constructors can be used separately as follows:
Product prod = new Product(); // Car, 0
Product prod2 = new Product("Phone"); // Phone, 0
Product prod3 = new Product("Phone", 55); // Phone, 55
But you can actually achieve the same result using constructor chaining in your Product
class.
Take a look at the following example:
class Product {
String name;
int price;
Product() {
this("Car");
}
Product(String name) {
this(name, 0);
}
Product(String name, int price) {
this.name = name;
this.price = price;
}
}
In the new Product
class above, the first two constructors of the class call the other constructor to initialize the class members.
This updated class produces the same result as the former class, but with less code written inside the constructors.
Constructor chaining with super()
Aside from avoiding redundant code, constructor chaining is also used by a subclass to call the constructor of the superclass.
For example, suppose you have a Baby
class that is a subclass of the Human
class as shown below:
class Baby extends Human {
Baby(String name) {
this.name = name;
}
}
class Human {
String name;
int fingers;
Human() {
this.fingers = 5;
}
}
During the construction of the Human
class, the fingers
property is assigned the value of 5
.
But when you create a new Baby
object, this is the output of the fingers
property:
Baby lisa = new Baby("Lisa");
System.out.println(lisa.fingers); // 5
How come lisa.fingers
return 5
when the Baby
class constructor only assigns the value of the name
property?
This is because the superclass constructor is automatically called by Java before running the subclass constructor.
The above Baby
class is executed as follows:
class Baby extends Human {
Baby(String name) {
super();
this.name = name;
}
}
But keep in mind that only the default constructor without any parameters super()
is called by the subclass.
If you have a super constructor with one parameter or more, you need to call it explicitly from the subclass.
Suppose the Human
class has a second constructor adding a superpower
as shown below:
class Human {
String name;
int fingers;
String superpower;
Human(String superpower){
this.fingers = 5;
this.superpower = superpower;
}
}
Without the default Human()
constructor, the Baby()
constructor line will throw an error saying There is no default constructor in Human
.
You need to explicitly call super()
as shown below:
class Baby extends Human {
Baby(String name) {
super("Breathe underwater");
this.name = name;
}
}
The super()
call above should resolve the no default constructor error.
The rules of constructor chaining
Java has some rules regarding constructor chaining as follows:
- Constructor call with
this()
orsuper()
keyword must be the first line in any constructor that calls another constructor. - Each
class
requires at least one constructor that doesn’t call another constructor. This is to avoid an infinite loop of constructor calls.
There’s no rule regarding the order of the constructor chaining, but a common rule of thumb is that constructors with fewer parameters should call constructors with more parameters.
Constructor chaining is one of the features frequently used in Java packages because of its ability to make flexible classes.
Now you’ve learned how constructor chaining works in Java.
You’ve also seen a practical example where constructor chaining has reduced code redundancy and called the constructor of the superclass.
I hope this tutorial has been useful for you. 🙏