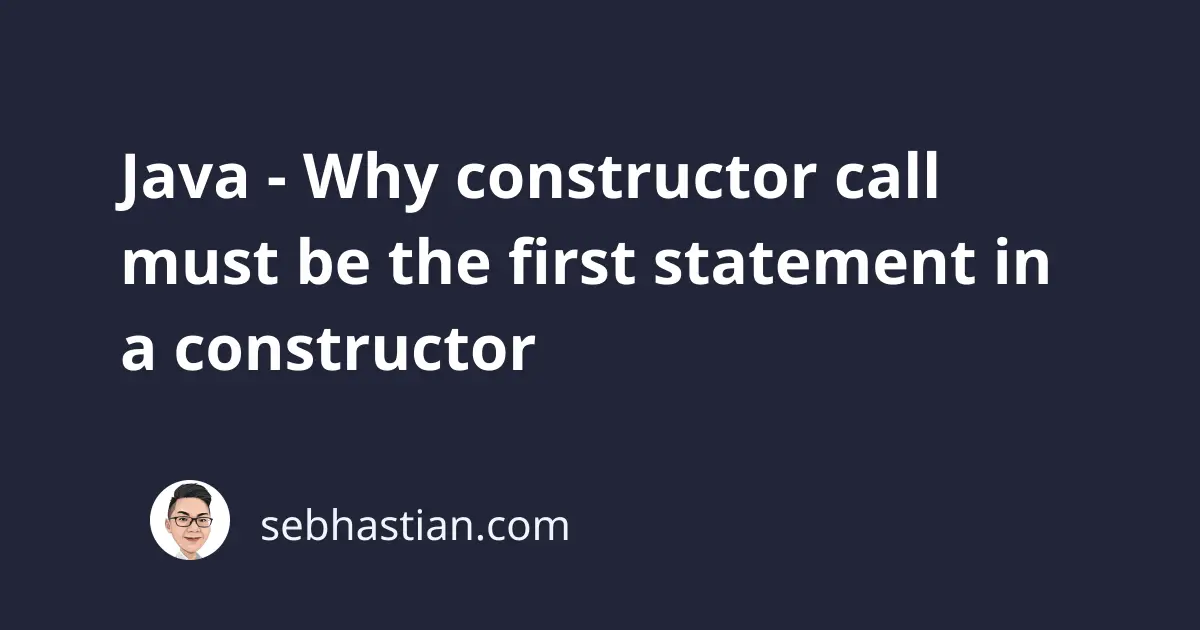
When you define a Java class
constructor that calls another constructor, you need to place the constructor call at the top of the constructor definition.
For example, the following Product
class has two constructors, with the first constructor calling the second:
class Product {
public String name;
public Product() {
this("Mouse");
this.name = "Keyboard";
}
public Product(String name) {
this.name = name;
}
}
When you move the constructor call below the this.name
assignment, Java will throw the call to this must be first statement in constructor
:
class Product {
public String name;
public Product() {
this.name = "Keyboard";
this("Mouse"); // ERROR
}
public Product(String name) {
this.name = name;
}
}
This is because when you run other statements before the call to the constructor, then you might call some methods or states that are not yet defined.
The same happens when you extend a superclass. You need to call the super()
constructor before anything else.
When other statements before the super()
constructor are allowed, then you might try to call or manipulate class members and methods that are not yet constructed.
It’s a rule set up by Java itself to prevent errors by disabling dynamic orders between the initialization of the class object and other assignments and operations.
So when you need to perform a constructor chaining or call the superclass constructor, make sure that you do it on the first line.