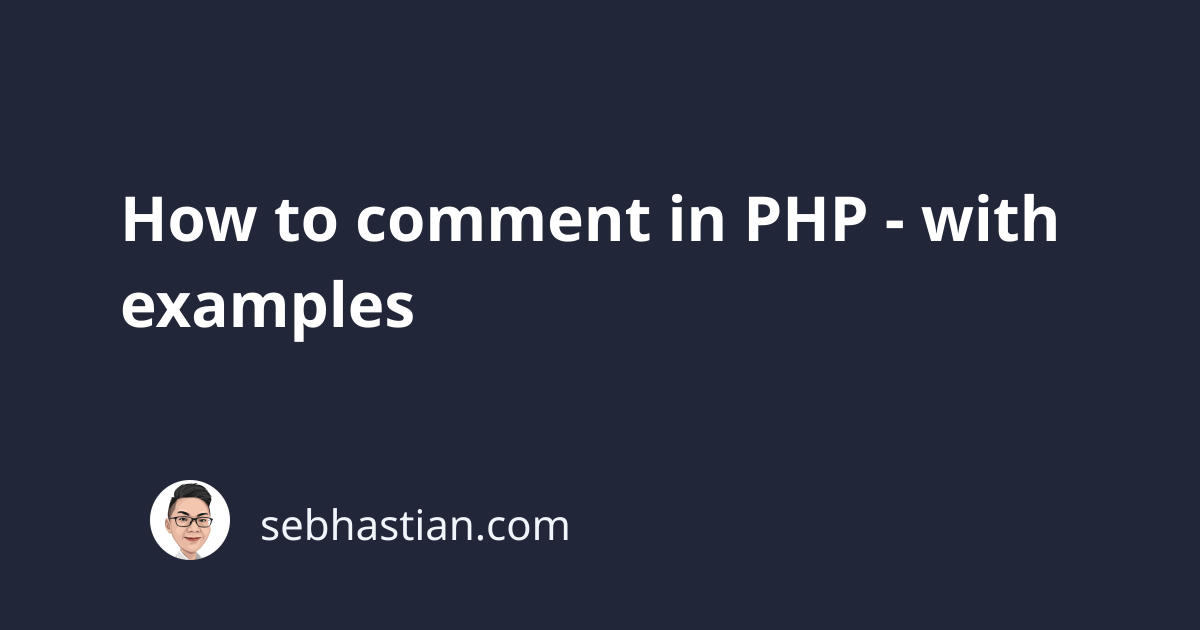
In programming, comments are written descriptions that are not executed as part of the program.
The purpose of a comment is to help fellow humans understand the purpose of the written code.
Comments are useful because you can:
- Communicate with others about the intention of your code
- Document parts of your code. Remind yourself the purpose of that code block
- Temporarily turn off parts of your code in debugging process
Some may argue that without comments, developers and programmers should be able to figure out the intention of the code by looking at it.
While this is true, figuring out the code you didn’t write yourself may get an ambiguous meaning.
By adding comments, you will remove all doubts about the purpose of the code, allowing others to work more effectively.
Commenting is also used to remove parts of your code from execution. This allows you to debug your code without having to delete that code from the file.
There are two different ways to write comments in PHP:
- A single-line comment
- Multi-line comments
Let’s see how you can do both in this guide.
Create PHP single-line comment
To create a single-line comment in PHP, you need to add the double forward slash (//
) or hash (#
) symbol to your code.
Consider the example below:
<?php
echo "Hello World!";
// This is a comment
# This is also a comment
The comment symbol will only mark all text to the right of the symbol.
You can add code on the left side of the comment:
<?php
echo "Hello World!"; // Right-side comment
echo "Good morning"; # Also a right-side comment
You can comment a code to exclude it from running like this:
// echo "Hello World!"; // Right-side comment
echo "Good morning";
The first echo
function above is commented, so Hello World!
will not be printed the next time PHP compiler process the code.
And that’s how you create a single-line comment. Let’s see how to create a multi-line comment next.
PHP multi-line comments
To create a multi-line comment in PHP, you need to:
- Open the comment using the forward slash and asterisk symbol (
/*
) - Close the comment with the asterisk and forward slash symbol (
*/
)
Here’s an example of creating multi-line comments:
<?php
/* This is a multi-line PHP comment
The comment will continue
as long as you do not close it. */
echo "Hello World!";
?>
There are three lines of comments in the example above.
PHP also has an extension called PHPDoc that increases the usefulness of a comment.
For example, you can describe the parameters used in a function using the @param
tag like this:
<?php
/**
* Print greetings.
*
* @param string $name The name to greet.
* @param string $greeting The greeting to print
* Defaults to `Hello`.
*/
function greetings(string $name, string $greeting = "Hello")
{
echo "$greeting, $name!";
}
The PHPDoc format as shown above will be picked up by modern PHP IDE, allowing you to see the code source when you hover over the calling syntax:
As you can see, comments are very useful to verify the purpose of your code.
Verbose, detailed descriptions using the PHPDoc format also allow PHP IDEs to provide auto-completion and code hints.
When you work on long-term projects, adding comments will speed up your progress and help others to work more effectively.