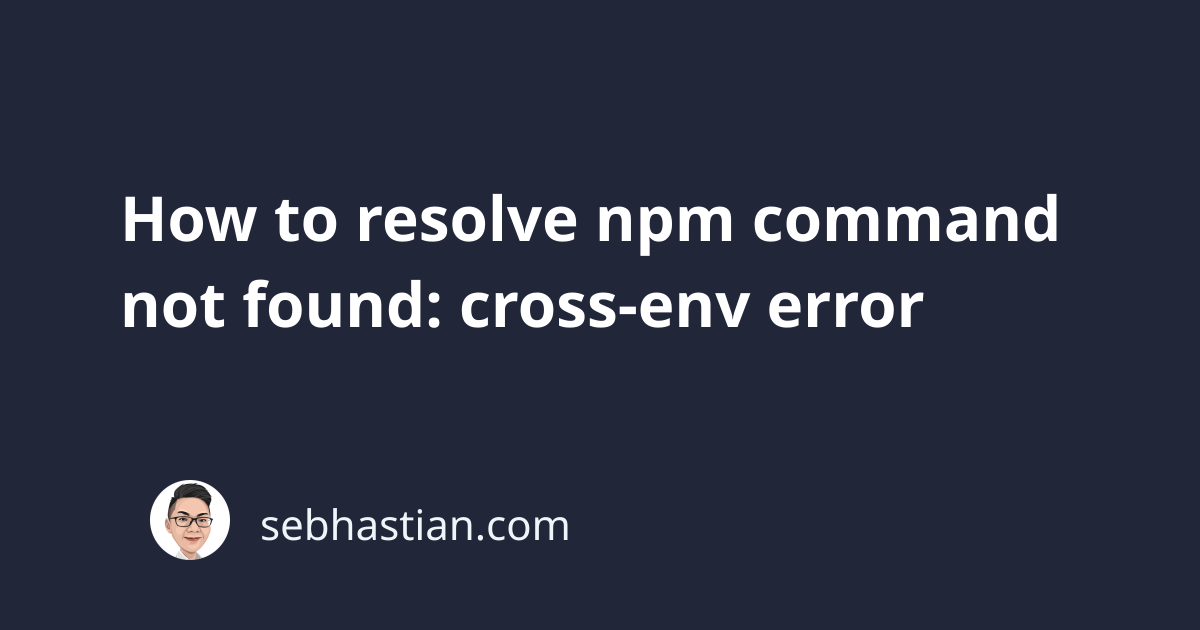
The command not found: cross-env
error happens when your Node server can’t find the cross-env
package required by the npm command you just run.
The cross-env
package is an npm package used for setting the environment variable.
Usually, the cross-env
package is added to the scripts
object in your package.json
file like this:
{
"scripts": {
"dev": "cross-env NODE_ENV=development webpack",
"watch": "cross-env NODE_ENV=development webpack",
"hot": "cross-env NODE_ENV=development webpack-dev-server",
"production": "cross-env NODE_ENV=production webpack"
},
}
When you run one of the commands above using npm, then npm will look for the cross-env
variable to set the NODE_ENV
variable as defined in the above script.
When you don’t have the cross-env
package installed under your node_modules/
folder, then the terminal will respond with the cross-env: command not found
error:
$ npm run dev
> [email protected] dev
> cross-env NODE_ENV=development webpack
sh: cross-env: command not found
To resolve this error, you need to make sure that the cross-env
package is available in your node_modules/
folder.
Open the terminal in your project directory, then use npm install
command to install the package like this:
npm install cross-env
You should be able to run the npm commands successfully now.
If that doesn’t work, make sure that you are installing the package locally and not globally. Don’t add the -g
or --global
flag with your npm install
command:
# 👇 will not work
npm install -g cross-env
Run the npm list
command from your project directory. You should see cross-env
listed in the output as follows:
$ npm list
[email protected] /Users/nsebhastian/Desktop/DEV/n-app
├── [email protected]
├── [email protected]
└── [email protected]
If you don’t see the cross-env
package, then it’s possible that the installation of cross-env
is interrupted.
You may have an outdated npm version, so check your npm version with the npm -v
command.
At the time of this writing, the latest npm version is 8.12.1,
You can check the latest version of npm
available from its npmjs page.
You can install the latest npm version with this command:
npm install -g npm@latest
Once you have the latest version of npm, try install cross-env
again. You should see the error fixed.
Why not use npx?
If the cross-env
command is included in your scripts
object, that means that the package is required for running the project.
When a package is required for the project, it’s recommended to include it as a dependency.
The npx
command is created to run a package infrequently, like a one-time setup using create-react-app
for instance.
When you use npx, then you need to copy the whole script command to the console like this:
# 👇 Run cross-env with npx
npx cross-env NODE_ENV=development webpack
With npx, you can’t do npx run dev
or npx run production
.
You also can’t import the cross-env
package when you need it in your code later:
// 👇 Error: Cannot find module 'cross-env'
var env = require('cross-env');
Finally, npx will get the latest version of the package, so you might see compatibility issues when the package introduces a major update.
With npm, a package-lock.json
file will be generated to store the exact version of the package installed.
That’s why you are recommended to install the package using npm.
I hope this tutorial has been useful for you. 🙏