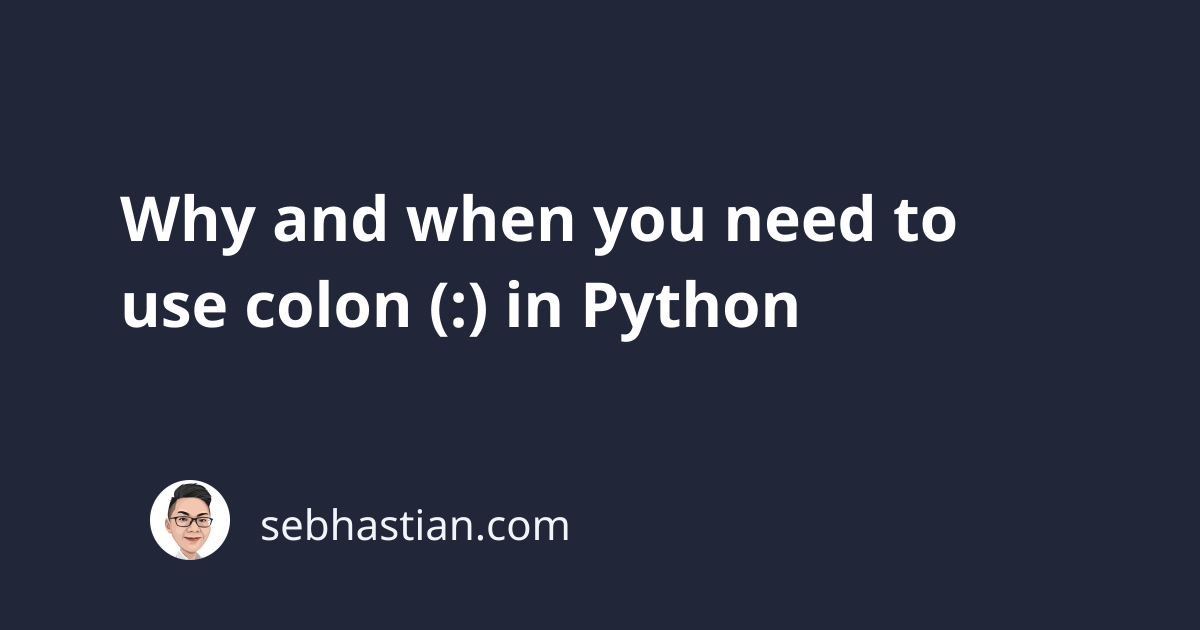
In Python, the colon :
symbol is used in many situations:
- When you need to create an indented block
- To slice a list or a string
- Create a
key:value
pair in a dictionary object
This tutorial will show you when to use a colon in practice.
1. Creating an indented block
The colon symbol is commonly used in Python to create an indented block.
In Python, indents have a special meaning because they indicate that the code written in that line is a part of something.
That something can be a class, a function, a conditional, or a loop.
For example, you used the colon symbol when defining a class as follows:
class Car:
pass
# class body here
If you only indent the lines below the class Car
line, Python will respond with an syntax error expecting a colon.
The colon here works as the indicator marking the start of the class body.
The same also applies for functions, conditionals, and loops:
# A function requires parentheses and a colon
def my_function():
pass
# A conditonal requires a colon after the condition to evaluate
if True:
pass
# A loop requires a colon after the loop
for i in range[5]:
pass
The code above shows when you need to use a colon to start an indented block.
2. To slice a list or a string
Colons are also used to slice a list or a string in Python.
The slicing syntax is as follows:
string[start:end:step]
The three numbers marking the start
, end
, and step
in a slice is separated using colons.
When you omit the other two parameters, you can use a double colon ::
as follows:
my_str = "abcdefg"
print(my_str[::2]) # aceg
print(my_str[3::]) # defg
print(my_str[:5:]) # abcde
Here, the colons are used to slice a string. You can also do the same with a list.
3. Create a key:value pair in a dictionary object
Another use of the colon symbol is to create a key:value
pair in a dictionary object.
You use the curly brackets to start a dictionary object, then create the key:value pair using colons like this:
my_dict = {
'name': 'Nathan',
'age': 29,
'hobby': 'programming'
}
When you have more than one key:value pair, you need to separate them using a comma.
Conclusion
In other programming languages, you would see curly brackets used as the indicator of an indented block.
In Python, a colon is used to create an indented block, as well as for slicing a list or a string, and creating a key:value pair in a dictionary.
I hope this tutorial helps. See you in other tutorials!