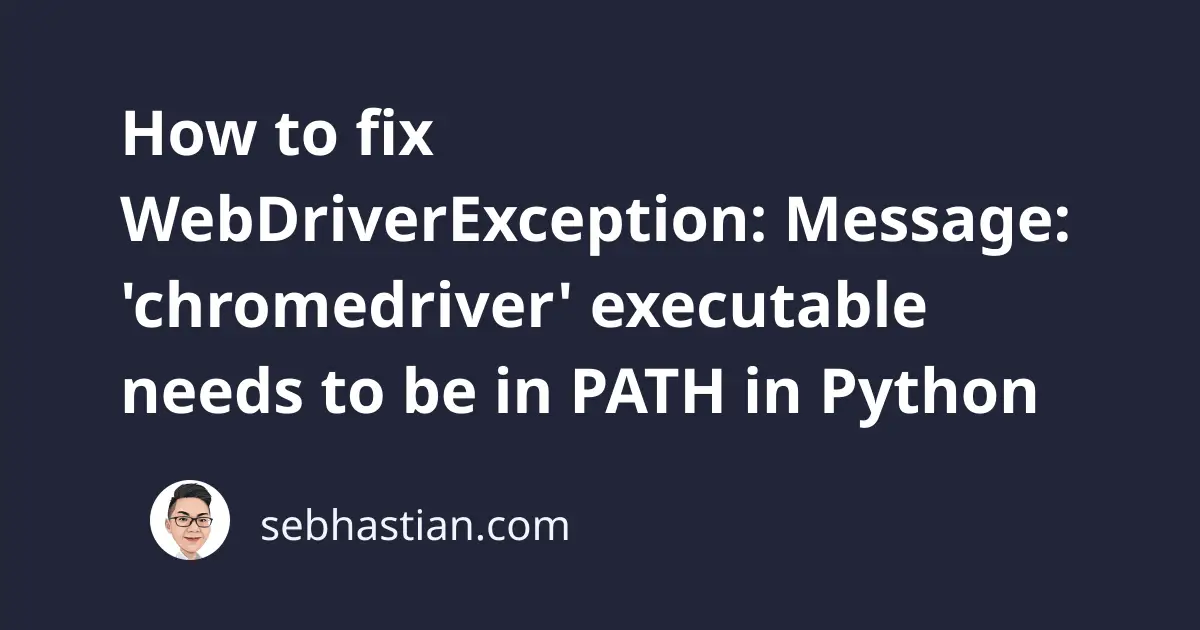
When running Selenium to automate the Chrome browser using Python, you might get the following error:
WebDriverException: Message: 'chromedriver' executable needs to be in PATH
This error occurs when you use Selenium to automate the Chrome browser, but the ChromeDriver is not found in the system’s PATH variable.
This tutorial shows an example that causes this error and how you can fix it.
How to reproduce this error,
Suppose you import the webdriver
module from selenium and create an instance of the Chrome
driver as follows:
from selenium import webdriver
browser = webdriver.Chrome()
Without having the ChromeDriver in the PATH, you’ll get an error as follows:
selenium.common.exceptions.WebDriverException:
Message: 'chromedriver' executable needs to be in PATH.
This error tells you that the ChromeDriver is not found in the PATH.
How to fix the error
There are three possible solutions to resolve this error:
- Upgrade selenium to version 4
- Use the webdriver_manager package to help you install web drivers
- Manually download Geckodriver and make it available under your PATH
Let’s see how you can fix the error using these solutions next.
1. Upgrade Selenium to version 4
The Selenium maintainers have released the Selenium Manager starting from Selenium 4.6.0.
The Selenium Manager is used to get the web drivers required by Selenium to automate browsers, so you no longer need to download the drivers separately.
To upgrade your Python Selenium bindings, use the pip
command below:
pip install selenium --upgrade
# for pip3:
pip3 install selenium --upgrade
Once you upgraded, automate the Chrome browser using the webdriver
module as follows:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://selenium.dev")
driver.quit()
Notice that this time you didn’t receive any errors. This is because the ChromeDriver is bundled in Selenium 4.
If for some reason you’re not ready to upgrade to Selenium 4, then you can use the webdriver_manager
package.
2. Install drivers using the webdriver_manager package
The webdriver_manager
package is used to install web drivers. This package can be used to install the ChromeDriver required by Selenium to automate Chrome.
First, install the webdriver_manager
package using pip
as follows:
pip install webdriver_manager
# or if you use pip3:
pip3 install webdriver_manager
Once installed, import the ChromeDriverManager
into your code. The code is a bit different depending on your Selenium version:
# selenium 4
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
driver = webdriver.Chrome(
service=ChromeService(ChromeDriverManager().install()))
# selenium 3
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
driver = webdriver.Chrome(ChromeDriverManager().install())
The ChromeDriverManager
will automatically check if the ChromeDriver executable is already available in the PATH or not. If not, it will download the latest version of ChromeDriver and add it to the PATH.
Once you get the ChromeDriver executable, you should be able to run your automated Chrome tests.
Using the webdriver_manager
is convenient because it removes the need to manually download and edit your system PATH.
If you don’t want to use the webdriver_manager
, you can install the ChromeDriver manually.
3. Download the ChromeDriver manually
You can download the ChromeDriver executable from its Download page and place it in a directory that is already in the system’s PATH. Please make sure you’re downloading the driver that’s compatible with your Chrome version.
For Windows, you can place the executable in the System32
folder. For macOS and Linux, you can place the driver in /usr/local/bin
or /usr/bin
.
Alternatively, you can include the directory where the executable was downloaded in your PATH variable.
Once the ChromeDriver is added to PATH, you should restart the terminal to make sure that the program is found.
Conclusion
The error WebDriverException: Message: 'chromedriver' executable needs to be in PATH
occurs in Python when you attempt to automate Chrome using Selenium, but you don’t have the ChromeDriver available under the PATH variable.
To resolve this error, you can upgrade Selenium to the latest stable version, which includes the required web driver automatically.
You can also use the webdriver_manager
package to help you install the ChromeDriver, or you can download the driver manually as well.
I hope this tutorial is helpful. See you in other articles! 😉