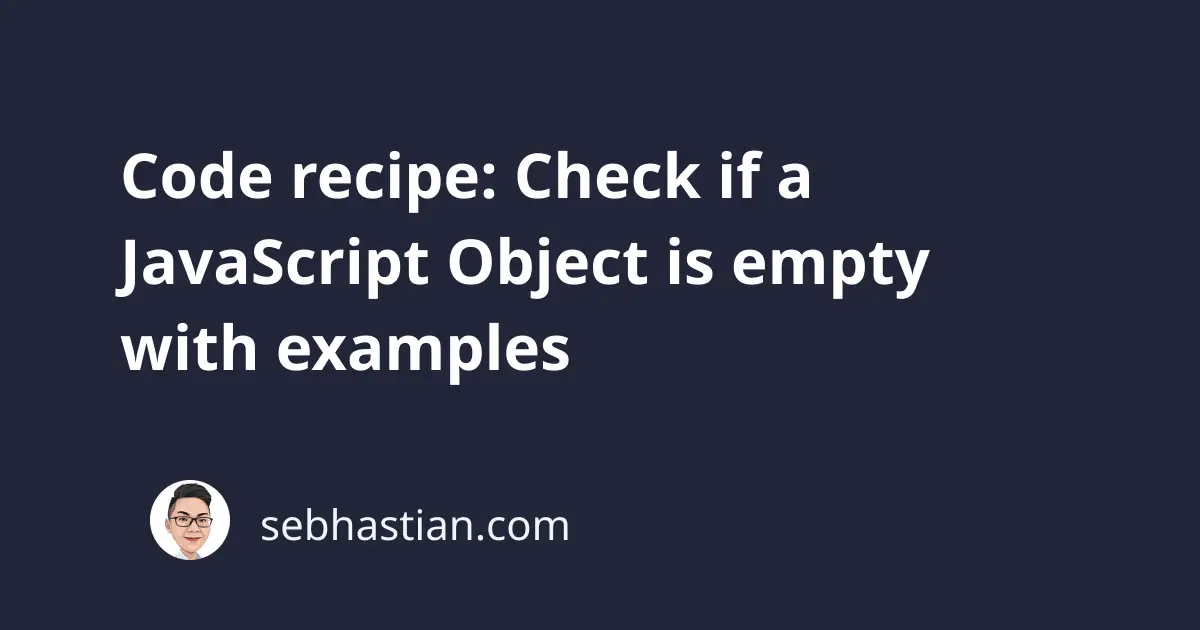
If you don’t want to know the explanations, here’s the code snippet to help you check whether a JavaScript Object
is empty or not:
let emptyObj = {};
if (Object.keys(emptyObj).length === 0 && emptyObj.constructor === Object) {
console.log("Object is empty");
}
Read on to learn how and why it works.
To check whether a JavaScript Object
is empty or not, you can check whether the Object
variable has enumerable key names or not by using the Object.keys()
method.
When you have no defined property names, the Object.keys()
method will return an empty Array
as follows:
let emptyObj = {};
console.log(Object.keys(emptyObj)); // Array []
let user = {
name: "Nathan",
age: 29,
};
console.log(Object.keys(user)); // Array ["name", "age"]
With this method, you can simply check the length
of the keys array and know that the Object
is empty when the length is 0
:
let emptyObj = {};
if (Object.keys(emptyObj).length === 0) {
console.log("The object is empty");
}
But be careful because JavaScript also has built-in constructors that allows you to create an Object
version of primitive data types. For example, you can create a String Object
like this:
let userObj = new String();
In total, JavaScript has 8 built-in constructors:
new Object(); // Object object
new String(); // String object
new Number(); // Number object
new Boolean(); // Boolean object
new Array(); // Array object
new RegExp(); // RegExp object
new Function(); // Function object
new Date(); // Date object
To check whether your Object
is really an Object
type, you can check whether the constructor
property equals to Object
.
Here’s the example:
let emptyObj = {};
console.log(emptyObj.constructor === Object); // true
let emptyStr = new String();
console.log(emptyStr.constructor === String); // true
console.log(emptyStr.constructor === Object); // false
let emptyNum = new Number();
console.log(emptyNum.constructor === Number); // true
console.log(emptyNum.constructor === Object); // false
Now you can combine both expressions to check whether an Object
variable is really an Object
and is empty:
let emptyObj = {};
if (Object.keys(emptyObj).length === 0 && emptyObj.constructor === Object) {
console.log("Object is empty");
} else {
console.log("Object is not empty");
}
Checking empty object before ES5
The Object.keys()
method is only available for JavaScript version ES5 (2009) or above, so if you need to support older browsers like Internet Explorer, you can use the JSON.stringify()
method and see if it returns an empty object.
Here’s an example:
let emptyObj = {};
if (JSON.stringify(emptyObj) === "{}") {
console.log("Object is empty");
}
It’s considered a hack, but it actually works 😉