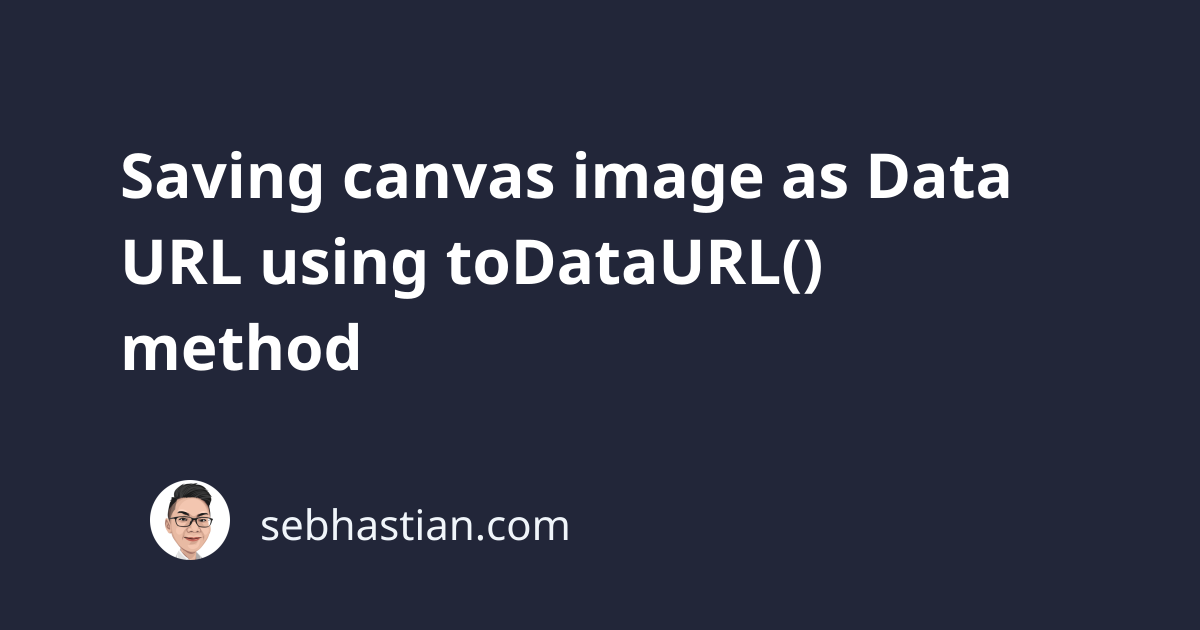
The toDataURL()
method is a method from the Canvas API that allows you to store an image drawn on top of the <canvas>
element in the form of a Data URL scheme.
The Data URL scheme allows you to embed an image in-line inside your HTML, saving you one extra HTTP request that would otherwise be performed for that image.
The method accepts two optional parameters:
- The type
string
for the image format. Defaults toimage/png
- The imageQuality
number
for image types that use lossy compression likeimage/jpeg
. The value must be between0
and1
with the default value being0.92
Let’s see the method in action. Suppose you’ve created a <canvas>
element as follows:
<body>
<canvas
id="emptyCanvas"
width="300"
height="300"
></canvas>
<script>
let canvas = document.getElementById("emptyCanvas");
let ctx = canvas.getContext("2d");
ctx.fillStyle = "blue";
ctx.fillRect(50, 100, 150, 150);
</script>
</body>
The code above will produce a rectangle drawing in your web browser as follows:
To transform the image drawn into a Data URL, you only need to call the toDataURI()
method on the canvas
object:
const dataURL = canvas.toDataURL();
console.log(dataURL);
The console.log()
result would be as follows. The ellipsis ...
in the second line omits the rest of the output and the third line shows the end of the URI:
data:image/png;base64,
iVBORw0KGgoAAAANSUhEUgAAASwAAAEsCAYAAAB5fY51AAALBElEQ...
ooAghcEBCsC6puIoDACQHBOsHqKAIIXBAQrAuqbiKAwAmBP7PLLTzDQluOAAAAAElFTkSuQmCC
The format of the scheme is as shown below:
data:[<mime type>][;charset=<charset>][;base64],<encoded data>
The encoded data is a very long string that the browser will render as an image. You need to pass the entire scheme as the src
attribute of an <img>
element.
To test the data scheme, you can copy and pass the Data URL into a new browser window to see the image rendered using the <img>
element. Notice how the data:image/png
scheme entered into the address bar below:
By transforming the image to a Data URL scheme, you can embed any image rendered inside the <canvas>
element in any web page without making an extra HTTP request.
But keep in mind that the Data URL scheme is not recommended for large images or images that are changed frequently because the scheme is not cached by the browser, meaning that the image will be downloaded each time the page is loaded.
On the other hand, an HTTP request for the image can be cached by the browser so that the image will be served from the cache when the page is loaded again.